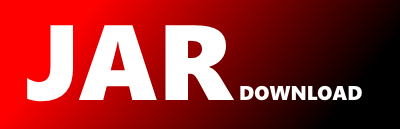
com.emc.mongoose.base.env.DateUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mongoose-base Show documentation
Show all versions of mongoose-base Show documentation
Mongoose is a high-load storage performance testing tool
package com.emc.mongoose.base.env;
import com.emc.mongoose.base.Constants;
import java.text.DateFormat;
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.HashMap;
import java.util.Locale;
import java.util.Map;
import java.util.TimeZone;
/** Created by andrey on 18.11.16. */
//TODO: update using formatters
public interface DateUtil {
TimeZone TZ_UTC = TimeZone.getTimeZone("UTC");
// e.g. 2001-07-04T12:08:56.235-0700
String PATTERN_ISO8601 = "yyyy-MM-dd'T'HH:mm:ss,SSS";
DateFormat FMT_DATE_ISO8601 = new SimpleDateFormat(PATTERN_ISO8601, Constants.LOCALE_DEFAULT) {
{
setTimeZone(TZ_UTC);
}
};
static String formatNowIso8601() {
return FMT_DATE_ISO8601.format(new Date(System.currentTimeMillis()));
}
// e.g. 20210901T182320Z
String PATTERN_AMAZON = "yyyyMMdd'T'HHmmss'Z'"; // close to ISO_INSTANT but not quite
DateFormat FMT_DATE_AMAZON = new SimpleDateFormat(PATTERN_AMAZON, Constants.LOCALE_DEFAULT) {
{
setTimeZone(TZ_UTC);
}
};
static String formatNowAmazonStyle() {
return FMT_DATE_AMAZON.format(new Date(System.currentTimeMillis()));
}
// e.g. Sun, 06 Nov 1994 08:49:37 GMT
String PATTERN_RFC1123 = "EEE, dd MMM yyyy HH:mm:ss zzz";
DateFormat FMT_DATE_RFC1123 = new SimpleDateFormat(PATTERN_RFC1123, Constants.LOCALE_DEFAULT) {
{
setTimeZone(TZ_UTC);
}
};
static String formatNowRfc1123() {
return FMT_DATE_RFC1123.format(new Date(System.currentTimeMillis()));
}
String PATTERN_METRICS_TABLE = "yyMMddHHmmss";
DateFormat FMT_DATE_METRICS_TABLE = new SimpleDateFormat(PATTERN_METRICS_TABLE, Constants.LOCALE_DEFAULT) {
{
setTimeZone(TZ_UTC);
}
};
ThreadLocal
© 2015 - 2025 Weber Informatics LLC | Privacy Policy