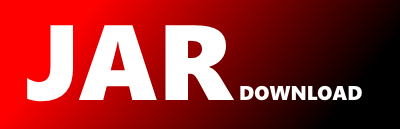
com.emc.mongoose.base.config.el.AsyncExpressionInputImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mongoose-base Show documentation
Show all versions of mongoose-base Show documentation
Mongoose is a high-load storage performance testing tool
package com.emc.mongoose.base.config.el;
import static com.github.akurilov.commons.lang.Exceptions.throwUnchecked;
import com.emc.mongoose.base.concurrent.ServiceTaskExecutor;
import com.github.akurilov.commons.io.el.ExpressionInput;
import com.github.akurilov.commons.io.el.SynchronousExpressionInput;
import com.github.akurilov.fiber4j.ExclusiveFiberBase;
import java.util.List;
import javax.el.ELException;
import javax.el.PropertyNotFoundException;
class AsyncExpressionInputImpl extends ExclusiveFiberBase implements AsyncExpressionInput {
private ExpressionInput input;
AsyncExpressionInputImpl(final ExpressionInput input) {
super(ServiceTaskExecutor.INSTANCE);
if (input instanceof SynchronousExpressionInput) {
throw new IllegalArgumentException("An expression input to wrap should not be synchronous");
}
this.input = input;
}
@Override
public final T call() {
return last();
}
@Override
public T last() {
return input.last();
}
@Override
public T get() throws PropertyNotFoundException, ELException {
return input.get();
}
@Override
public int get(final List buffer, final int limit)
throws PropertyNotFoundException, ELException {
return input.get(buffer, limit);
}
@Override
public long skip(final long count) {
return input.skip(count);
}
@Override
public void reset() {
input.reset();
}
@Override
public String expr() {
return input.expr();
}
@Override
protected final void invokeTimedExclusively(final long startTimeNanos) {
input.call();
}
@Override
protected final void doClose() {
try {
super.doClose();
input.close();
} catch (final Exception e) {
throwUnchecked(e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy