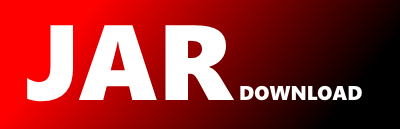
com.github.eladb.watchful.WatchApiGateway Maven / Gradle / Ivy
Show all versions of cdk-watchful Show documentation
package com.github.eladb.watchful;
/**
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.13.0 (build 385c325)", date = "2020-10-05T09:37:29.727Z")
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Jsii(module = com.github.eladb.watchful.$Module.class, fqn = "cdk-watchful.WatchApiGateway")
public class WatchApiGateway extends software.amazon.awscdk.core.Construct {
protected WatchApiGateway(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
}
protected WatchApiGateway(final software.amazon.jsii.JsiiObject.InitializationMode initializationMode) {
super(initializationMode);
}
/**
* @param scope This parameter is required.
* @param id This parameter is required.
* @param props This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public WatchApiGateway(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.core.Construct scope, final @org.jetbrains.annotations.NotNull java.lang.String id, final @org.jetbrains.annotations.NotNull com.github.eladb.watchful.WatchApiGatewayProps props) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required"), java.util.Objects.requireNonNull(props, "props is required") });
}
/**
* A fluent builder for {@link com.github.eladb.watchful.WatchApiGateway}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
/**
* @return a new instance of {@link Builder}.
* @param scope This parameter is required.
* @param id This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static Builder create(final software.amazon.awscdk.core.Construct scope, final java.lang.String id) {
return new Builder(scope, id);
}
private final software.amazon.awscdk.core.Construct scope;
private final java.lang.String id;
private final com.github.eladb.watchful.WatchApiGatewayProps.Builder props;
private Builder(final software.amazon.awscdk.core.Construct scope, final java.lang.String id) {
this.scope = scope;
this.id = id;
this.props = new com.github.eladb.watchful.WatchApiGatewayProps.Builder();
}
/**
* Include a dashboard graph for caching metrics.
*
* Default: false
*
* @return {@code this}
* @param cacheGraph Include a dashboard graph for caching metrics. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder cacheGraph(final java.lang.Boolean cacheGraph) {
this.props.cacheGraph(cacheGraph);
return this;
}
/**
* Alarm when 5XX errors reach this threshold over 5 minutes.
*
* Default: 1 any 5xx HTTP response will trigger the alarm
*
* @return {@code this}
* @param serverErrorThreshold Alarm when 5XX errors reach this threshold over 5 minutes. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder serverErrorThreshold(final java.lang.Number serverErrorThreshold) {
this.props.serverErrorThreshold(serverErrorThreshold);
return this;
}
/**
* A list of operations to monitor separately.
*
* Default: - only API-level monitoring is added.
*
* @return {@code this}
* @param watchedOperations A list of operations to monitor separately. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder watchedOperations(final java.util.List extends com.github.eladb.watchful.WatchedOperation> watchedOperations) {
this.props.watchedOperations(watchedOperations);
return this;
}
/**
* The API Gateway REST API that is being watched.
*
* @return {@code this}
* @param restApi The API Gateway REST API that is being watched. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder restApi(final software.amazon.awscdk.services.apigateway.RestApi restApi) {
this.props.restApi(restApi);
return this;
}
/**
* The title of this section.
*
* @return {@code this}
* @param title The title of this section. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder title(final java.lang.String title) {
this.props.title(title);
return this;
}
/**
* The Watchful instance to add widgets into.
*
* @return {@code this}
* @param watchful The Watchful instance to add widgets into. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder watchful(final com.github.eladb.watchful.IWatchful watchful) {
this.props.watchful(watchful);
return this;
}
/**
* @returns a newly built instance of {@link com.github.eladb.watchful.WatchApiGateway}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public com.github.eladb.watchful.WatchApiGateway build() {
return new com.github.eladb.watchful.WatchApiGateway(
this.scope,
this.id,
this.props.build()
);
}
}
}