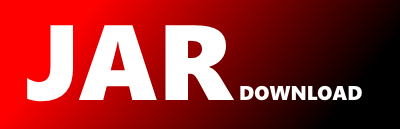
com.elopteryx.paint.upload.rs.UploadReader Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of paint-upload-jaxrs Show documentation
Show all versions of paint-upload-jaxrs Show documentation
Paint Upload Jax-Rs is a file upload library for Jax-Rs applications.
The newest version!
/*
* Copyright (C) 2015 Adam Forgacs
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.elopteryx.paint.upload.rs;
import com.elopteryx.paint.upload.OnPartBegin;
import com.elopteryx.paint.upload.OnPartEnd;
import com.elopteryx.paint.upload.internal.Headers;
import com.elopteryx.paint.upload.rs.internal.MultiPartImpl;
import com.elopteryx.paint.upload.rs.internal.RestUploadParser;
import java.io.InputStream;
import java.io.IOException;
import java.lang.annotation.Annotation;
import java.lang.reflect.ParameterizedType;
import java.lang.reflect.Type;
import java.util.Collection;
import java.util.List;
import javax.annotation.Nullable;
import javax.ws.rs.Consumes;
import javax.ws.rs.WebApplicationException;
import javax.ws.rs.core.MediaType;
import javax.ws.rs.core.MultivaluedMap;
import javax.ws.rs.ext.MessageBodyReader;
/**
* This class is a message body reader for multipart requests. It works like the blocking
* upload parser. It was made abstract to make sure that it will be properly configured.
* Actual readers should extend this and implement the callback interfaces,
* which are the part begin and end callbacks.
*
* The reader does not support the request callback, because that code can go
* into the controller method instead and the error callback, because there are
* exception mappers for that.
*
* The reader can inject the following parameters:
*
*
* - {@link MultiPart}
* - {@link Part} with a valid {@link UploadParam} annotation
* - {@link Collection} of {@link Part} instances
* - {@link List} of {@link Part} instances
*
*
* Other parameters are not supported by the reader.
*/
@Consumes(MediaType.MULTIPART_FORM_DATA)
public abstract class UploadReader implements MessageBodyReader
© 2015 - 2025 Weber Informatics LLC | Privacy Policy