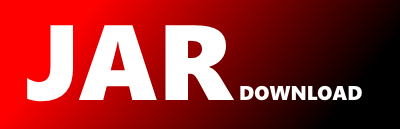
com.github.eladb.watchful.WatchDynamoTableProps Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cdk-watchful Show documentation
Show all versions of cdk-watchful Show documentation
Watching your CDK apps since 2019
package com.github.eladb.watchful;
/**
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.13.0 (build 385c325)", date = "2020-11-09T06:11:47.374Z")
@software.amazon.jsii.Jsii(module = com.github.eladb.watchful.$Module.class, fqn = "cdk-watchful.WatchDynamoTableProps")
@software.amazon.jsii.Jsii.Proxy(WatchDynamoTableProps.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public interface WatchDynamoTableProps extends software.amazon.jsii.JsiiSerializable, com.github.eladb.watchful.WatchDynamoTableOptions {
/**
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull software.amazon.awscdk.services.dynamodb.Table getTable();
/**
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getTitle();
/**
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull com.github.eladb.watchful.IWatchful getWatchful();
/**
* @return a {@link Builder} of {@link WatchDynamoTableProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link WatchDynamoTableProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
private software.amazon.awscdk.services.dynamodb.Table table;
private java.lang.String title;
private com.github.eladb.watchful.IWatchful watchful;
private java.lang.Number readCapacityThresholdPercent;
private java.lang.Number writeCapacityThresholdPercent;
/**
* Sets the value of {@link WatchDynamoTableProps#getTable}
* @param table the value to be set. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder table(software.amazon.awscdk.services.dynamodb.Table table) {
this.table = table;
return this;
}
/**
* Sets the value of {@link WatchDynamoTableProps#getTitle}
* @param title the value to be set. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder title(java.lang.String title) {
this.title = title;
return this;
}
/**
* Sets the value of {@link WatchDynamoTableProps#getWatchful}
* @param watchful the value to be set. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder watchful(com.github.eladb.watchful.IWatchful watchful) {
this.watchful = watchful;
return this;
}
/**
* Sets the value of {@link WatchDynamoTableProps#getReadCapacityThresholdPercent}
* @param readCapacityThresholdPercent Threshold for read capacity alarm (percentage).
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder readCapacityThresholdPercent(java.lang.Number readCapacityThresholdPercent) {
this.readCapacityThresholdPercent = readCapacityThresholdPercent;
return this;
}
/**
* Sets the value of {@link WatchDynamoTableProps#getWriteCapacityThresholdPercent}
* @param writeCapacityThresholdPercent Threshold for read capacity alarm (percentage).
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder writeCapacityThresholdPercent(java.lang.Number writeCapacityThresholdPercent) {
this.writeCapacityThresholdPercent = writeCapacityThresholdPercent;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link WatchDynamoTableProps}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public WatchDynamoTableProps build() {
return new Jsii$Proxy(table, title, watchful, readCapacityThresholdPercent, writeCapacityThresholdPercent);
}
}
/**
* An implementation for {@link WatchDynamoTableProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements WatchDynamoTableProps {
private final software.amazon.awscdk.services.dynamodb.Table table;
private final java.lang.String title;
private final com.github.eladb.watchful.IWatchful watchful;
private final java.lang.Number readCapacityThresholdPercent;
private final java.lang.Number writeCapacityThresholdPercent;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.table = this.jsiiGet("table", software.amazon.awscdk.services.dynamodb.Table.class);
this.title = this.jsiiGet("title", java.lang.String.class);
this.watchful = this.jsiiGet("watchful", com.github.eladb.watchful.IWatchful.class);
this.readCapacityThresholdPercent = this.jsiiGet("readCapacityThresholdPercent", java.lang.Number.class);
this.writeCapacityThresholdPercent = this.jsiiGet("writeCapacityThresholdPercent", java.lang.Number.class);
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
private Jsii$Proxy(final software.amazon.awscdk.services.dynamodb.Table table, final java.lang.String title, final com.github.eladb.watchful.IWatchful watchful, final java.lang.Number readCapacityThresholdPercent, final java.lang.Number writeCapacityThresholdPercent) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.table = java.util.Objects.requireNonNull(table, "table is required");
this.title = java.util.Objects.requireNonNull(title, "title is required");
this.watchful = java.util.Objects.requireNonNull(watchful, "watchful is required");
this.readCapacityThresholdPercent = readCapacityThresholdPercent;
this.writeCapacityThresholdPercent = writeCapacityThresholdPercent;
}
@Override
public software.amazon.awscdk.services.dynamodb.Table getTable() {
return this.table;
}
@Override
public java.lang.String getTitle() {
return this.title;
}
@Override
public com.github.eladb.watchful.IWatchful getWatchful() {
return this.watchful;
}
@Override
public java.lang.Number getReadCapacityThresholdPercent() {
return this.readCapacityThresholdPercent;
}
@Override
public java.lang.Number getWriteCapacityThresholdPercent() {
return this.writeCapacityThresholdPercent;
}
@Override
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("table", om.valueToTree(this.getTable()));
data.set("title", om.valueToTree(this.getTitle()));
data.set("watchful", om.valueToTree(this.getWatchful()));
if (this.getReadCapacityThresholdPercent() != null) {
data.set("readCapacityThresholdPercent", om.valueToTree(this.getReadCapacityThresholdPercent()));
}
if (this.getWriteCapacityThresholdPercent() != null) {
data.set("writeCapacityThresholdPercent", om.valueToTree(this.getWriteCapacityThresholdPercent()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("cdk-watchful.WatchDynamoTableProps"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
WatchDynamoTableProps.Jsii$Proxy that = (WatchDynamoTableProps.Jsii$Proxy) o;
if (!table.equals(that.table)) return false;
if (!title.equals(that.title)) return false;
if (!watchful.equals(that.watchful)) return false;
if (this.readCapacityThresholdPercent != null ? !this.readCapacityThresholdPercent.equals(that.readCapacityThresholdPercent) : that.readCapacityThresholdPercent != null) return false;
return this.writeCapacityThresholdPercent != null ? this.writeCapacityThresholdPercent.equals(that.writeCapacityThresholdPercent) : that.writeCapacityThresholdPercent == null;
}
@Override
public int hashCode() {
int result = this.table.hashCode();
result = 31 * result + (this.title.hashCode());
result = 31 * result + (this.watchful.hashCode());
result = 31 * result + (this.readCapacityThresholdPercent != null ? this.readCapacityThresholdPercent.hashCode() : 0);
result = 31 * result + (this.writeCapacityThresholdPercent != null ? this.writeCapacityThresholdPercent.hashCode() : 0);
return result;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy