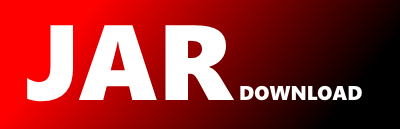
org.xmind.core.Core Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of xmindjbehaveplugin Show documentation
Show all versions of xmindjbehaveplugin Show documentation
Plugin that manages JBehave stories storage in XMind mindmap file, which allows design jBehave tests right after the brainstorm
The newest version!
/* ******************************************************************************
* Copyright (c) 2006-2012 XMind Ltd. and others.
*
* This file is a part of XMind 3. XMind releases 3 and
* above are dual-licensed under the Eclipse Public License (EPL),
* which is available at http://www.eclipse.org/legal/epl-v10.html
* and the GNU Lesser General Public License (LGPL),
* which is available at http://www.gnu.org/licenses/lgpl.html
* See http://www.xmind.net/license.html for details.
*
* Contributors:
* XMind Ltd. - initial API and implementation
*******************************************************************************/
package org.xmind.core;
import java.util.Comparator;
import org.xmind.core.internal.InternalCore;
import org.xmind.core.marker.IMarkerSheetBuilder;
import org.xmind.core.style.IStyleSheetBuilder;
import org.xmind.core.util.ILogger;
public class Core {
/**
* Core event type for modifying the title text (value is 'titleText').
*
* - Source:
* - {@link org.xmind.core.ITitled}
* - OldValue:
* - the old title ({@link String}), or
null
indicating that
* the title was unspecified
* - NewValue:
* - the new title ({@link String}), or
null
indicating that
* the title is unspecified
*
*
* @see org.xmind.core.ITitled#getTitleText()
* @see org.xmind.core.ITitled#setTitleText(String)
*/
public static final String TitleText = "titleText"; //$NON-NLS-1$
/**
* Core event type for modifying a topic's title width (value is
* 'titleWidth').
*
* - Source:
* - {@link org.xmind.core.ITopic}
* - OldValue:
* - the old width ({@link Integer}), or
null
indicating that
* the width was unspecified
* - NewValue:
* - the new width ({@link Integer}), or
null
indicating that
* the with is unspecified
*
*
* @see org.xmind.core.ITopic#getTitleWidth()
* @see org.xmind.core.ITopic#setTitleWidth(int)
*/
public static final String TitleWidth = "titleWidth"; //$NON-NLS-1$
/**
* Core event type for modifying the style id (value is 'style').
*
* - Source:
* - {@link org.xmind.core.style.IStyled}
* - OldValue:
* - the old style's id ({@link String}), or
null
indicating
* that the style was unspecified
* - NewValue:
* - the new style's id ({@link String}), or
null
indicating
* that the style is unspecified
*
*
* @see org.xmind.core.style.IStyled#getStyleId()
* @see org.xmind.core.style.IStyled#setStyleId(String)
*/
public static final String Style = "style"; //$NON-NLS-1$
/**
* Core event type for folding/unfolding a topic (value is 'topicFolded').
*
* - Source:
* - {@link org.xmind.core.ITopic}
* - OldValue:
* - the old folding state ({@link Boolean})
* - NewValue:
* - the new folding state ({@link Boolean})
*
*
* @see org.xmind.core.ITopic#isFolded()
* @see org.xmind.core.ITopic#setFolded(boolean)
*/
public static final String TopicFolded = "topicFolded"; //$NON-NLS-1$
/**
* Core event type for modifying an object's position (value is 'position').
*
* - Source:
* - {@link org.xmind.core.IPositioned}
* - OldValue:
* - the old position ({@link org.xmind.core.util.Point}), or
*
null
indicating that the position was unspecified
* - NewValue:
* - the new position ({@link org.xmind.core.util.Point}), or
*
null
indicating that the position is unspecified
*
*
* @see org.xmind.core.IPositioned#getPosition()
* @see org.xmind.core.IPositioned#setPosition(org.xmind.core.util.Point)
* @see org.xmind.core.IPositioned#setPosition(int, int)
*/
public static final String Position = "position"; //$NON-NLS-1$
/**
* Core event type for modifying a topic's hyperlink reference (value is
* 'topicHyperlink').
*
* - Source:
* - {@link org.xmind.core.ITopic}
* - OldValue:
* - the old hyperlink reference ({@link String}), or
null
* indicating that the hyperlink was unspecified
* - NewValue:
* - the new hyperlink reference ({@link String}), or
null
* indicating that the hyperlink is unspecified
*
*
* @see org.xmind.core.ITopic#getHyperlink()
* @see org.xmind.core.ITopic#setHyperlink(String)
*/
public static final String TopicHyperlink = "topicHyperlink"; //$NON-NLS-1$
/**
* Core event type for adding a subtopic to a topic (value is 'topicAdd').
*
* - Source:
* - the parent {@link org.xmind.core.ITopic}
* - Target:
* - the child {@link org.xmind.core.ITopic}
* - Index:
* - the index of the added subtopic
* - Data:
* - the child topic's type ({@link String})
*
*
* @see org.xmind.core.ITopic#getType()
* @see org.xmind.core.ITopic#add(org.xmind.core.ITopic)
* @see org.xmind.core.ITopic#add(org.xmind.core.ITopic, String)
* @see org.xmind.core.ITopic#add(org.xmind.core.ITopic, int, String)
*/
public static final String TopicAdd = "topicAdd"; //$NON-NLS-1$
/**
* Core event type for removing a subtopic from a topic (value is
* 'topicRemove').
*
* - Source:
* - the parent {@link org.xmind.core.ITopic}
* - Target:
* - the child {@link org.xmind.core.ITopic}
* - Index:
* - the index of the removed subtopic
* - Data:
* - the child topic's type ({@link String})
*
*
* @see org.xmind.core.ITopic#remove(org.xmind.core.ITopic)
*/
public static final String TopicRemove = "topicRemove"; //$NON-NLS-1$
/**
* Core event type for adding a sheet to a workbook (value is 'sheetAdd').
*
* - Source:
* - {@link org.xmind.core.IWorkbook}
* - Target:
* - the added {@link org.xmind.core.ISheet}
* - Index:
* - the index of the added sheet
*
*
* @see org.xmind.core.IWorkbook#addSheet(org.xmind.core.ISheet)
* @see org.xmind.core.IWorkbook#addSheet(org.xmind.core.ISheet, int)
*/
public static final String SheetAdd = "sheetAdd"; //$NON-NLS-1$
/**
* Core event type for removing a sheet from a workbook (value is
* 'sheetRemove').
*
* - Source:
* - {@link org.xmind.core.IWorkbook}
* - Target:
* - the removed {@link org.xmind.core.ISheet}
* - Index:
* - the index of the removed sheet
*
*
* @see org.xmind.core.IWorkbook#removeSheet(org.xmind.core.ISheet)
*/
public static final String SheetRemove = "sheetRemove"; //$NON-NLS-1$
/**
* Core event type for moving a sheet to a different index within the owned
* workbook (value is 'sheetMove').
*
* - Source:
* - {@link org.xmind.core.IWorkbook}
* - Target:
* - the moved {@link org.xmind.core.ISheet}
* - Index:
* - the old index of the moved sheet
*
*
* @see org.xmind.core.IWorkbook#moveSheet(int, int)
*/
public static final String SheetMove = "sheetMove"; //$NON-NLS-1$
/**
* Core event type for adding a relationship to a sheet (value is
* 'relationshipAdd').
*
* - Source:
* - {@link org.xmind.core.ISheet}
* - Target:
* - the added {@link org.xmind.core.IRelationship}
*
*
* @see org.xmind.core.ISheet#addRelationship(org.xmind.core.IRelationship)
*/
public static final String RelationshipAdd = "relationshipAdd"; //$NON-NLS-1$
/**
* Core event type for removing a relationship from a sheet (value is
* 'relationshipRemove').
*
* - Source:
* - {@link org.xmind.core.ISheet}
* - Target:
* - the removed {@link org.xmind.core.IRelationship}
*
*
* @see org.xmind.core.ISheet#removeRelationship(org.xmind.core.IRelationship)
*/
public static final String RelationshipRemove = "relationshipRemove"; //$NON-NLS-1$
/**
* Core event type for replacing the root topic of a sheet (value is
* 'rootTopic').
*
* - Source:
* - {@link org.xmind.core.ISheet}
* - OldValue:
* - the old root {@link org.xmind.core.ITopic}
* - NewValue:
* - the new root {@link org.xmind.core.ITopic}
*
*
* @see org.xmind.core.ISheet#getRootTopic()
* @see org.xmind.core.ISheet#replaceRootTopic(ITopic)
*/
public static final String RootTopic = "rootTopic"; //$NON-NLS-1$
// /**
// * Core event type for modifying one control point on a relationship (value
// * is 'relationshipControlPoint').
// *
// * - Source:
// * - {@link org.xmind.core.IRelationship}
// * - Target:
// * - the modified {@link org.xmind.core.IControlPoint}, or
// *
null
indicating that the control point is unspecified
// * - Index:
// * - the index of the modified control point
// *
// *
// * @see org.xmind.core.IRelationship#getControlPoint(int);
// * @see org.xmind.core.IRelationship#setControlPoint(int, double, double);
// * @see org.xmind.core.IRelationship#resetControlPoint(int);
// */
// public static final String RelationshipControlPoint = "relationshipControlPoint"; //$NON-NLS-1$
/**
* Core event type for modifying the first end of a relationship (value is
* 'relationshipEnd1').
*
* - Source:
* - {@link org.xmind.core.IRelationship}
* - OldValue:
* - the old first end's id ({@link String}), or
null
* indicating that the first end was unspecified
* - NewValue:
* - the new first end's id ({@link String}), or
null
* indicating that the first end is unspecified
*
*
* @see org.xmind.core.IRelationship#getEnd1()
* @see org.xmind.core.IRelationship#getEnd1Id()
* @see org.xmind.core.IRelationship#setEnd1Id(String)
*/
public static final String RelationshipEnd1 = "relationshipEnd1"; //$NON-NLS-1$
/**
* Core event type for modifying the second end of a relationship (value is
* 'relationshipEnd2').
*
* - Source:
* - {@link org.xmind.core.IRelationship}
* - OldValue:
* - the old second end's id ({@link String}), or
null
* indicating that the second end was unspecified
* - NewValue:
* - the new second end's id ({@link String}), or
null
* indicating that the second end is unspecified
*
*
* @see org.xmind.core.IRelationship#getEnd2()
* @see org.xmind.core.IRelationship#getEnd2Id()
* @see org.xmind.core.IRelationship#setEnd2Id(String)
*/
public static final String RelationshipEnd2 = "relationshipEnd2"; //$NON-NLS-1$
/**
* Core event type for adding a marker to a marker group (value is
* 'markerAdd').
*
* - Source:
* - {@link org.xmind.core.marker.IMarkerGroup}
* - Target:
* - the added {@link org.xmind.core.marker.IMarker}
* - Index:
* - the index of the added marker
*
*
* @see org.xmind.core.marker.IMarkerGroup#addMarker(org.xmind.core.marker.IMarker)
*/
public static final String MarkerAdd = "markerAdd"; //$NON-NLS-1$
/**
* Core event type for removing a marker from a marker group (value is
* 'markerRemove').
*
* - Source:
* - {@link org.xmind.core.marker.IMarkerGroup}
* - Target:
* - the removed {@link org.xmind.core.marker.IMarker}
* - Index:
* - the index of the removed marker
*
*
* @see org.xmind.core.marker.IMarkerGroup#removeMarker(org.xmind.core.marker.IMarker)
*/
public static final String MarkerRemove = "markerRemove"; //$NON-NLS-1$
/**
* Core event type for adding a marker group to a marker sheet (value is
* 'markerGroupAdd').
*
* - Source:
* - {@link org.xmind.core.marker.IMarkerSheet}
* - Target:
* - the added {@link org.xmind.core.marker.IMarkerGroup}
* - Index:
* - the index of the added marker group
*
*
* @see org.xmind.core.marker.IMarkerSheet#addMarkerGroup(org.xmind.core.marker.IMarkerGroup)
*/
public static final String MarkerGroupAdd = "markerGroupAdd"; //$NON-NLS-1$
/**
* Core event type for removing a marker group from a marker sheet (value is
* 'markerGroupRemove').
*
* - Source:
* - {@link org.xmind.core.marker.IMarkerSheet}
* - Target:
* - the removed {@link org.xmind.core.marker.IMarkerGroup}
* - Index:
* - the index of the removed marker group
*
*
* @see org.xmind.core.marker.IMarkerSheet#removeMarkerGroup(org.xmind.core.marker.IMarkerGroup)
*/
public static final String MarkerGroupRemove = "markerGroupRemove"; //$NON-NLS-1$
/**
* Core event type for adding a marker to a topic (value is 'markerRefAdd').
*
* - Source:
* - {@link org.xmind.core.ITopic}
* - Target:
* - the added marker's id ({@link String})
*
*
* @see org.xmind.core.ITopic#addMarker(String)
*/
public static final String MarkerRefAdd = "markerRefAdd"; //$NON-NLS-1$
/**
* Core event type for removong a marker from a topic (value is
* 'markerRefRemove').
*
* - Source:
* - {@link org.xmind.core.ITopic}
* - Target:
* - the removed marker's id ({@link String})
*
*
* @see org.xmind.core.ITopic#removeMarker(String)
*/
public static final String MarkerRefRemove = "markerRefRemove"; //$NON-NLS-1$
/**
* Core event type for modifying the range of a Range object (value is
* 'range').
*
* An event of this type usually follows a 'startIndex' or 'endIndex' event.
*
*
* - Source:
* - {@link org.xmind.core.IRange}
* - OldValue:
* - unspecified
* - NewValue:
* - unspecified
*
*/
public static final String Range = "range"; //$NON-NLS-1$;
/**
* Core event type for modifying the starting index of a Range object (value
* is 'startIndex').
*
* NOTE: This event is always followed by a 'range' event as the
* range is modified.
*
*
*
* - Source:
* - {@link org.xmind.core.IRange}
* - OldValue:
* - the old starting index ({@link Integer}) of the range, or
*
null
indicating that the index was unspecified
* - NewValue:
* - the new starting index ({@link Integer}) of the range, or
*
null
indicating that the index is unspecified
*
*/
public static final String StartIndex = "startIndex"; //$NON-NLS-1$
/**
* Core event type for modifying the ending index of a Range object (value
* is 'endIndex').
*
* NOTE: This event is always followed by a 'range' event as the
* range is modified.
*
*
*
* - Source:
* - {@link org.xmind.core.IRange}
* - OldValue:
* - the old ending index ({@link Integer}) of the range, or
*
null
indicating that the index was unspecified
* - NewValue:
* - the new ending index ({@link Integer}) of the range, or
*
null
indicating that the index is unspecified
*
*/
public static final String EndIndex = "endIndex"; //$NON-NLS-1$
/**
* Core event type for adding a boundary to a topic (value is
* 'boundaryAdd').
*
*
* - Source:
* - {@link org.xmind.core.ITopic}
* - Target:
* - the added {@link org.xmind.core.IBoundary}
*
*/
public static final String BoundaryAdd = "boundaryAdd"; //$NON-NLS-1$
/**
* Core event type for removing a boundary to a topic (value is
* 'boundaryRemove').
*
*
* - Source:
* - {@link org.xmind.core.ITopic}
* - Target:
* - the removed {@link org.xmind.core.IBoundary}
*
*/
public static final String BoundaryRemove = "boundaryRemove"; //$NON-NLS-1$
/**
* Core event type for modifying the attached topic of a summary (value is
* 'topicRefId').
*
* - Source:
* - {@link org.xmind.core.ISummary}
* - OldValue:
* - the old topic's id ({@link String})
* - NewValue:
* - the new topic's id ({@link String})
*
*/
public static final String TopicRefId = "topicRefId"; //$NON-NLS-1$
/**
* Core event type for adding a summary to a topic (value is 'summaryAdd').
*
*
* - Source:
* - {@link org.xmind.core.ITopic}
* - Target:
* - the added {@link org.xmind.core.IBoundary}
*
*/
public static final String SummaryAdd = "summaryAdd"; //$NON-NLS-1$
/**
* Core event type for removing a summary to a topic (value is
* 'summaryRemove').
*
*
* - Source:
* - {@link org.xmind.core.ITopic}
* - Target:
* - the removed {@link org.xmind.core.IBoundary}
*
*/
public static final String SummaryRemove = "summaryRemove"; //$NON-NLS-1$
/**
* Core event type for adding a style to a style sheet (value is
* 'styleAdd').
*
*
* - Source:
* - {@link org.xmind.core.style.IStyleSheet}
* - Target:
* - the added {@link org.xmind.core.style.IStyle}
*
*/
public static final String StyleAdd = "styleAdd"; //$NON-NLS-1$
/**
* Core event type for removing a style from a style sheet (value is
* 'styleRemove').
*
*
* - Source:
* - {@link org.xmind.core.style.IStyleSheet}
* - Target:
* - the removed {@link org.xmind.core.style.IStyle}
*
*/
public static final String StyleRemove = "styleRemove"; //$NON-NLS-1$
/**
* Core event type for modifying a the name (value is 'name).
*
* - Source:
* - {@link org.xmind.core.INamed}
* - OldValue:
* - the old name ({@link String}), or
null
indicating that
* the name was unspecified
* - NewValue:
* - the new name ({@link String}), or
null
indicating that
* the name is unspecified
*
*/
public static final String Name = "name"; //$NON-NLS-1$
/**
* Core event type for modifying a property (value is 'property').
*
* - Source:
* - {@link org.xmind.core.IProperties}
* - Target:
* - the property name ({@link String})
* - OldValue:
* - the old property value ({@link String}), or
null
* indicating that the property was unspecified
* - NewValue:
* - the new property value ({@link String}), or
null
* indicating that the property is unspecified
*
*/
public static final String Property = "property"; //$NON-NLS-1$
/**
* Core event type for modifying a sheet's theme id (value is 'themeId').
*
* - Source:
* - {@link org.xmind.core.ISheet}
* - OldValue:
* - the old theme id ({@link String}), or
null
indicating
* that the theme id was unspecified
* - NewValue:
* - the new theme id ({@link String}), or
null
indicating
* that the theme id is unspecified
*
*/
public static final String ThemeId = "themeId"; //$NON-NLS-1$
/**
* Core event type for modifying the structure class of a topic (value is
* 'structureClass').
*
* - Source:
* - {@link org.xmind.core.ITopic}
* - OldValue:
* - the old structure class ({@link String}), or
null
* indicating that the structure class was unspecified
* - NewValue:
* - the new structure class ({@link String}), or
null
* indicating that the structure class is unspecified
*
*/
public static final String StructureClass = "structureClass"; //$NON-NLS-1$
/**
* Core event type for modifying the note content of a topic (value is
* 'topicNotes').
*
* - Source:
* - {@link org.xmind.core.ITopic}
* - Target:
* - the note format (either {@link org.xmind.core.INotes#PLAIN} or
* {@link org.xmind.core.INotes#HTML})
* - OldValue:
* - the old notes content ({@link org.xmind.core.INotesContent}), or
*
null
indicating that the note content was unspecified
* - NewValue:
* - the new notes content ({@link org.xmind.core.INotesContent}), or
*
null
indicating that the note content is unspecified
*
*
* @see org.xmind.core.INotes#PLAIN
* @see org.xmind.core.INotes#HTML
* @see org.xmind.core.INotes#getContent(String)
* @see org.xmind.core.INotes#setContent(String, INotesContent)
*/
public static final String TopicNotes = "topicNotes"; //$NON-NLS-1$
/**
* Core event type for modifying the labels (value is 'labels').
*
* - Source:
* - {@link org.xmind.core.ILabeled}
* - OldValue:
* - a {@link java.util.Set} of
String
containing all the old
* labels (may be empty, but is never null)
* - NewValue:
* - a {@link java.util.Set} of
String
containing all the new
* labels (may be empty, but is never null)
*
*/
public static final String Labels = "labels"; //$NON-NLS-1$
/**
* Core event type for changes of the resource references (value is
* 'resourceRefs').
*
* - Source:
* - {@link org.xmind.core.util.IRefCounter}
* - OldValue:
* - a {@link java.util.Collection} of
String
containing all
* the old resource references (may be empty, but is never null)
* - NewValue:
* - a {@link java.util.Collection} of
String
containing all
* the new resource references (may be empty, but is never null)
*
*/
public static final String ResourceRefs = "resourceRefs"; //$NON-NLS-1$
/**
* Core event type for modifying the source url of an image (value is
* 'imageSource').
*
* - Source:
* - {@link org.xmind.core.IImage}
* - OldValue:
* - the old source url of the image ({@link String}), or
*
null
indicating that the source url was unspecified
* - NewValue:
* - the new source url of the image ({@link String}), or
*
null
indicating that the source url is unspecified
*
*/
public static final String ImageSource = "imageSource"; //$NON-NLS-1$
/**
* Core event type for modifying the width of an image (value is
* 'imageWidth').
*
* - Source:
* - {@link org.xmind.core.IImage}
* - OldValue:
* - the old width of the image ({@link Integer}), or
null
* indicating that the width was unspecified
* - NewValue:
* - the new width of the image ({@link String}), or
null
* indicating that the width is unspecified
*
*/
public static final String ImageWidth = "imageWidth"; //$NON-NLS-1$
/**
* Core event type for modifying the height of an image (value is
* 'imageHeight').
*
* - Source:
* - {@link org.xmind.core.IImage}
* - OldValue:
* - the old height of the image ({@link Integer}), or
null
* indicating that the height was unspecified
* - NewValue:
* - the new height of the image ({@link String}), or
null
* indicating that the height is unspecified
*
*/
public static final String ImageHeight = "imageHeight"; //$NON-NLS-1$
/**
* Core event type for modifying the alignment of an image (value is
* 'imageAlignment').
*
* - Source:
* - {@link org.xmind.core.IImage}
* - OldValue:
* - the old alignment of the image ({@link Integer}), or
*
null
indicating that the alignment was unspecified
* - NewValue:
* - the new alignment of the image ({@link String}), or
null
* indicating that the alignment is unspecified
*
*/
public static final String ImageAlignment = "imageAlignment"; //$NON-NLS-1$
/**
* Core event type for modifying the visiblity of a legend (value is
* 'visibility').
*
* - Source:
* - {@link org.xmind.core.ILegend}
* - OldValue:
* - the old visibility ({@link Boolean})
* - NewValue:
* - the new visibility ({@link Boolean})
*
*/
public static final String Visibility = "visibility"; //$NON-NLS-1$
/**
* Core event type for modifying the description of a marker on a sheet
* (value is 'markerDescription').
*
* - Source:
* - {@link org.xmind.core.ILegend}
* - Target:
* - the marker's id ({@link String})
* - OldValue:
* - the old description ({@link String}) of the target marker, or
*
null
indicating that the description was unspecified
* - NewValue:
* - the new description ({@link String}) of the target marker, or
*
null
indicating that the description is unspecified
*
*/
public static final String MarkerDescription = "markerDescription"; //$NON-NLS-1$
public static final String NumberFormat = "numberingFormat"; //$NON-NLS-1$
public static final String NumberingPrefix = "numberingPrefix"; //$NON-NLS-1$
public static final String NumberingSuffix = "numberingSuffix"; //$NON-NLS-1$
public static final String NumberPrepending = "parentNumberingPrepending"; //$NON-NLS-1$
/**
* Core event type for changing the password of a workbook (value is
* 'passwordChange').
*
* - Source:
* - {@link org.xmind.core.IWorkboook}
* - OldValue:
* - the old password ({@link String}) of the workbook, or
*
null
indicating that the password was unspecified
* - NewValue:
* - the new password ({@link String}) of the workbook, or
*
null
indicating that the password is unspecified
*
*
* @see org.xmind.core.IWorkbook#setPassword(String)
* @see org.xmind.core.IWorkbook#getPassword()
*/
public static final String PasswordChange = "passwordChange"; //$NON-NLS-1$
/**
* Core event type for going to save a workbook (value is
* 'workbookPreSave'). This type of events is dispatched before a workbook
* is saved. Listening to this type of events allows modification to the
* workbook content before saving it to the destination. The most common
* usage is to save preview pictures by the UI, for we can't generate a
* preview picture without a graphical environment.
*
*
* - Source:
* - {@link org.xmind.core.IWorkboook}
*
*
* @see org.xmind.core.IWorkbook#save()
* @see org.xmind.core.IWorkbook#save(org.xmind.core.io.IOutputTarget)
* @see org.xmind.core.IWorkbook#save(java.io.OutputStream)
* @see org.xmind.core.IWorkbook#save(String)
*/
public static final String WorkbookPreSave = "workbookPreSave"; //$NON-NLS-1$
/**
* Core event type for going to save a workbook (value is
* 'workbookPreSaveOnce'). Similar to {@link #WorkbookPreSave}, but
* listeners to this type events are notified only once and removed from the
* event list thereafter. This type of events are commonly used when some
* pending work that may modify the content of a workbook should be counted
* before saving the workbook.
*
*
* - Source:
* - {@link org.xmind.core.IWorkboook}
*
*
* @see org.xmind.core.IWorkbook#save()
* @see org.xmind.core.IWorkbook#save(org.xmind.core.io.IOutputTarget)
* @see org.xmind.core.IWorkbook#save(java.io.OutputStream)
* @see org.xmind.core.IWorkbook#save(String)
*/
public static final String WorkbookPreSaveOnce = "workbookPreSaveOnce"; //$NON-NLS-1$
/**
* Core event type for having saved a workbook (value is 'workbookSave').
* This type of events is dispatched after a workbook is saved.
*
*
* - Source:
* - {@link org.xmind.core.IWorkboook}
*
*
*
* @see org.xmind.core.IWorkbook#save()
* @see org.xmind.core.IWorkbook#save(org.xmind.core.io.IOutputTarget)
* @see org.xmind.core.IWorkbook#save(java.io.OutputStream)
* @see org.xmind.core.IWorkbook#save(String)
*/
public static final String WorkbookSave = "workbookSave"; //$NON-NLS-1$
/**
* Core event for the topic was modified.
*/
public static final String ModifyTime = "modifyTime"; //$NON-NLS-1$
/**
* Core event type for adding a revision to the revision manager (value is
* 'revisionAdd').
*
*
* - Source:
* - the parent {@link org.xmind.core.IRevisionManager}
* - Target:
* - the child {@link org.xmind.core.IRevision}
* - Data:
* - Corresponding sheet ID
*
*/
public static final String RevisionAdd = "revisionAdd"; //$NON-NLS-1$
/**
* Core event type for removing a revision from the revision manager (value
* is 'revisionRemove').
*
*
* - Source:
* - the parent {@link org.xmind.core.IRevisionManager}
* - Target:
* - the child {@link org.xmind.core.IRevision}
* - Data:
* - Corresponding sheet ID
*
*/
public static final String RevisionRemove = "revisionRemove"; //$NON-NLS-1$
/**
* Core event type for metadata change in {@link org.xmind.core.IMeta}
* (value is 'metadata').
*
*
* - Source:
* - {@link org.xmind.core.IMeta}
* - Target:
* - a {@link String} representing the key of the modified metadata
* - OldValue:
* - the old {@link String} value of the metadata, or
null
to
* indicate this metadata was created
* - NewValue:
* - the new {@link String} value of the metadata, or
null
to
* indicate this metadata was deleted
*
*/
public static final String Metadata = "metadata"; //$NON-NLS-1$
/**
* Core event type for attribute change in {@link org.xmind.core.IMetaData}
* (value is 'metadataAttribute').
*
*
* - Source:
* - {@link org.xmind.core.IMeta}
* - Target:
* - a {@link String} representing the key of the modified metadata
* - Data:
* - a {@link String} representing the name of the modified attribute
* - OldValue:
* - the old {@link String} value of the attribute, or
null
* to indicate this attribute was added
* - NewValue:
* - the new {@link String} value of the attribute, or
null
* to indicate this attribute was removed
*/
public static final String MetadataAttribute = "metadataAttribute"; //$NON-NLS-1$
// /**
// * Core event type for adding one or more labels (value is 'labelAdd').
// *
// * - Source:
// * - a {@link org.xmind.core.ILabeled labeled object}
// * - Target:
// * - a {@link java.util.Set}
<String>
containing the
// * added labels
// *
// */
// public static final String LabelAdd = "labelAdd"; //$NON-NLS-1$
//
// /**
// * Core event type for removing one or more labels (value is 'labelRemove').
// *
// * - Source:
// * - a {@link org.xmind.core.ILabeled labeled object}
// * - Target:
// * - a {@link java.util.Set}
<String>
containing the
// * removed labels
// *
// */
// public static final String LabelRemove = "labelRemove"; //$NON-NLS-1$
/**
* Error constants indicating that an unknown error occurs (value=1).
*/
public static final int ERROR_UNKNOWN = 1;
/**
* Error constants indicating that a null argument is passed in (value=2).
*/
public static final int ERROR_NULL_ARGUMENT = 2;
/**
* Error constants indicating that an invalid argument is passed in
* (value=3).
*/
public static final int ERROR_INVALID_ARGUMENT = 3;
public static final int ERROR_INVALID_FILE = 10;
public static final int ERROR_NO_SUCH_ENTRY = 11;
public static final int ERROR_FAIL_ACCESS_XML_PARSER = 12;
public static final int ERROR_FAIL_PARSING_XML = 13;
public static final int ERROR_NO_WORKBOOK_CONTENT = 14;
public static final int ERROR_FAIL_ACCESS_XML_TRANSFORMER = 15;
public static final int ERROR_FAIL_INIT_CRYPTOGRAM = 16;
public static final int ERROR_WRONG_PASSWORD = 17;
public static final int ERROR_CANCELLATION = 100;
/**
* Media type for a textual file (value='text/xml').
*
* @see org.xmind.core.IFileEntry#getMediaType()
*/
public static final String MEDIA_TYPE_TEXT_XML = "text/xml"; //$NON-NLS-1$
/**
* Media type for an image file (value='image/png').
*
* @see org.xmind.core.IFileEntry#getMediaType()
*/
public static final String MEDIA_TYPE_IMAGE_PNG = "image/png"; //$NON-NLS-1$
private Core() {
}
public static IIdFactory getIdFactory() {
return getInternal().getIdFactory();
}
public static IWorkbookBuilder getWorkbookBuilder() {
return getInternal().getWorkbookBuilder();
}
public static IWorkspace getWorkspace() {
return getInternal().getWorkspace();
}
public static IMarkerSheetBuilder getMarkerSheetBuilder() {
return getInternal().getMarkerSheetBuilder();
}
public static Comparator getTopicComparator() {
return getInternal().getTopicComparator();
}
public static IStyleSheetBuilder getStyleSheetBuilder() {
return getInternal().getStyleSheetBuilder();
}
public static final String getCurrentVersion() {
return getInternal().getCurrentVersion();
}
public static final ILogger getLogger() {
return getInternal().getLogger();
}
private static InternalCore getInternal() {
return InternalCore.getInstance();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy