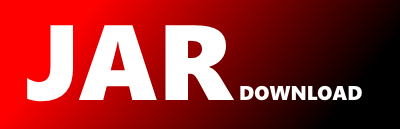
com.emc.mongoose.api.model.load.LoadGenerator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mongoose-api-model Show documentation
Show all versions of mongoose-api-model Show documentation
Mongoose is a high-load storage performance testing tool
package com.emc.mongoose.api.model.load;
import com.github.akurilov.commons.concurrent.Throttle;
import com.github.akurilov.commons.io.Output;
import com.emc.mongoose.api.model.concurrent.Daemon;
import com.emc.mongoose.api.common.concurrent.WeightThrottle;
import com.emc.mongoose.api.model.io.task.IoTask;
import com.emc.mongoose.api.model.item.Item;
import com.emc.mongoose.api.model.io.IoType;
import java.util.List;
/**
Created on 11.07.16.
*/
public interface LoadGenerator>
extends Daemon {
void setWeightThrottle(final WeightThrottle weightThrottle);
void setRateThrottle(final Throttle
© 2015 - 2025 Weber Informatics LLC | Privacy Policy