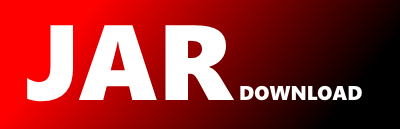
org.projen.github.Stale Maven / Gradle / Ivy
Show all versions of projen Show documentation
package org.projen.github;
/**
* (experimental) Warns and then closes issues and PRs that have had no activity for a specified amount of time.
*
* The default configuration will:
*
*
* - Add a "Stale" label to pull requests after 14 days and closed after 2 days
* - Add a "Stale" label to issues after 60 days and closed after 7 days
* - If a comment is added, the label will be removed and timer is restarted.
*
*
* @see https://github.com/actions/stale
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.34.0 (build 9b72778)", date = "2021-09-05T18:03:09.276Z")
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@software.amazon.jsii.Jsii(module = org.projen.$Module.class, fqn = "projen.github.Stale")
public class Stale extends org.projen.Component {
protected Stale(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
}
protected Stale(final software.amazon.jsii.JsiiObject.InitializationMode initializationMode) {
super(initializationMode);
}
/**
* @param github This parameter is required.
* @param options
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Stale(final @org.jetbrains.annotations.NotNull org.projen.github.GitHub github, final @org.jetbrains.annotations.Nullable org.projen.github.StaleOptions options) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { java.util.Objects.requireNonNull(github, "github is required"), options });
}
/**
* @param github This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Stale(final @org.jetbrains.annotations.NotNull org.projen.github.GitHub github) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { java.util.Objects.requireNonNull(github, "github is required") });
}
/**
* (experimental) A fluent builder for {@link org.projen.github.Stale}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public static final class Builder implements software.amazon.jsii.Builder {
/**
* @return a new instance of {@link Builder}.
* @param github This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public static Builder create(final org.projen.github.GitHub github) {
return new Builder(github);
}
private final org.projen.github.GitHub github;
private org.projen.github.StaleOptions.Builder options;
private Builder(final org.projen.github.GitHub github) {
this.github = github;
}
/**
* (experimental) How to handle stale issues.
*
* Default: - By default, stale issues with no activity will be marked as
* stale after 60 days and closed within 7 days.
*
* @return {@code this}
* @param issues How to handle stale issues. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder issues(final org.projen.github.StaleBehavior issues) {
this.options().issues(issues);
return this;
}
/**
* (experimental) How to handle stale pull requests.
*
* Default: - By default, pull requests with no activity will be marked as
* stale after 14 days and closed within 2 days with relevant comments.
*
* @return {@code this}
* @param pullRequest How to handle stale pull requests. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder pullRequest(final org.projen.github.StaleBehavior pullRequest) {
this.options().pullRequest(pullRequest);
return this;
}
/**
* @returns a newly built instance of {@link org.projen.github.Stale}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@Override
public org.projen.github.Stale build() {
return new org.projen.github.Stale(
this.github,
this.options != null ? this.options.build() : null
);
}
private org.projen.github.StaleOptions.Builder options() {
if (this.options == null) {
this.options = new org.projen.github.StaleOptions.Builder();
}
return this.options;
}
}
}