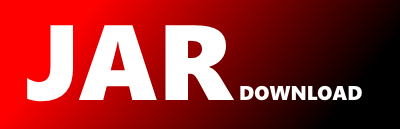
jalse.actions.UnmodifiableDelegateActionContext Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of JALSE Show documentation
Show all versions of JALSE Show documentation
Java Artificial Life Simulation Engine
package jalse.actions;
import java.util.concurrent.TimeUnit;
class UnmodifiableDelegateActionContext extends UnmodifiableDelegateActionBindings implements
MutableActionContext {
private final MutableActionContext delegate;
UnmodifiableDelegateActionContext(final MutableActionContext delegate) {
super(delegate);
this.delegate = delegate;
}
@Override
public void await() throws InterruptedException {
if (delegate != null) {
delegate.await();
}
}
@Override
public boolean cancel() {
return delegate != null ? delegate.cancel() : false;
}
@Override
public Action getAction() {
return delegate != null ? delegate.getAction() : null;
}
@Override
public T getActor() {
return delegate != null ? delegate.getActor() : null;
}
@Override
public ActionEngine getEngine() {
return delegate != null ? delegate.getEngine() : null;
}
@Override
public long getInitialDelay(final TimeUnit unit) {
return delegate != null ? delegate.getInitialDelay(unit) : 0L;
}
@Override
public long getPeriod(final TimeUnit unit) {
return delegate != null ? delegate.getPeriod(unit) : 0L;
}
@Override
public boolean isCancelled() {
return delegate != null ? delegate.isCancelled() : false;
}
@Override
public boolean isDone() {
return delegate != null ? delegate.isDone() : true;
}
@Override
public void schedule() {
throw new UnsupportedOperationException();
}
@Override
public void scheduleAndAwait() throws InterruptedException {
throw new UnsupportedOperationException();
}
@Override
public void setActor(final T actor) {
throw new UnsupportedOperationException();
}
@Override
public void setInitialDelay(final long initialDelay, final TimeUnit unit) {
throw new UnsupportedOperationException();
}
@Override
public void setPeriod(final long period, final TimeUnit unit) {
throw new UnsupportedOperationException();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy