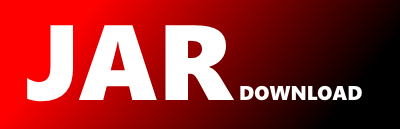
com.github.egatlovs.variablemanager.processing.FieldNames Maven / Gradle / Ivy
package com.github.egatlovs.variablemanager.processing;
import com.github.egatlovs.variablemanager.annotations.Ignore;
import com.github.egatlovs.variablemanager.annotations.ObjectValue;
import java.lang.reflect.Field;
import java.util.HashSet;
import java.util.Set;
/**
* FieldNames
*
* FieldNames is capable of building names out of a given Class. If the Class is
* annotated with {@code @ObjectValue} the values of the Annotation will be used
* to determine if the name of the Object or of the Fields of the Object are
* returned.
*
* Note:
* Fields annotated with {@code @Ignore} are ignored and no name will be
* provided.
*
* @author egatlovs
*/
public class FieldNames {
/**
* This method determines names out of a given Class. If the Class is annotated
* with {@code @ObjectValue} the values of the Annotation will be used to
* determine if the name of the Object or of the Fields of the Object are
* returned.
*
* Note:
* Fields annotated with {@code @Ignore} are ignored and no name will be
* provided.
*
* @param clazz - The Class from which the names will be processed
* @return The processed names
*/
public Set getNames(Class clazz) {
Set names = new HashSet<>();
if (clazz.isAnnotationPresent(ObjectValue.class)) {
ObjectValue objectValue = clazz.getAnnotation(ObjectValue.class);
if (!objectValue.storeFields()) {
names.add(getObjectName(clazz));
} else {
getFieldNames(clazz, names);
}
} else {
names.add(getObjectName(clazz));
}
return names;
}
private void getFieldNames(Class clazz, Set names) {
FieldNameExtractor nameExtractor = new FieldNameExtractor();
Field[] declaredFields = clazz.getDeclaredFields();
for (Field declaredField : declaredFields) {
if (!declaredField.isSynthetic() && !declaredField.isAnnotationPresent(Ignore.class)) {
if (declaredField.isAnnotationPresent(ObjectValue.class)) {
ObjectValue objectValue = declaredField.getAnnotation(ObjectValue.class);
if (objectValue.storeFields()) {
getFieldNames(declaredField.getType(), names);
} else {
names.add(nameExtractor.getFrom(declaredField));
}
} else {
names.add(nameExtractor.getFrom(declaredField));
}
}
}
}
private String getObjectName(Class clazz) {
FieldNameExtractor nameExtractor = new FieldNameExtractor();
return nameExtractor.getFrom(clazz);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy