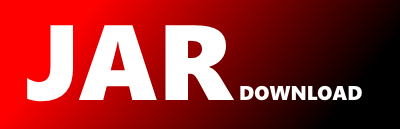
io.github.emm035.openapi.immutables.v3.OpenApi Maven / Gradle / Ivy
package io.github.emm035.openapi.immutables.v3;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonAutoDetect;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.google.common.base.MoreObjects;
import com.google.common.collect.ImmutableList;
import com.google.common.collect.ImmutableMap;
import com.google.common.collect.Lists;
import com.google.errorprone.annotations.CanIgnoreReturnValue;
import io.github.emm035.openapi.immutables.v3.metadata.ExternalDocumentation;
import io.github.emm035.openapi.immutables.v3.metadata.Info;
import io.github.emm035.openapi.immutables.v3.security.SecurityRequirement;
import io.github.emm035.openapi.immutables.v3.servers.Server;
import io.github.emm035.openapi.immutables.v3.shared.Extensible;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.CheckReturnValue;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
/**
* Immutable implementation of {@link AbstractOpenApi}.
*
* Use the builder to create immutable instances:
* {@code OpenApi.builder()}.
*/
@SuppressWarnings({"all"})
@ParametersAreNonnullByDefault
@Immutable
@CheckReturnValue
public final class OpenApi extends AbstractOpenApi {
private final String openApi;
private final Info info;
private final ImmutableList servers;
private final @Nullable Components components;
private final ImmutableList security;
private final ImmutableList tags;
private final @Nullable ExternalDocumentation externalDocs;
private final Paths paths;
private final ImmutableMap extensions;
private OpenApi(OpenApi.Builder builder) {
this.openApi = builder.openApi;
this.info = builder.info;
this.components = builder.components;
this.security = builder.security.build();
this.tags = builder.tags.build();
this.externalDocs = builder.externalDocs;
this.extensions = builder.extensions.build();
if (builder.serversIsSet()) {
initShim.setServers(builder.servers.build());
}
if (builder.paths != null) {
initShim.setPaths(builder.paths);
}
this.servers = initShim.getServers();
this.paths = initShim.getPaths();
this.initShim = null;
}
private OpenApi(
String openApi,
Info info,
ImmutableList servers,
@Nullable Components components,
ImmutableList security,
ImmutableList tags,
@Nullable ExternalDocumentation externalDocs,
Paths paths,
ImmutableMap extensions) {
this.openApi = openApi;
this.info = info;
this.servers = servers;
this.components = components;
this.security = security;
this.tags = tags;
this.externalDocs = externalDocs;
this.paths = paths;
this.extensions = extensions;
this.initShim = null;
}
private static final int STAGE_INITIALIZING = -1;
private static final int STAGE_UNINITIALIZED = 0;
private static final int STAGE_INITIALIZED = 1;
private transient volatile InitShim initShim = new InitShim();
private final class InitShim {
private ImmutableList servers;
private int serversBuildStage;
ImmutableList getServers() {
if (serversBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (serversBuildStage == STAGE_UNINITIALIZED) {
serversBuildStage = STAGE_INITIALIZING;
this.servers = ImmutableList.copyOf(OpenApi.super.getServers());
serversBuildStage = STAGE_INITIALIZED;
}
return this.servers;
}
void setServers(ImmutableList servers) {
this.servers = servers;
serversBuildStage = STAGE_INITIALIZED;
}
private Paths paths;
private int pathsBuildStage;
Paths getPaths() {
if (pathsBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (pathsBuildStage == STAGE_UNINITIALIZED) {
pathsBuildStage = STAGE_INITIALIZING;
this.paths = Objects.requireNonNull(OpenApi.super.getPaths(), "paths");
pathsBuildStage = STAGE_INITIALIZED;
}
return this.paths;
}
void setPaths(Paths paths) {
this.paths = paths;
pathsBuildStage = STAGE_INITIALIZED;
}
private String formatInitCycleMessage() {
ArrayList attributes = Lists.newArrayList();
if (serversBuildStage == STAGE_INITIALIZING) attributes.add("servers");
if (pathsBuildStage == STAGE_INITIALIZING) attributes.add("paths");
return "Cannot build OpenApi, attribute initializers form cycle" + attributes;
}
}
/**
* @return The value of the {@code openApi} attribute
*/
@JsonProperty("openapi")
@Override
public String getOpenApi() {
return openApi;
}
/**
* @return The value of the {@code info} attribute
*/
@JsonProperty("info")
@Override
public Info getInfo() {
return info;
}
/**
* @return The value of the {@code servers} attribute
*/
@JsonProperty("servers")
@Override
public ImmutableList getServers() {
InitShim shim = this.initShim;
return shim != null
? shim.getServers()
: this.servers;
}
/**
* @return The value of the {@code components} attribute
*/
@JsonProperty("components")
@Override
public Optional getComponents() {
return Optional.ofNullable(components);
}
/**
* @return The value of the {@code security} attribute
*/
@JsonProperty("security")
@Override
public ImmutableList getSecurity() {
return security;
}
/**
* @return The value of the {@code tags} attribute
*/
@JsonProperty("tags")
@Override
public ImmutableList getTags() {
return tags;
}
/**
* @return The value of the {@code externalDocs} attribute
*/
@JsonProperty("externalDocs")
@Override
public Optional getExternalDocs() {
return Optional.ofNullable(externalDocs);
}
/**
* @return The value of the {@code paths} attribute
*/
@JsonProperty("paths")
@Override
public Paths getPaths() {
InitShim shim = this.initShim;
return shim != null
? shim.getPaths()
: this.paths;
}
/**
* @return The value of the {@code extensions} attribute
*/
@JsonProperty("extensions")
@JsonAnyGetter
@Override
public ImmutableMap getExtensions() {
return extensions;
}
/**
* Copy the current immutable object by setting a value for the {@link AbstractOpenApi#getOpenApi() openApi} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for openApi
* @return A modified copy of the {@code this} object
*/
public final OpenApi withOpenApi(String value) {
if (this.openApi.equals(value)) return this;
String newValue = Objects.requireNonNull(value, "openApi");
return validate(new OpenApi(
newValue,
this.info,
this.servers,
this.components,
this.security,
this.tags,
this.externalDocs,
this.paths,
this.extensions));
}
/**
* Copy the current immutable object by setting a value for the {@link AbstractOpenApi#getInfo() info} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for info
* @return A modified copy of the {@code this} object
*/
public final OpenApi withInfo(Info value) {
if (this.info == value) return this;
Info newValue = Objects.requireNonNull(value, "info");
return validate(new OpenApi(
this.openApi,
newValue,
this.servers,
this.components,
this.security,
this.tags,
this.externalDocs,
this.paths,
this.extensions));
}
/**
* Copy the current immutable object with elements that replace the content of {@link AbstractOpenApi#getServers() servers}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final OpenApi withServers(Server... elements) {
ImmutableList newValue = ImmutableList.copyOf(elements);
return validate(new OpenApi(
this.openApi,
this.info,
newValue,
this.components,
this.security,
this.tags,
this.externalDocs,
this.paths,
this.extensions));
}
/**
* Copy the current immutable object with elements that replace the content of {@link AbstractOpenApi#getServers() servers}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of servers elements to set
* @return A modified copy of {@code this} object
*/
public final OpenApi withServers(Iterable extends Server> elements) {
if (this.servers == elements) return this;
ImmutableList newValue = ImmutableList.copyOf(elements);
return validate(new OpenApi(
this.openApi,
this.info,
newValue,
this.components,
this.security,
this.tags,
this.externalDocs,
this.paths,
this.extensions));
}
/**
* Copy the current immutable object by setting a present value for the optional {@link AbstractOpenApi#getComponents() components} attribute.
* @param value The value for components, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final OpenApi withComponents(@Nullable Components value) {
@Nullable Components newValue = value;
if (this.components == newValue) return this;
return validate(new OpenApi(
this.openApi,
this.info,
this.servers,
newValue,
this.security,
this.tags,
this.externalDocs,
this.paths,
this.extensions));
}
/**
* Copy the current immutable object by setting an optional value for the {@link AbstractOpenApi#getComponents() components} attribute.
* A shallow reference equality check is used on unboxed optional value to prevent copying of the same value by returning {@code this}.
* @param optional A value for components
* @return A modified copy of {@code this} object
*/
public final OpenApi withComponents(Optional extends Components> optional) {
@Nullable Components value = optional.orElse(null);
if (this.components == value) return this;
return validate(new OpenApi(
this.openApi,
this.info,
this.servers,
value,
this.security,
this.tags,
this.externalDocs,
this.paths,
this.extensions));
}
/**
* Copy the current immutable object with elements that replace the content of {@link AbstractOpenApi#getSecurity() security}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final OpenApi withSecurity(SecurityRequirement... elements) {
ImmutableList newValue = ImmutableList.copyOf(elements);
return validate(new OpenApi(
this.openApi,
this.info,
this.servers,
this.components,
newValue,
this.tags,
this.externalDocs,
this.paths,
this.extensions));
}
/**
* Copy the current immutable object with elements that replace the content of {@link AbstractOpenApi#getSecurity() security}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of security elements to set
* @return A modified copy of {@code this} object
*/
public final OpenApi withSecurity(Iterable extends SecurityRequirement> elements) {
if (this.security == elements) return this;
ImmutableList newValue = ImmutableList.copyOf(elements);
return validate(new OpenApi(
this.openApi,
this.info,
this.servers,
this.components,
newValue,
this.tags,
this.externalDocs,
this.paths,
this.extensions));
}
/**
* Copy the current immutable object with elements that replace the content of {@link AbstractOpenApi#getTags() tags}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final OpenApi withTags(Tag... elements) {
ImmutableList newValue = ImmutableList.copyOf(elements);
return validate(new OpenApi(
this.openApi,
this.info,
this.servers,
this.components,
this.security,
newValue,
this.externalDocs,
this.paths,
this.extensions));
}
/**
* Copy the current immutable object with elements that replace the content of {@link AbstractOpenApi#getTags() tags}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of tags elements to set
* @return A modified copy of {@code this} object
*/
public final OpenApi withTags(Iterable extends Tag> elements) {
if (this.tags == elements) return this;
ImmutableList newValue = ImmutableList.copyOf(elements);
return validate(new OpenApi(
this.openApi,
this.info,
this.servers,
this.components,
this.security,
newValue,
this.externalDocs,
this.paths,
this.extensions));
}
/**
* Copy the current immutable object by setting a present value for the optional {@link AbstractOpenApi#getExternalDocs() externalDocs} attribute.
* @param value The value for externalDocs, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final OpenApi withExternalDocs(@Nullable ExternalDocumentation value) {
@Nullable ExternalDocumentation newValue = value;
if (this.externalDocs == newValue) return this;
return validate(new OpenApi(
this.openApi,
this.info,
this.servers,
this.components,
this.security,
this.tags,
newValue,
this.paths,
this.extensions));
}
/**
* Copy the current immutable object by setting an optional value for the {@link AbstractOpenApi#getExternalDocs() externalDocs} attribute.
* A shallow reference equality check is used on unboxed optional value to prevent copying of the same value by returning {@code this}.
* @param optional A value for externalDocs
* @return A modified copy of {@code this} object
*/
public final OpenApi withExternalDocs(Optional extends ExternalDocumentation> optional) {
@Nullable ExternalDocumentation value = optional.orElse(null);
if (this.externalDocs == value) return this;
return validate(new OpenApi(
this.openApi,
this.info,
this.servers,
this.components,
this.security,
this.tags,
value,
this.paths,
this.extensions));
}
/**
* Copy the current immutable object by setting a value for the {@link AbstractOpenApi#getPaths() paths} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for paths
* @return A modified copy of the {@code this} object
*/
public final OpenApi withPaths(Paths value) {
if (this.paths == value) return this;
Paths newValue = Objects.requireNonNull(value, "paths");
return validate(new OpenApi(
this.openApi,
this.info,
this.servers,
this.components,
this.security,
this.tags,
this.externalDocs,
newValue,
this.extensions));
}
/**
* Copy the current immutable object by replacing the {@link AbstractOpenApi#getExtensions() extensions} map with the specified map.
* Nulls are not permitted as keys or values.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param entries The entries to be added to the extensions map
* @return A modified copy of {@code this} object
*/
public final OpenApi withExtensions(Map entries) {
if (this.extensions == entries) return this;
ImmutableMap newValue = ImmutableMap.copyOf(entries);
return validate(new OpenApi(
this.openApi,
this.info,
this.servers,
this.components,
this.security,
this.tags,
this.externalDocs,
this.paths,
newValue));
}
/**
* This instance is equal to all instances of {@code OpenApi} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof OpenApi
&& equalTo((OpenApi) another);
}
private boolean equalTo(OpenApi another) {
return openApi.equals(another.openApi)
&& info.equals(another.info)
&& servers.equals(another.servers)
&& Objects.equals(components, another.components)
&& security.equals(another.security)
&& tags.equals(another.tags)
&& Objects.equals(externalDocs, another.externalDocs)
&& paths.equals(another.paths)
&& extensions.equals(another.extensions);
}
/**
* Computes a hash code from attributes: {@code openApi}, {@code info}, {@code servers}, {@code components}, {@code security}, {@code tags}, {@code externalDocs}, {@code paths}, {@code extensions}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + openApi.hashCode();
h += (h << 5) + info.hashCode();
h += (h << 5) + servers.hashCode();
h += (h << 5) + Objects.hashCode(components);
h += (h << 5) + security.hashCode();
h += (h << 5) + tags.hashCode();
h += (h << 5) + Objects.hashCode(externalDocs);
h += (h << 5) + paths.hashCode();
h += (h << 5) + extensions.hashCode();
return h;
}
/**
* Prints the immutable value {@code OpenApi} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return MoreObjects.toStringHelper("OpenApi")
.omitNullValues()
.add("openApi", openApi)
.add("info", info)
.add("servers", servers)
.add("components", components)
.add("security", security)
.add("tags", tags)
.add("externalDocs", externalDocs)
.add("paths", paths)
.add("extensions", extensions)
.toString();
}
/**
* Utility type used to correctly read immutable object from JSON representation.
* @deprecated Do not use this type directly, it exists only for the Jackson-binding infrastructure
*/
@Deprecated
@JsonAutoDetect(fieldVisibility = JsonAutoDetect.Visibility.NONE)
static final class Json extends AbstractOpenApi {
@Nullable String openApi;
@Nullable Info info;
@Nullable List servers = ImmutableList.of();
boolean serversIsSet;
@Nullable Optional components = Optional.empty();
@Nullable List security = ImmutableList.of();
@Nullable List tags = ImmutableList.of();
@Nullable Optional externalDocs = Optional.empty();
@Nullable Paths paths;
final Map extensions = new HashMap();
@JsonProperty("openapi")
public void setOpenApi(String openApi) {
this.openApi = openApi;
}
@JsonProperty("info")
public void setInfo(Info info) {
this.info = info;
}
@JsonProperty("servers")
public void setServers(List servers) {
this.servers = servers;
this.serversIsSet = true;
}
@JsonProperty("components")
public void setComponents(Optional components) {
this.components = components;
}
@JsonProperty("security")
public void setSecurity(List security) {
this.security = security;
}
@JsonProperty("tags")
public void setTags(List tags) {
this.tags = tags;
}
@JsonProperty("externalDocs")
public void setExternalDocs(Optional externalDocs) {
this.externalDocs = externalDocs;
}
@JsonProperty("paths")
public void setPaths(Paths paths) {
this.paths = paths;
}
@JsonAnySetter
public void setExtensions(String key, Object value) {
this.extensions.put(key, value);
}
@Override
public String getOpenApi() { throw new UnsupportedOperationException(); }
@Override
public Info getInfo() { throw new UnsupportedOperationException(); }
@Override
public List getServers() { throw new UnsupportedOperationException(); }
@Override
public Optional getComponents() { throw new UnsupportedOperationException(); }
@Override
public List getSecurity() { throw new UnsupportedOperationException(); }
@Override
public List getTags() { throw new UnsupportedOperationException(); }
@Override
public Optional getExternalDocs() { throw new UnsupportedOperationException(); }
@Override
public Paths getPaths() { throw new UnsupportedOperationException(); }
@Override
public Map getExtensions() { throw new UnsupportedOperationException(); }
}
/**
* @param json A JSON-bindable data structure
* @return An immutable value type
* @deprecated Do not use this method directly, it exists only for the Jackson-binding infrastructure
*/
@Deprecated
@JsonCreator(mode = JsonCreator.Mode.DELEGATING)
static OpenApi fromJson(Json json) {
OpenApi.Builder builder = OpenApi.builder();
if (json.openApi != null) {
builder.setOpenApi(json.openApi);
}
if (json.info != null) {
builder.setInfo(json.info);
}
if (json.serversIsSet) {
builder.addAllServers(json.servers);
}
if (json.components != null) {
builder.setComponents(json.components);
}
if (json.security != null) {
builder.addAllSecurity(json.security);
}
if (json.tags != null) {
builder.addAllTags(json.tags);
}
if (json.externalDocs != null) {
builder.setExternalDocs(json.externalDocs);
}
if (json.paths != null) {
builder.setPaths(json.paths);
}
if (json.extensions != null) {
builder.putAllExtensions(json.extensions);
}
return builder.build();
}
private static OpenApi validate(OpenApi instance) {
instance = (OpenApi) instance.normalizeExtensions();
return instance;
}
/**
* Creates an immutable copy of a {@link AbstractOpenApi} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable OpenApi instance
*/
public static OpenApi copyOf(AbstractOpenApi instance) {
if (instance instanceof OpenApi) {
return (OpenApi) instance;
}
return OpenApi.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link OpenApi OpenApi}.
* @return A new OpenApi builder
*/
public static OpenApi.Builder builder() {
return new OpenApi.Builder();
}
/**
* Builds instances of type {@link OpenApi OpenApi}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@NotThreadSafe
public static final class Builder {
private static final long INIT_BIT_OPEN_API = 0x1L;
private static final long INIT_BIT_INFO = 0x2L;
private static final long OPT_BIT_SERVERS = 0x1L;
private long initBits = 0x3L;
private long optBits;
private @Nullable String openApi;
private @Nullable Info info;
private ImmutableList.Builder servers = ImmutableList.builder();
private @Nullable Components components;
private ImmutableList.Builder security = ImmutableList.builder();
private ImmutableList.Builder tags = ImmutableList.builder();
private @Nullable ExternalDocumentation externalDocs;
private @Nullable Paths paths;
private ImmutableMap.Builder extensions = ImmutableMap.builder();
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code io.github.emm035.openapi.immutables.v3.shared.Extensible} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder from(Extensible instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return this;
}
/**
* Fill a builder with attribute values from the provided {@code io.github.emm035.openapi.immutables.v3.AbstractOpenApi} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder from(AbstractOpenApi instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return this;
}
private void from(Object object) {
if (object instanceof Extensible) {
Extensible instance = (Extensible) object;
putAllExtensions(instance.getExtensions());
}
if (object instanceof AbstractOpenApi) {
AbstractOpenApi instance = (AbstractOpenApi) object;
Optional componentsOptional = instance.getComponents();
if (componentsOptional.isPresent()) {
setComponents(componentsOptional);
}
addAllSecurity(instance.getSecurity());
addAllServers(instance.getServers());
setOpenApi(instance.getOpenApi());
setPaths(instance.getPaths());
Optional externalDocsOptional = instance.getExternalDocs();
if (externalDocsOptional.isPresent()) {
setExternalDocs(externalDocsOptional);
}
setInfo(instance.getInfo());
addAllTags(instance.getTags());
}
}
/**
* Initializes the value for the {@link AbstractOpenApi#getOpenApi() openApi} attribute.
* @param openApi The value for openApi
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder setOpenApi(String openApi) {
this.openApi = Objects.requireNonNull(openApi, "openApi");
initBits &= ~INIT_BIT_OPEN_API;
return this;
}
/**
* Initializes the value for the {@link AbstractOpenApi#getInfo() info} attribute.
* @param info The value for info
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder setInfo(Info info) {
this.info = Objects.requireNonNull(info, "info");
initBits &= ~INIT_BIT_INFO;
return this;
}
/**
* Adds one element to {@link AbstractOpenApi#getServers() servers} list.
* @param element A servers element
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addServers(Server element) {
this.servers.add(element);
optBits |= OPT_BIT_SERVERS;
return this;
}
/**
* Adds elements to {@link AbstractOpenApi#getServers() servers} list.
* @param elements An array of servers elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addServers(Server... elements) {
this.servers.add(elements);
optBits |= OPT_BIT_SERVERS;
return this;
}
/**
* Sets or replaces all elements for {@link AbstractOpenApi#getServers() servers} list.
* @param elements An iterable of servers elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder setServers(Iterable extends Server> elements) {
this.servers = ImmutableList.builder();
return addAllServers(elements);
}
/**
* Adds elements to {@link AbstractOpenApi#getServers() servers} list.
* @param elements An iterable of servers elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addAllServers(Iterable extends Server> elements) {
this.servers.addAll(elements);
optBits |= OPT_BIT_SERVERS;
return this;
}
/**
* Initializes the optional value {@link AbstractOpenApi#getComponents() components} to components.
* @param components The value for components, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
@CanIgnoreReturnValue
public final Builder setComponents(@Nullable Components components) {
this.components = components;
return this;
}
/**
* Initializes the optional value {@link AbstractOpenApi#getComponents() components} to components.
* @param components The value for components
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder setComponents(Optional extends Components> components) {
this.components = components.orElse(null);
return this;
}
/**
* Adds one element to {@link AbstractOpenApi#getSecurity() security} list.
* @param element A security element
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addSecurity(SecurityRequirement element) {
this.security.add(element);
return this;
}
/**
* Adds elements to {@link AbstractOpenApi#getSecurity() security} list.
* @param elements An array of security elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addSecurity(SecurityRequirement... elements) {
this.security.add(elements);
return this;
}
/**
* Sets or replaces all elements for {@link AbstractOpenApi#getSecurity() security} list.
* @param elements An iterable of security elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder setSecurity(Iterable extends SecurityRequirement> elements) {
this.security = ImmutableList.builder();
return addAllSecurity(elements);
}
/**
* Adds elements to {@link AbstractOpenApi#getSecurity() security} list.
* @param elements An iterable of security elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addAllSecurity(Iterable extends SecurityRequirement> elements) {
this.security.addAll(elements);
return this;
}
/**
* Adds one element to {@link AbstractOpenApi#getTags() tags} list.
* @param element A tags element
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addTags(Tag element) {
this.tags.add(element);
return this;
}
/**
* Adds elements to {@link AbstractOpenApi#getTags() tags} list.
* @param elements An array of tags elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addTags(Tag... elements) {
this.tags.add(elements);
return this;
}
/**
* Sets or replaces all elements for {@link AbstractOpenApi#getTags() tags} list.
* @param elements An iterable of tags elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder setTags(Iterable extends Tag> elements) {
this.tags = ImmutableList.builder();
return addAllTags(elements);
}
/**
* Adds elements to {@link AbstractOpenApi#getTags() tags} list.
* @param elements An iterable of tags elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addAllTags(Iterable extends Tag> elements) {
this.tags.addAll(elements);
return this;
}
/**
* Initializes the optional value {@link AbstractOpenApi#getExternalDocs() externalDocs} to externalDocs.
* @param externalDocs The value for externalDocs, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
@CanIgnoreReturnValue
public final Builder setExternalDocs(@Nullable ExternalDocumentation externalDocs) {
this.externalDocs = externalDocs;
return this;
}
/**
* Initializes the optional value {@link AbstractOpenApi#getExternalDocs() externalDocs} to externalDocs.
* @param externalDocs The value for externalDocs
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder setExternalDocs(Optional extends ExternalDocumentation> externalDocs) {
this.externalDocs = externalDocs.orElse(null);
return this;
}
/**
* Initializes the value for the {@link AbstractOpenApi#getPaths() paths} attribute.
* If not set, this attribute will have a default value as returned by the initializer of {@link AbstractOpenApi#getPaths() paths}.
* @param paths The value for paths
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder setPaths(Paths paths) {
this.paths = Objects.requireNonNull(paths, "paths");
return this;
}
/**
* Put one entry to the {@link AbstractOpenApi#getExtensions() extensions} map.
* @param key The key in the extensions map
* @param value The associated value in the extensions map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonAnySetter
public final Builder putExtensions(String key, Object value) {
this.extensions.put(key, value);
return this;
}
/**
* Put one entry to the {@link AbstractOpenApi#getExtensions() extensions} map. Nulls are not permitted
* @param entry The key and value entry
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putExtensions(Map.Entry entry) {
this.extensions.put(entry);
return this;
}
/**
* Sets or replaces all mappings from the specified map as entries for the {@link AbstractOpenApi#getExtensions() extensions} map. Nulls are not permitted
* @param extensions The entries that will be added to the extensions map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder setExtensions(Map extensions) {
this.extensions = ImmutableMap.builder();
return putAllExtensions(extensions);
}
/**
* Put all mappings from the specified map as entries to {@link AbstractOpenApi#getExtensions() extensions} map. Nulls are not permitted
* @param extensions The entries that will be added to the extensions map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putAllExtensions(Map extensions) {
this.extensions.putAll(extensions);
return this;
}
/**
* Builds a new {@link OpenApi OpenApi}.
* @return An immutable instance of OpenApi
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public OpenApi build() {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
return OpenApi.validate(new OpenApi(this));
}
private boolean serversIsSet() {
return (optBits & OPT_BIT_SERVERS) != 0;
}
private String formatRequiredAttributesMessage() {
List attributes = Lists.newArrayList();
if ((initBits & INIT_BIT_OPEN_API) != 0) attributes.add("openApi");
if ((initBits & INIT_BIT_INFO) != 0) attributes.add("info");
return "Cannot build OpenApi, some of required attributes are not set " + attributes;
}
}
}