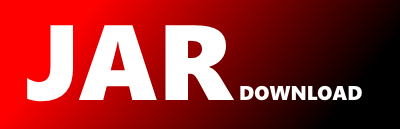
com.emc.mongoose.base.config.ConfigUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mongoose-base Show documentation
Show all versions of mongoose-base Show documentation
Mongoose is a high-load storage performance testing tool
package com.emc.mongoose.base.config;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.core.util.DefaultIndenter;
import com.fasterxml.jackson.core.util.DefaultPrettyPrinter;
import com.fasterxml.jackson.databind.DeserializationFeature;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.ObjectWriter;
import com.fasterxml.jackson.databind.SerializationFeature;
import com.fasterxml.jackson.dataformat.yaml.YAMLMapper;
import com.github.akurilov.commons.collection.TreeUtil;
import com.github.akurilov.confuse.Config;
import com.github.akurilov.confuse.impl.BasicConfig;
import java.io.File;
import java.io.IOException;
import java.util.Collections;
import java.util.List;
import java.util.Map;
import java.util.stream.Collectors;
import static com.emc.mongoose.base.config.ConfigFormat.JSON;
import static com.emc.mongoose.base.config.ConfigFormat.YAML;
public interface ConfigUtil {
static ObjectMapper readConfigMapper(final ConfigFormat format, final Map schema)
throws NoSuchMethodException {
final ObjectMapper mapper;
switch (format) {
case JSON:
mapper = new ObjectMapper();
break;
case YAML:
mapper = new YAMLMapper();
break;
default:
throw new AssertionError();
}
return mapper
.enable(DeserializationFeature.FAIL_ON_NULL_FOR_PRIMITIVES)
.enable(DeserializationFeature.FAIL_ON_READING_DUP_TREE_KEY)
.enable(SerializationFeature.INDENT_OUTPUT);
}
static ObjectWriter writerWithPrettyPrinter(final ObjectMapper om) {
final var indenter = (DefaultPrettyPrinter.Indenter) new DefaultIndenter(" ", DefaultIndenter.SYS_LF);
final var printer = new DefaultPrettyPrinter();
printer.indentObjectsWith(indenter);
printer.indentArraysWith(indenter);
om.enable(SerializationFeature.INDENT_OUTPUT);
return om.writer(printer);
}
static ObjectWriter configWriter(final ConfigFormat format) {
final ObjectMapper mapper;
switch (format) {
case JSON:
mapper = new ObjectMapper();
break;
case YAML:
mapper = new YAMLMapper();
break;
default:
throw new AssertionError();
}
return writerWithPrettyPrinter(mapper);
}
static Map configTree(final Config config) {
final var configCopy = (Config) new BasicConfig(config);
final var configTree = configCopy.mapVal(null);
for (final var e : configTree.entrySet()) {
final var key = e.getKey();
final var val = e.getValue();
if (val instanceof Config) {
configTree.replace(key, configTree((Config) val));
}
}
return configTree;
}
static String toString(final Config config, final ConfigFormat format) {
try {
final var configTree = configTree(config);
return configWriter(format).writeValueAsString(configTree);
} catch (final JsonProcessingException e) {
throw new RuntimeException(e);
}
}
static Config loadConfig(final File file, final Map schema)
throws NoSuchMethodException, IOException {
final ConfigFormat format;
if (file.getName().endsWith(".json")) {
format = JSON;
} else {
format = YAML;
}
final Map configTree = readConfigMapper(format, schema)
.readValue(file, new TypeReference
© 2015 - 2025 Weber Informatics LLC | Privacy Policy