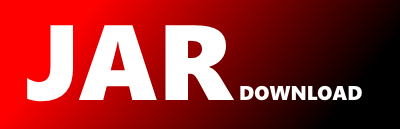
jalse.actions.ThreadPoolActionEngine Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of JALSE Show documentation
Show all versions of JALSE Show documentation
Java Artificial Life Simulation Engine
package jalse.actions;
import java.util.concurrent.ScheduledThreadPoolExecutor;
import java.util.concurrent.TimeUnit;
import java.util.logging.Level;
import java.util.logging.Logger;
/**
* An implementation of {@link ActionEngine} based on {@link ScheduledThreadPoolExecutor}.
*
* @author Elliot Ford
*
*/
public class ThreadPoolActionEngine extends AbstractActionEngine {
/**
* Thread pool context.
*
* @author Elliot Ford
*
* @param
* Actor type.
*/
public class ThreadPoolContext extends AbstractFutureActionContext implements Runnable {
/**
* Creates new instance of ThreadPoolContext.
*
* @param action
* Action this context is for.
*/
protected ThreadPoolContext(final Action action) {
super(ThreadPoolActionEngine.this, action, getBindings());
}
@Override
public void run() {
try {
getAction().perform(this); // Execute action
} catch (final InterruptedException e) {
Thread.currentThread().interrupt();
cancel(); // Just to be sure
} catch (final Exception e) {
logger.log(Level.WARNING, "Error performing action", e);
}
}
@Override
public void schedule() {
if (!isDone()) {
final ScheduledThreadPoolExecutor stpe = (ScheduledThreadPoolExecutor) executorService;
final long initialDelay = getInitialDelay(TimeUnit.NANOSECONDS);
if (isPeriodic()) {
setFuture(stpe.scheduleAtFixedRate(this, initialDelay, getPeriod(TimeUnit.NANOSECONDS),
TimeUnit.NANOSECONDS)); // At rate
} else {
setFuture(stpe.schedule(this, initialDelay, TimeUnit.NANOSECONDS));
}
}
}
}
private static final Logger logger = Logger.getLogger(ThreadPoolActionEngine.class.getName());
/**
* Creates a new instance of ThreadPoolActionEngine with the supplied core pool size.
*
* @param corePoolSize
* Number of threads to process actions using.
*/
public ThreadPoolActionEngine(final int corePoolSize) {
super(new ScheduledThreadPoolExecutor(corePoolSize));
}
@Override
public ThreadPoolContext newContext(final Action action) {
return new ThreadPoolContext<>(action);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy