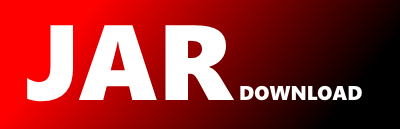
jalse.entities.methods.GetEntityMethod Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of JALSE Show documentation
Show all versions of JALSE Show documentation
Java Artificial Life Simulation Engine
package jalse.entities.methods;
import jalse.entities.Entity;
import jalse.entities.EntityContainer;
import jalse.entities.annotations.GetEntity;
import jalse.entities.functions.GetEntityFunction;
import java.util.Collections;
import java.util.Objects;
import java.util.Set;
import java.util.UUID;
import java.util.function.Supplier;
/**
* This is used for mapping calls to:
*
* - {@link EntityContainer#getEntityAsType(UUID, Class)}
* - {@link EntityContainer#getOptEntityAsType(UUID, Class)}
*
*
* @author Elliot Ford
*
* @see GetEntity
* @see GetEntityFunction
*
*/
public class GetEntityMethod implements EntityMethod {
private final Class extends Entity> type;
private Supplier idSupplier;
private final boolean optional;
private final boolean entityType;
/**
* Creates a get entity method.
*
* @param type
* Entity type.
* @param optional
* Whether is opt.
*/
public GetEntityMethod(final Class extends Entity> type, final boolean optional) {
this.type = Objects.requireNonNull(type);
entityType = Entity.class.equals(type);
this.optional = optional;
idSupplier = null;
}
@Override
public Set> getDependencies() {
return Collections.singleton(type);
}
/**
* Gets entity ID supplier.
*
* @return ID supplier.
*/
public Supplier getIDSupplier() {
return idSupplier;
}
/**
* Gets the entity type.
*
* @return Entity return type.
*/
public Class extends Entity> getType() {
return type;
}
@Override
public Object invoke(final Object proxy, final Entity entity, final Object[] args) throws Throwable {
// Get entity ID
final UUID idArg = idSupplier != null ? idSupplier.get() : (UUID) args[0];
// Check optional
if (optional) {
return entityType ? entity.getOptEntity(idArg) : entity.getOptEntityAsType(idArg, type);
} else {
return entityType ? entity.getEntity(idArg) : entity.getEntityAsType(idArg, type);
}
}
/**
* Checks whether this is an opt method.
*
* @return Opt method.
*/
public boolean isOptional() {
return optional;
}
/**
* Sets the entity ID supplier.
*
* @param idSupplier
*/
public void setIDSupplier(final Supplier idSupplier) {
this.idSupplier = idSupplier;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy