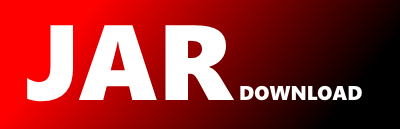
jalse.actions.DefaultActionBindings Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of JALSE Show documentation
Show all versions of JALSE Show documentation
Java Artificial Life Simulation Engine
package jalse.actions;
import java.util.HashMap;
import java.util.Map;
import java.util.Objects;
import java.util.concurrent.ConcurrentHashMap;
import java.util.concurrent.ConcurrentMap;
/**
* This is a simple yet fully-featured implementation of {@link MutableActionBindings}.
*
* Keys cannot be null or empty.
*
* @author Elliot Ford
*
*/
@SuppressWarnings("unchecked")
public class DefaultActionBindings implements MutableActionBindings {
private static void validateKey(final String key) {
if (key == null || key.length() == 0) {
throw new IllegalArgumentException();
}
}
private final ConcurrentMap bindings;
/**
* Creates a new instance of DefaultActionBindings.
*/
public DefaultActionBindings() {
bindings = new ConcurrentHashMap<>();
}
/**
* Creates a new instance of DefaultActionBindings with the supplied source bindings.
*
* @param sourceBindings
* Source bindings to shallow copy.
*/
public DefaultActionBindings(final ActionBindings sourceBindings) {
this(sourceBindings.toMap());
}
/**
* Creates a new instance of DefaultActionBindings with the supplied key-value pairs.
*
* @param map
* Key-value pairs to add.
*
*/
public DefaultActionBindings(final Map map) {
this();
putAll(map);
}
@Override
public T get(final String key) {
validateKey(key);
return (T) bindings.get(key);
}
@Override
public T put(final String key, final T value) {
validateKey(key);
return (T) bindings.put(key, Objects.requireNonNull(value));
}
@Override
public void putAll(final Map map) {
map.entrySet().forEach(e -> {
put(e.getKey(), e.getValue());
});
}
@Override
public T remove(final String key) {
validateKey(key);
return (T) bindings.remove(key);
}
@Override
public void removeAll() {
bindings.clear();
}
@Override
public Map toMap() {
return new HashMap<>(bindings);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy