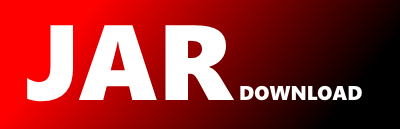
com.github.ejahns.token.KeywordProvider Maven / Gradle / Ivy
The newest version!
package com.github.ejahns.token;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import com.github.ejahns.Parser.TokenType;
import static com.github.ejahns.Constants.*;
import static com.github.ejahns.Parser.TokenType.*;
public class KeywordProvider {
private final String language;
private final Map> keywords;
private final Map, TokenType> titleAssociations;
private final Map, TokenType> stepAssociations;
KeywordProvider(String language, Map> keywords) {
this.language = language;
this.keywords = keywords;
titleAssociations = new LinkedHashMap<>();
stepAssociations = new LinkedHashMap<>();
titleAssociations.put(getFeatureKeywords(), FeatureLineToken);
titleAssociations.put(getBackgroundKeywords(), BackgroundLineToken);
titleAssociations.put(getScenarioKeywords(), ScenarioLineToken);
titleAssociations.put(getScenarioOutlineKeywords(), ScenarioOutlineLineToken);
titleAssociations.put(getExamplesKeywords(), ExamplesLineToken);
stepAssociations.put(getStepKeywords(), StepLineToken);
}
TokenAssociation matchTokenType(String line) {
for (List list : titleAssociations.keySet()) {
for (String keyword : list) {
if (line.startsWith(keyword + TITLE_SEPARATOR)) {
line = line.substring(keyword.length() + TITLE_SEPARATOR.length()).trim();
line = line.equals("") ? null : line;
return new TokenAssociation(
keyword,
titleAssociations.get(list),
line
);
}
}
}
for (List list : stepAssociations.keySet()) {
for (String keyword : list) {
if (line.startsWith(keyword)) {
return new TokenAssociation(
keyword.trim(),
stepAssociations.get(list),
line.substring(keyword.length())
.trim()
);
}
}
}
return null;
}
private List getFeatureKeywords() {
return keywords.get("feature");
}
private List getScenarioKeywords() {
return keywords.get("scenario");
}
private List getBackgroundKeywords() {
return keywords.get("background");
}
private List getScenarioOutlineKeywords() {
return keywords.get("scenarioOutline");
}
private List getExamplesKeywords() {
return keywords.get("examples");
}
private List getStepKeywords() {
HashSet keywords = new HashSet<>();
keywords.addAll(getGivenKeywords());
keywords.addAll(getWhenKeywords());
keywords.addAll(getThenKeywords());
keywords.addAll(getAndKeywords());
keywords.addAll(getButKeywords());
return new ArrayList<>(keywords);
}
private List getGivenKeywords() {
return keywords.get("given");
}
private List getWhenKeywords() {
return keywords.get("when");
}
private List getThenKeywords() {
return keywords.get("then");
}
private List getAndKeywords() {
return keywords.get("and");
}
private List getButKeywords() {
return keywords.get("but");
}
public String getLanguage() {
return language;
}
class TokenAssociation {
final String keyword;
final TokenType tokenType;
final String line;
TokenAssociation(String keyword, TokenType tokenType, String line) {
this.keyword = keyword;
this.tokenType = tokenType;
this.line = line;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy