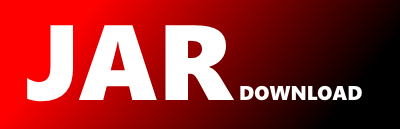
jalse.attributes.UnmodifiableDelegateAttributeContainer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of JALSE Show documentation
Show all versions of JALSE Show documentation
Java Artificial Life Simulation Engine
package jalse.attributes;
import java.util.Collections;
import java.util.Set;
import java.util.stream.Stream;
class UnmodifiableDelegateAttributeContainer implements AttributeContainer {
private final AttributeContainer delegate;
UnmodifiableDelegateAttributeContainer(final AttributeContainer delegate) {
this.delegate = delegate;
}
@Override
public boolean addAttributeListener(final String name, final AttributeType type,
final AttributeListener listener) {
throw new UnsupportedOperationException();
}
@Override
public void fireAttributeChanged(final String name, final AttributeType type) {
throw new UnsupportedOperationException();
}
@Override
public T getAttribute(final String name, final AttributeType type) {
return delegate != null ? delegate.getAttribute(name, type) : null;
}
@Override
public int getAttributeCount() {
return delegate != null ? delegate.getAttributeCount() : 0;
}
@Override
public Set getAttributeListenerNames() {
return delegate != null ? delegate.getAttributeListenerNames() : Collections.emptySet();
}
@Override
public Set extends AttributeListener> getAttributeListeners(final String name, final AttributeType type) {
return delegate != null ? delegate.getAttributeListeners(name, type) : Collections.emptySet();
}
@Override
public Set> getAttributeListenerTypes(final String name) {
return delegate != null ? delegate.getAttributeListenerTypes(name) : Collections.emptySet();
}
@Override
public Set getAttributeNames() {
return delegate != null ? delegate.getAttributeNames() : Collections.emptySet();
}
@Override
public Set> getAttributes() {
return delegate != null ? delegate.getAttributes() : Collections.emptySet();
}
@Override
public Set> getAttributeTypes(final String name) {
return delegate != null ? delegate.getAttributeTypes(name) : Collections.emptySet();
}
@Override
public T removeAttribute(final String name, final AttributeType type) {
throw new UnsupportedOperationException();
}
@Override
public boolean removeAttributeListener(final String name, final AttributeType type,
final AttributeListener listener) {
throw new UnsupportedOperationException();
}
@Override
public void removeAttributeListeners() {
throw new UnsupportedOperationException();
}
@Override
public void removeAttributeListeners(final String name, final AttributeType type) {
throw new UnsupportedOperationException();
}
@Override
public void removeAttributes() {
throw new UnsupportedOperationException();
}
@Override
public T setAttribute(final String name, final AttributeType type, final T attr) {
throw new UnsupportedOperationException();
}
@Override
public Stream> streamAttributes() {
return delegate != null ? delegate.streamAttributes() : Stream.empty();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy