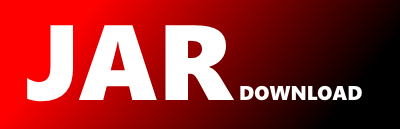
jalse.actions.MultiAction Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of JALSE Show documentation
Show all versions of JALSE Show documentation
Java Artificial Life Simulation Engine
package jalse.actions;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
class MultiAction implements Action {
private class ActionsAndOperation {
private final Collection extends Action> actions;
private final MultiActionOperation operation;
private ActionsAndOperation(final Collection extends Action> actions, final MultiActionOperation operation) {
this.actions = actions;
this.operation = operation;
}
}
enum MultiActionOperation {
PERFORM, SCHEDULE, SCHEDULE_AWAIT
}
private final List operations;
MultiAction() {
operations = new ArrayList<>();
}
public void addOperation(final Action action, final MultiActionOperation operation) {
operations.add(new ActionsAndOperation(Collections.singletonList(action), operation));
}
public void addOperation(final Collection extends Action> actions, final MultiActionOperation operation) {
operations.add(new ActionsAndOperation(actions, operation));
}
public boolean hasOperations() {
return !operations.isEmpty();
}
@Override
public void perform(final ActionContext context) throws InterruptedException {
for (final ActionsAndOperation aao : operations) {
switch (aao.operation) {
case PERFORM:
performAll(context, aao.actions);
break;
case SCHEDULE:
scheduleAll(context, aao.actions);
break;
case SCHEDULE_AWAIT:
scheduleAwaitAll(context, aao.actions);
break;
}
}
}
private void performAll(final ActionContext context, final Collection extends Action> actions)
throws InterruptedException {
for (final Action action : actions) {
action.perform(context); // Execute action
}
}
private Collection> scheduleAll(final ActionContext context,
final Collection extends Action> actions) {
final Collection> newContexts = new ArrayList<>();
final ActionEngine engine = context.getEngine();
final T actor = context.getActor();
for (final Action action : actions) {
final MutableActionContext newContext = engine.newContext(action);
newContext.setActor(actor); // Same actor
newContext.putAll(context.toMap()); // Copy bindings (current)
newContext.schedule();
newContexts.add(newContext);
}
return newContexts;
}
private void scheduleAwaitAll(final ActionContext context, final Collection extends Action> actions)
throws InterruptedException {
for (final MutableActionContext newContext : scheduleAll(context, actions)) {
if (!newContext.isDone()) { // Stops us for waiting forever
newContext.await();
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy