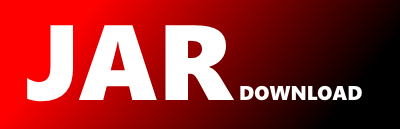
com.emc.mongoose.api.model.item.CsvItemInput Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mongoose-api-model Show documentation
Show all versions of mongoose-api-model Show documentation
Mongoose is a high-load storage performance testing tool
package com.emc.mongoose.api.model.item;
import com.emc.mongoose.api.common.Constants;
import com.github.akurilov.commons.io.Input;
import java.io.BufferedReader;
import java.io.EOFException;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.nio.charset.StandardCharsets;
import java.util.List;
/**
The data item input using CSV file containing the human-readable data item records as the source
*/
public class CsvItemInput
implements Input {
protected BufferedReader itemsSrc;
protected final ItemFactory itemFactory;
/**
@param in the input stream to get the data item records from
@param itemFactory the concrete item factory used to parse the records
@throws IOException
@throws NoSuchMethodException
*/
public CsvItemInput(final InputStream in, final ItemFactory itemFactory)
throws IOException, NoSuchMethodException {
this(
new BufferedReader(new InputStreamReader(in, StandardCharsets.UTF_8), Constants.MIB),
itemFactory
);
}
protected CsvItemInput(final BufferedReader itemsSrc, final ItemFactory itemFactory) {
setItemsSrc(itemsSrc);
this.itemFactory = itemFactory;
}
protected final void setItemsSrc(final BufferedReader itemsSrc) {
this.itemsSrc = itemsSrc;
}
@Override
public long skip(final long itemsCount)
throws IOException {
long i = 0;
while(i < itemsCount && null != itemsSrc.readLine()) {
i ++;
}
return i;
}
@Override
public I get()
throws IOException {
final String nextLine = itemsSrc.readLine();
try {
return nextLine == null ? null : itemFactory.getItem(nextLine);
} catch(final IllegalArgumentException e) {
e.printStackTrace(System.err);
return null;
}
}
@Override
public int get(final List buffer, final int limit)
throws IOException {
int i = 0;
String nextLine;
try {
while(i < limit) {
nextLine = itemsSrc.readLine();
if(nextLine == null) {
if(i == 0) {
throw new EOFException();
} else {
break;
}
}
buffer.add(itemFactory.getItem(nextLine));
i ++;
}
} catch(final IllegalArgumentException e) {
e.printStackTrace(System.err);
}
return i;
}
/**
Most probably will cause an IOException due to missing mark
@throws IOException
*/
@Override
public void reset()
throws IOException {
itemsSrc.reset();
}
@Override
public void close()
throws IOException {
itemsSrc.close();
}
@Override
public String toString() {
return "csvItemInput<" + itemsSrc.toString() + ">";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy