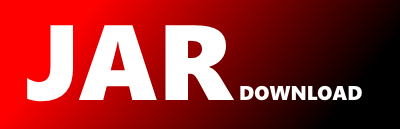
com.emc.mongoose.api.common.supply.async.AsyncUpdatingValueSupplier Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mongoose-api-common Show documentation
Show all versions of mongoose-api-common Show documentation
Mongoose is a high-load storage performance testing tool
package com.emc.mongoose.api.common.supply.async;
import com.github.akurilov.commons.concurrent.InitCallable;
import com.github.akurilov.coroutines.Coroutine;
import com.github.akurilov.coroutines.CoroutinesProcessor;
import com.github.akurilov.coroutines.ExclusiveCoroutineBase;
import com.emc.mongoose.api.common.exception.OmgDoesNotPerformException;
import com.emc.mongoose.api.common.supply.BasicUpdatingValueSupplier;
import java.io.IOException;
import java.util.List;
import java.util.logging.Level;
import java.util.logging.Logger;
/**
Created by kurila on 10.02.16.
*/
public class AsyncUpdatingValueSupplier
extends BasicUpdatingValueSupplier {
private static final Logger LOG = Logger.getLogger(AsyncUpdatingValueSupplier.class.getName());
private final Coroutine updateTask;
public AsyncUpdatingValueSupplier(
final CoroutinesProcessor coroutinesProcessor, final T initialValue,
final InitCallable updateAction
)
throws OmgDoesNotPerformException {
super(initialValue, null);
if(updateAction == null) {
throw new NullPointerException("Argument should not be null");
}
updateTask = new ExclusiveCoroutineBase(coroutinesProcessor) {
@Override
protected final void invokeTimedExclusively(final long startTimeNanos) {
try {
lastValue = updateAction.call();
} catch(final Exception e) {
LOG.log(Level.WARNING, "Failed to execute the value update action", e);
e.printStackTrace(System.err);
}
}
@Override
protected final void doClose()
throws IOException {
lastValue = null;
}
};
}
public static abstract class InitializedCallableBase
implements InitCallable {
@Override
public final boolean isInitialized() {
return true;
}
}
@Override
public final T get() {
// do not refresh on the request
return lastValue;
}
@Override
public final int get(final List buffer, final int limit) {
int count = 0;
for(; count < limit; count ++) {
buffer.add(lastValue);
}
return count;
}
@Override
public long skip(final long count) {
return 0;
}
@Override
public void close()
throws IOException {
super.close();
updateTask.close();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy