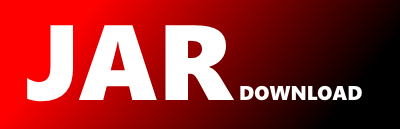
com.github.elibracha.model.ChangedParameters Maven / Gradle / Ivy
package com.github.elibracha.model;
import io.swagger.v3.oas.models.parameters.Parameter;
import java.util.ArrayList;
import java.util.List;
import lombok.Getter;
import lombok.Setter;
import lombok.experimental.Accessors;
@Getter
@Setter
@Accessors(chain = true)
public class ChangedParameters implements ComposedChanged {
private final List oldParameterList;
private final List newParameterList;
private final DiffContext context;
private List increased;
private List missing;
private List changed;
public ChangedParameters(
List oldParameterList, List newParameterList, DiffContext context) {
this.oldParameterList = oldParameterList;
this.newParameterList = newParameterList;
this.context = context;
this.increased = new ArrayList<>();
this.missing = new ArrayList<>();
this.changed = new ArrayList<>();
}
@Override
public List getChangedElements() {
return new ArrayList<>(changed);
}
@Override
public DiffResult isCoreChanged() {
if (increased.isEmpty() && missing.isEmpty()) {
return DiffResult.NO_CHANGES;
}
if (increased.stream().noneMatch(Parameter::getRequired) && missing.isEmpty()) {
return DiffResult.COMPATIBLE;
}
return DiffResult.INCOMPATIBLE;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy