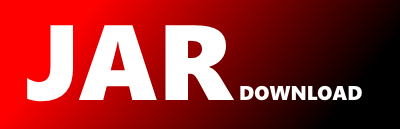
com.elopteryx.paint.upload.PartOutput Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of paint-upload Show documentation
Show all versions of paint-upload Show documentation
Paint Upload is an asynchronous file upload library for servlets.
The newest version!
/*
* Copyright (C) 2015 Adam Forgacs
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.elopteryx.paint.upload;
import java.io.OutputStream;
import java.nio.channels.WritableByteChannel;
import java.nio.file.Path;
import javax.annotation.CheckReturnValue;
import javax.annotation.Nonnull;
/**
* A value holder class, allowing the caller to provide
* various output objects, like a byte channel or an output stream.
*/
public class PartOutput {
/**
* The value object.
*/
private final Object value;
/**
* Protected constructor, no need for public access.
* The parser will use the given object here, which is why using
* the static factory methods is encouraged. Passing an invalid
* object will terminate the upload process.
* @param value The value object.
*/
protected PartOutput(Object value) {
this.value = value;
}
/**
* Returns whether it is safe to retrieve the value object
* with the class parameter.
* @param clazz The class type to check
* @param Type parameter
* @return Whether it is safe to cast or not
*/
@CheckReturnValue
public boolean safeToCast(Class clazz) {
return value != null && clazz.isAssignableFrom(value.getClass());
}
/**
* Retrieves the value object, casting it to the
* given type.
* @param clazz The class to cast
* @param Type parameter
* @return The stored value object
*/
@CheckReturnValue
public T unwrap(Class clazz) {
return clazz.cast(value);
}
/**
* Creates a new instance from the given channel object. The parser will
* use the channel to write out the bytes and will attempt to close it.
* @param byteChannel A channel which can be used for writing
* @return A new PartOutput instance
*/
public static PartOutput from(@Nonnull WritableByteChannel byteChannel) {
return new PartOutput(byteChannel);
}
/**
* Creates a new instance from the given stream object. The parser will
* create a channel from the stream to write out the bytes and
* will attempt to close it.
* @param outputStream A stream which can be used for writing
* @return A new PartOutput instance
*/
public static PartOutput from(@Nonnull OutputStream outputStream) {
return new PartOutput(outputStream);
}
/**
* Creates a new instance from the given path object. The parser will
* create a channel from the path to write out the bytes and
* will attempt to close it. If the file represented by the path
* does not exist, it will be created. If the file exists and is not
* empty then the uploaded bytes will be appended to the end of it.
* @param path A file path which can be used for writing
* @return A new PartOutput instance
*/
public static PartOutput from(@Nonnull Path path) {
return new PartOutput(path);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy