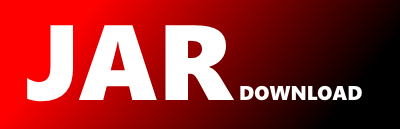
io.github.emm035.openapi.immutables.v3.Operation Maven / Gradle / Ivy
package io.github.emm035.openapi.immutables.v3;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonAutoDetect;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.google.common.base.MoreObjects;
import com.google.common.collect.ImmutableList;
import com.google.common.collect.ImmutableMap;
import com.google.common.collect.Lists;
import com.google.errorprone.annotations.CanIgnoreReturnValue;
import io.github.emm035.openapi.immutables.v3.metadata.ExternalDocumentation;
import io.github.emm035.openapi.immutables.v3.parameters.Parameter;
import io.github.emm035.openapi.immutables.v3.references.Referenceable;
import io.github.emm035.openapi.immutables.v3.requests.RequestBody;
import io.github.emm035.openapi.immutables.v3.responses.Responses;
import io.github.emm035.openapi.immutables.v3.security.SecurityRequirement;
import io.github.emm035.openapi.immutables.v3.servers.Server;
import io.github.emm035.openapi.immutables.v3.shared.Deprecatable;
import io.github.emm035.openapi.immutables.v3.shared.Describable;
import io.github.emm035.openapi.immutables.v3.shared.Extensible;
import io.github.emm035.openapi.immutables.v3.shared.Summarizable;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.CheckReturnValue;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
/**
* Immutable implementation of {@link AbstractOperation}.
*
* Use the builder to create immutable instances:
* {@code Operation.builder()}.
*/
@SuppressWarnings({"all"})
@ParametersAreNonnullByDefault
@Immutable
@CheckReturnValue
public final class Operation extends AbstractOperation {
private final Responses responses;
private final @Nullable Referenceable requestBody;
private final @Nullable ExternalDocumentation externalDocs;
private final @Nullable String operationId;
private final ImmutableList tags;
private final ImmutableList> parameters;
private final ImmutableList servers;
private final ImmutableList security;
private final ImmutableMap callbacks;
private final @Nullable String summary;
private final @Nullable String description;
private final ImmutableMap extensions;
private final @Nullable Boolean deprecated;
private Operation(
Responses responses,
@Nullable Referenceable requestBody,
@Nullable ExternalDocumentation externalDocs,
@Nullable String operationId,
ImmutableList tags,
ImmutableList> parameters,
ImmutableList servers,
ImmutableList security,
ImmutableMap callbacks,
@Nullable String summary,
@Nullable String description,
ImmutableMap extensions,
@Nullable Boolean deprecated) {
this.responses = responses;
this.requestBody = requestBody;
this.externalDocs = externalDocs;
this.operationId = operationId;
this.tags = tags;
this.parameters = parameters;
this.servers = servers;
this.security = security;
this.callbacks = callbacks;
this.summary = summary;
this.description = description;
this.extensions = extensions;
this.deprecated = deprecated;
}
/**
* @return The value of the {@code responses} attribute
*/
@JsonProperty("responses")
@Override
public Responses getResponses() {
return responses;
}
/**
* @return The value of the {@code requestBody} attribute
*/
@JsonProperty("requestBody")
@Override
public Optional> getRequestBody() {
return Optional.ofNullable(requestBody);
}
/**
* @return The value of the {@code externalDocs} attribute
*/
@JsonProperty("externalDocs")
@Override
public Optional getExternalDocs() {
return Optional.ofNullable(externalDocs);
}
/**
* @return The value of the {@code operationId} attribute
*/
@JsonProperty("operationId")
@Override
public Optional getOperationId() {
return Optional.ofNullable(operationId);
}
/**
* @return The value of the {@code tags} attribute
*/
@JsonProperty("tags")
@Override
public ImmutableList getTags() {
return tags;
}
/**
* @return The value of the {@code parameters} attribute
*/
@JsonProperty("parameters")
@Override
public ImmutableList> getParameters() {
return parameters;
}
/**
* @return The value of the {@code servers} attribute
*/
@JsonProperty("servers")
@Override
public ImmutableList getServers() {
return servers;
}
/**
* @return The value of the {@code security} attribute
*/
@JsonProperty("security")
@Override
public ImmutableList getSecurity() {
return security;
}
/**
* @return The value of the {@code callbacks} attribute
*/
@JsonProperty("callbacks")
@Override
public ImmutableMap getCallbacks() {
return callbacks;
}
/**
* @return The value of the {@code summary} attribute
*/
@JsonProperty("summary")
@Override
public Optional getSummary() {
return Optional.ofNullable(summary);
}
/**
* @return The value of the {@code description} attribute
*/
@JsonProperty("description")
@Override
public Optional getDescription() {
return Optional.ofNullable(description);
}
/**
* @return The value of the {@code extensions} attribute
*/
@JsonProperty("extensions")
@JsonAnyGetter
@Override
public ImmutableMap getExtensions() {
return extensions;
}
/**
* @return The value of the {@code deprecated} attribute
*/
@JsonProperty("deprecated")
@Override
public Optional getDeprecated() {
return Optional.ofNullable(deprecated);
}
/**
* Copy the current immutable object by setting a value for the {@link AbstractOperation#getResponses() responses} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for responses
* @return A modified copy of the {@code this} object
*/
public final Operation withResponses(Responses value) {
if (this.responses == value) return this;
Responses newValue = Objects.requireNonNull(value, "responses");
return validate(new Operation(
newValue,
this.requestBody,
this.externalDocs,
this.operationId,
this.tags,
this.parameters,
this.servers,
this.security,
this.callbacks,
this.summary,
this.description,
this.extensions,
this.deprecated));
}
/**
* Copy the current immutable object by setting a present value for the optional {@link AbstractOperation#getRequestBody() requestBody} attribute.
* @param value The value for requestBody, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final Operation withRequestBody(@Nullable Referenceable value) {
@Nullable Referenceable newValue = value;
if (this.requestBody == newValue) return this;
return validate(new Operation(
this.responses,
newValue,
this.externalDocs,
this.operationId,
this.tags,
this.parameters,
this.servers,
this.security,
this.callbacks,
this.summary,
this.description,
this.extensions,
this.deprecated));
}
/**
* Copy the current immutable object by setting an optional value for the {@link AbstractOperation#getRequestBody() requestBody} attribute.
* A shallow reference equality check is used on unboxed optional value to prevent copying of the same value by returning {@code this}.
* @param optional A value for requestBody
* @return A modified copy of {@code this} object
*/
public final Operation withRequestBody(Optional extends Referenceable> optional) {
@Nullable Referenceable value = optional.orElse(null);
if (this.requestBody == value) return this;
return validate(new Operation(
this.responses,
value,
this.externalDocs,
this.operationId,
this.tags,
this.parameters,
this.servers,
this.security,
this.callbacks,
this.summary,
this.description,
this.extensions,
this.deprecated));
}
/**
* Copy the current immutable object by setting a present value for the optional {@link AbstractOperation#getExternalDocs() externalDocs} attribute.
* @param value The value for externalDocs, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final Operation withExternalDocs(@Nullable ExternalDocumentation value) {
@Nullable ExternalDocumentation newValue = value;
if (this.externalDocs == newValue) return this;
return validate(new Operation(
this.responses,
this.requestBody,
newValue,
this.operationId,
this.tags,
this.parameters,
this.servers,
this.security,
this.callbacks,
this.summary,
this.description,
this.extensions,
this.deprecated));
}
/**
* Copy the current immutable object by setting an optional value for the {@link AbstractOperation#getExternalDocs() externalDocs} attribute.
* A shallow reference equality check is used on unboxed optional value to prevent copying of the same value by returning {@code this}.
* @param optional A value for externalDocs
* @return A modified copy of {@code this} object
*/
public final Operation withExternalDocs(Optional extends ExternalDocumentation> optional) {
@Nullable ExternalDocumentation value = optional.orElse(null);
if (this.externalDocs == value) return this;
return validate(new Operation(
this.responses,
this.requestBody,
value,
this.operationId,
this.tags,
this.parameters,
this.servers,
this.security,
this.callbacks,
this.summary,
this.description,
this.extensions,
this.deprecated));
}
/**
* Copy the current immutable object by setting a present value for the optional {@link AbstractOperation#getOperationId() operationId} attribute.
* @param value The value for operationId, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final Operation withOperationId(@Nullable String value) {
@Nullable String newValue = value;
if (Objects.equals(this.operationId, newValue)) return this;
return validate(new Operation(
this.responses,
this.requestBody,
this.externalDocs,
newValue,
this.tags,
this.parameters,
this.servers,
this.security,
this.callbacks,
this.summary,
this.description,
this.extensions,
this.deprecated));
}
/**
* Copy the current immutable object by setting an optional value for the {@link AbstractOperation#getOperationId() operationId} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for operationId
* @return A modified copy of {@code this} object
*/
public final Operation withOperationId(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.operationId, value)) return this;
return validate(new Operation(
this.responses,
this.requestBody,
this.externalDocs,
value,
this.tags,
this.parameters,
this.servers,
this.security,
this.callbacks,
this.summary,
this.description,
this.extensions,
this.deprecated));
}
/**
* Copy the current immutable object with elements that replace the content of {@link AbstractOperation#getTags() tags}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final Operation withTags(String... elements) {
ImmutableList newValue = ImmutableList.copyOf(elements);
return validate(new Operation(
this.responses,
this.requestBody,
this.externalDocs,
this.operationId,
newValue,
this.parameters,
this.servers,
this.security,
this.callbacks,
this.summary,
this.description,
this.extensions,
this.deprecated));
}
/**
* Copy the current immutable object with elements that replace the content of {@link AbstractOperation#getTags() tags}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of tags elements to set
* @return A modified copy of {@code this} object
*/
public final Operation withTags(Iterable elements) {
if (this.tags == elements) return this;
ImmutableList newValue = ImmutableList.copyOf(elements);
return validate(new Operation(
this.responses,
this.requestBody,
this.externalDocs,
this.operationId,
newValue,
this.parameters,
this.servers,
this.security,
this.callbacks,
this.summary,
this.description,
this.extensions,
this.deprecated));
}
/**
* Copy the current immutable object with elements that replace the content of {@link AbstractOperation#getParameters() parameters}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
@SafeVarargs
public final Operation withParameters(Referenceable... elements) {
ImmutableList> newValue = ImmutableList.copyOf(elements);
return validate(new Operation(
this.responses,
this.requestBody,
this.externalDocs,
this.operationId,
this.tags,
newValue,
this.servers,
this.security,
this.callbacks,
this.summary,
this.description,
this.extensions,
this.deprecated));
}
/**
* Copy the current immutable object with elements that replace the content of {@link AbstractOperation#getParameters() parameters}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of parameters elements to set
* @return A modified copy of {@code this} object
*/
public final Operation withParameters(Iterable extends Referenceable> elements) {
if (this.parameters == elements) return this;
ImmutableList> newValue = ImmutableList.copyOf(elements);
return validate(new Operation(
this.responses,
this.requestBody,
this.externalDocs,
this.operationId,
this.tags,
newValue,
this.servers,
this.security,
this.callbacks,
this.summary,
this.description,
this.extensions,
this.deprecated));
}
/**
* Copy the current immutable object with elements that replace the content of {@link AbstractOperation#getServers() servers}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final Operation withServers(Server... elements) {
ImmutableList newValue = ImmutableList.copyOf(elements);
return validate(new Operation(
this.responses,
this.requestBody,
this.externalDocs,
this.operationId,
this.tags,
this.parameters,
newValue,
this.security,
this.callbacks,
this.summary,
this.description,
this.extensions,
this.deprecated));
}
/**
* Copy the current immutable object with elements that replace the content of {@link AbstractOperation#getServers() servers}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of servers elements to set
* @return A modified copy of {@code this} object
*/
public final Operation withServers(Iterable extends Server> elements) {
if (this.servers == elements) return this;
ImmutableList newValue = ImmutableList.copyOf(elements);
return validate(new Operation(
this.responses,
this.requestBody,
this.externalDocs,
this.operationId,
this.tags,
this.parameters,
newValue,
this.security,
this.callbacks,
this.summary,
this.description,
this.extensions,
this.deprecated));
}
/**
* Copy the current immutable object with elements that replace the content of {@link AbstractOperation#getSecurity() security}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final Operation withSecurity(SecurityRequirement... elements) {
ImmutableList newValue = ImmutableList.copyOf(elements);
return validate(new Operation(
this.responses,
this.requestBody,
this.externalDocs,
this.operationId,
this.tags,
this.parameters,
this.servers,
newValue,
this.callbacks,
this.summary,
this.description,
this.extensions,
this.deprecated));
}
/**
* Copy the current immutable object with elements that replace the content of {@link AbstractOperation#getSecurity() security}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of security elements to set
* @return A modified copy of {@code this} object
*/
public final Operation withSecurity(Iterable extends SecurityRequirement> elements) {
if (this.security == elements) return this;
ImmutableList newValue = ImmutableList.copyOf(elements);
return validate(new Operation(
this.responses,
this.requestBody,
this.externalDocs,
this.operationId,
this.tags,
this.parameters,
this.servers,
newValue,
this.callbacks,
this.summary,
this.description,
this.extensions,
this.deprecated));
}
/**
* Copy the current immutable object by replacing the {@link AbstractOperation#getCallbacks() callbacks} map with the specified map.
* Nulls are not permitted as keys or values.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param entries The entries to be added to the callbacks map
* @return A modified copy of {@code this} object
*/
public final Operation withCallbacks(Map entries) {
if (this.callbacks == entries) return this;
ImmutableMap newValue = ImmutableMap.copyOf(entries);
return validate(new Operation(
this.responses,
this.requestBody,
this.externalDocs,
this.operationId,
this.tags,
this.parameters,
this.servers,
this.security,
newValue,
this.summary,
this.description,
this.extensions,
this.deprecated));
}
/**
* Copy the current immutable object by setting a present value for the optional {@link AbstractOperation#getSummary() summary} attribute.
* @param value The value for summary, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final Operation withSummary(@Nullable String value) {
@Nullable String newValue = value;
if (Objects.equals(this.summary, newValue)) return this;
return validate(new Operation(
this.responses,
this.requestBody,
this.externalDocs,
this.operationId,
this.tags,
this.parameters,
this.servers,
this.security,
this.callbacks,
newValue,
this.description,
this.extensions,
this.deprecated));
}
/**
* Copy the current immutable object by setting an optional value for the {@link AbstractOperation#getSummary() summary} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for summary
* @return A modified copy of {@code this} object
*/
public final Operation withSummary(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.summary, value)) return this;
return validate(new Operation(
this.responses,
this.requestBody,
this.externalDocs,
this.operationId,
this.tags,
this.parameters,
this.servers,
this.security,
this.callbacks,
value,
this.description,
this.extensions,
this.deprecated));
}
/**
* Copy the current immutable object by setting a present value for the optional {@link AbstractOperation#getDescription() description} attribute.
* @param value The value for description, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final Operation withDescription(@Nullable String value) {
@Nullable String newValue = value;
if (Objects.equals(this.description, newValue)) return this;
return validate(new Operation(
this.responses,
this.requestBody,
this.externalDocs,
this.operationId,
this.tags,
this.parameters,
this.servers,
this.security,
this.callbacks,
this.summary,
newValue,
this.extensions,
this.deprecated));
}
/**
* Copy the current immutable object by setting an optional value for the {@link AbstractOperation#getDescription() description} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for description
* @return A modified copy of {@code this} object
*/
public final Operation withDescription(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.description, value)) return this;
return validate(new Operation(
this.responses,
this.requestBody,
this.externalDocs,
this.operationId,
this.tags,
this.parameters,
this.servers,
this.security,
this.callbacks,
this.summary,
value,
this.extensions,
this.deprecated));
}
/**
* Copy the current immutable object by replacing the {@link AbstractOperation#getExtensions() extensions} map with the specified map.
* Nulls are not permitted as keys or values.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param entries The entries to be added to the extensions map
* @return A modified copy of {@code this} object
*/
public final Operation withExtensions(Map entries) {
if (this.extensions == entries) return this;
ImmutableMap newValue = ImmutableMap.copyOf(entries);
return validate(new Operation(
this.responses,
this.requestBody,
this.externalDocs,
this.operationId,
this.tags,
this.parameters,
this.servers,
this.security,
this.callbacks,
this.summary,
this.description,
newValue,
this.deprecated));
}
/**
* Copy the current immutable object by setting a present value for the optional {@link AbstractOperation#getDeprecated() deprecated} attribute.
* @param value The value for deprecated, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final Operation withDeprecated(@Nullable Boolean value) {
@Nullable Boolean newValue = value;
if (Objects.equals(this.deprecated, newValue)) return this;
return validate(new Operation(
this.responses,
this.requestBody,
this.externalDocs,
this.operationId,
this.tags,
this.parameters,
this.servers,
this.security,
this.callbacks,
this.summary,
this.description,
this.extensions,
newValue));
}
/**
* Copy the current immutable object by setting an optional value for the {@link AbstractOperation#getDeprecated() deprecated} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for deprecated
* @return A modified copy of {@code this} object
*/
public final Operation withDeprecated(Optional optional) {
@Nullable Boolean value = optional.orElse(null);
if (Objects.equals(this.deprecated, value)) return this;
return validate(new Operation(
this.responses,
this.requestBody,
this.externalDocs,
this.operationId,
this.tags,
this.parameters,
this.servers,
this.security,
this.callbacks,
this.summary,
this.description,
this.extensions,
value));
}
/**
* This instance is equal to all instances of {@code Operation} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof Operation
&& equalTo((Operation) another);
}
private boolean equalTo(Operation another) {
return responses.equals(another.responses)
&& Objects.equals(requestBody, another.requestBody)
&& Objects.equals(externalDocs, another.externalDocs)
&& Objects.equals(operationId, another.operationId)
&& tags.equals(another.tags)
&& parameters.equals(another.parameters)
&& servers.equals(another.servers)
&& security.equals(another.security)
&& callbacks.equals(another.callbacks)
&& Objects.equals(summary, another.summary)
&& Objects.equals(description, another.description)
&& extensions.equals(another.extensions)
&& Objects.equals(deprecated, another.deprecated);
}
/**
* Computes a hash code from attributes: {@code responses}, {@code requestBody}, {@code externalDocs}, {@code operationId}, {@code tags}, {@code parameters}, {@code servers}, {@code security}, {@code callbacks}, {@code summary}, {@code description}, {@code extensions}, {@code deprecated}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + responses.hashCode();
h += (h << 5) + Objects.hashCode(requestBody);
h += (h << 5) + Objects.hashCode(externalDocs);
h += (h << 5) + Objects.hashCode(operationId);
h += (h << 5) + tags.hashCode();
h += (h << 5) + parameters.hashCode();
h += (h << 5) + servers.hashCode();
h += (h << 5) + security.hashCode();
h += (h << 5) + callbacks.hashCode();
h += (h << 5) + Objects.hashCode(summary);
h += (h << 5) + Objects.hashCode(description);
h += (h << 5) + extensions.hashCode();
h += (h << 5) + Objects.hashCode(deprecated);
return h;
}
/**
* Prints the immutable value {@code Operation} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return MoreObjects.toStringHelper("Operation")
.omitNullValues()
.add("responses", responses)
.add("requestBody", requestBody)
.add("externalDocs", externalDocs)
.add("operationId", operationId)
.add("tags", tags)
.add("parameters", parameters)
.add("servers", servers)
.add("security", security)
.add("callbacks", callbacks)
.add("summary", summary)
.add("description", description)
.add("extensions", extensions)
.add("deprecated", deprecated)
.toString();
}
/**
* Utility type used to correctly read immutable object from JSON representation.
* @deprecated Do not use this type directly, it exists only for the Jackson-binding infrastructure
*/
@Deprecated
@JsonAutoDetect(fieldVisibility = JsonAutoDetect.Visibility.NONE)
static final class Json extends AbstractOperation {
@Nullable Responses responses;
@Nullable Optional> requestBody = Optional.empty();
@Nullable Optional externalDocs = Optional.empty();
@Nullable Optional operationId = Optional.empty();
@Nullable List tags = ImmutableList.of();
@Nullable List> parameters = ImmutableList.of();
@Nullable List servers = ImmutableList.of();
@Nullable List security = ImmutableList.of();
@Nullable Map callbacks = ImmutableMap.of();
@Nullable Optional summary = Optional.empty();
@Nullable Optional description = Optional.empty();
@Nullable Optional deprecated = Optional.empty();
final Map extensions = new HashMap();
@JsonProperty("responses")
public void setResponses(Responses responses) {
this.responses = responses;
}
@JsonProperty("requestBody")
public void setRequestBody(Optional> requestBody) {
this.requestBody = requestBody;
}
@JsonProperty("externalDocs")
public void setExternalDocs(Optional externalDocs) {
this.externalDocs = externalDocs;
}
@JsonProperty("operationId")
public void setOperationId(Optional operationId) {
this.operationId = operationId;
}
@JsonProperty("tags")
public void setTags(List tags) {
this.tags = tags;
}
@JsonProperty("parameters")
public void setParameters(List> parameters) {
this.parameters = parameters;
}
@JsonProperty("servers")
public void setServers(List servers) {
this.servers = servers;
}
@JsonProperty("security")
public void setSecurity(List security) {
this.security = security;
}
@JsonProperty("callbacks")
public void setCallbacks(Map callbacks) {
this.callbacks = callbacks;
}
@JsonProperty("summary")
public void setSummary(Optional summary) {
this.summary = summary;
}
@JsonProperty("description")
public void setDescription(Optional description) {
this.description = description;
}
@JsonProperty("deprecated")
public void setDeprecated(Optional deprecated) {
this.deprecated = deprecated;
}
@JsonAnySetter
public void setExtensions(String key, Object value) {
this.extensions.put(key, value);
}
@Override
public Responses getResponses() { throw new UnsupportedOperationException(); }
@Override
public Optional> getRequestBody() { throw new UnsupportedOperationException(); }
@Override
public Optional getExternalDocs() { throw new UnsupportedOperationException(); }
@Override
public Optional getOperationId() { throw new UnsupportedOperationException(); }
@Override
public List getTags() { throw new UnsupportedOperationException(); }
@Override
public List> getParameters() { throw new UnsupportedOperationException(); }
@Override
public List getServers() { throw new UnsupportedOperationException(); }
@Override
public List getSecurity() { throw new UnsupportedOperationException(); }
@Override
public Map getCallbacks() { throw new UnsupportedOperationException(); }
@Override
public Optional getSummary() { throw new UnsupportedOperationException(); }
@Override
public Optional getDescription() { throw new UnsupportedOperationException(); }
@Override
public Map getExtensions() { throw new UnsupportedOperationException(); }
@Override
public Optional getDeprecated() { throw new UnsupportedOperationException(); }
}
/**
* @param json A JSON-bindable data structure
* @return An immutable value type
* @deprecated Do not use this method directly, it exists only for the Jackson-binding infrastructure
*/
@Deprecated
@JsonCreator(mode = JsonCreator.Mode.DELEGATING)
static Operation fromJson(Json json) {
Operation.Builder builder = Operation.builder();
if (json.responses != null) {
builder.setResponses(json.responses);
}
if (json.requestBody != null) {
builder.setRequestBody(json.requestBody);
}
if (json.externalDocs != null) {
builder.setExternalDocs(json.externalDocs);
}
if (json.operationId != null) {
builder.setOperationId(json.operationId);
}
if (json.tags != null) {
builder.addAllTags(json.tags);
}
if (json.parameters != null) {
builder.addAllParameters(json.parameters);
}
if (json.servers != null) {
builder.addAllServers(json.servers);
}
if (json.security != null) {
builder.addAllSecurity(json.security);
}
if (json.callbacks != null) {
builder.putAllCallbacks(json.callbacks);
}
if (json.summary != null) {
builder.setSummary(json.summary);
}
if (json.description != null) {
builder.setDescription(json.description);
}
if (json.extensions != null) {
builder.putAllExtensions(json.extensions);
}
if (json.deprecated != null) {
builder.setDeprecated(json.deprecated);
}
return builder.build();
}
private static Operation validate(Operation instance) {
instance = (Operation) instance.normalizeExtensions();
return instance;
}
/**
* Creates an immutable copy of a {@link AbstractOperation} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable Operation instance
*/
public static Operation copyOf(AbstractOperation instance) {
if (instance instanceof Operation) {
return (Operation) instance;
}
return Operation.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link Operation Operation}.
* @return A new Operation builder
*/
public static Operation.Builder builder() {
return new Operation.Builder();
}
/**
* Builds instances of type {@link Operation Operation}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@NotThreadSafe
public static final class Builder {
private static final long INIT_BIT_RESPONSES = 0x1L;
private long initBits = 0x1L;
private @Nullable Responses responses;
private @Nullable Referenceable requestBody;
private @Nullable ExternalDocumentation externalDocs;
private @Nullable String operationId;
private ImmutableList.Builder tags = ImmutableList.builder();
private ImmutableList.Builder> parameters = ImmutableList.builder();
private ImmutableList.Builder servers = ImmutableList.builder();
private ImmutableList.Builder security = ImmutableList.builder();
private ImmutableMap.Builder callbacks = ImmutableMap.builder();
private @Nullable String summary;
private @Nullable String description;
private ImmutableMap.Builder extensions = ImmutableMap.builder();
private @Nullable Boolean deprecated;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code io.github.emm035.openapi.immutables.v3.shared.Summarizable} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder from(Summarizable instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return this;
}
/**
* Fill a builder with attribute values from the provided {@code io.github.emm035.openapi.immutables.v3.AbstractOperation} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder from(AbstractOperation instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return this;
}
/**
* Fill a builder with attribute values from the provided {@code io.github.emm035.openapi.immutables.v3.shared.Deprecatable} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder from(Deprecatable instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return this;
}
/**
* Fill a builder with attribute values from the provided {@code io.github.emm035.openapi.immutables.v3.shared.Extensible} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder from(Extensible instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return this;
}
/**
* Fill a builder with attribute values from the provided {@code io.github.emm035.openapi.immutables.v3.shared.Describable} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder from(Describable instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return this;
}
private void from(Object object) {
if (object instanceof Summarizable) {
Summarizable instance = (Summarizable) object;
Optional summaryOptional = instance.getSummary();
if (summaryOptional.isPresent()) {
setSummary(summaryOptional);
}
}
if (object instanceof AbstractOperation) {
AbstractOperation instance = (AbstractOperation) object;
addAllSecurity(instance.getSecurity());
addAllServers(instance.getServers());
Optional> requestBodyOptional = instance.getRequestBody();
if (requestBodyOptional.isPresent()) {
setRequestBody(requestBodyOptional);
}
putAllCallbacks(instance.getCallbacks());
setResponses(instance.getResponses());
Optional operationIdOptional = instance.getOperationId();
if (operationIdOptional.isPresent()) {
setOperationId(operationIdOptional);
}
Optional externalDocsOptional = instance.getExternalDocs();
if (externalDocsOptional.isPresent()) {
setExternalDocs(externalDocsOptional);
}
addAllParameters(instance.getParameters());
addAllTags(instance.getTags());
}
if (object instanceof Deprecatable) {
Deprecatable instance = (Deprecatable) object;
Optional deprecatedOptional = instance.getDeprecated();
if (deprecatedOptional.isPresent()) {
setDeprecated(deprecatedOptional);
}
}
if (object instanceof Extensible) {
Extensible instance = (Extensible) object;
putAllExtensions(instance.getExtensions());
}
if (object instanceof Describable) {
Describable instance = (Describable) object;
Optional descriptionOptional = instance.getDescription();
if (descriptionOptional.isPresent()) {
setDescription(descriptionOptional);
}
}
}
/**
* Initializes the value for the {@link AbstractOperation#getResponses() responses} attribute.
* @param responses The value for responses
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder setResponses(Responses responses) {
this.responses = Objects.requireNonNull(responses, "responses");
initBits &= ~INIT_BIT_RESPONSES;
return this;
}
/**
* Initializes the optional value {@link AbstractOperation#getRequestBody() requestBody} to requestBody.
* @param requestBody The value for requestBody, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
@CanIgnoreReturnValue
public final Builder setRequestBody(@Nullable Referenceable requestBody) {
this.requestBody = requestBody;
return this;
}
/**
* Initializes the optional value {@link AbstractOperation#getRequestBody() requestBody} to requestBody.
* @param requestBody The value for requestBody
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder setRequestBody(Optional extends Referenceable> requestBody) {
this.requestBody = requestBody.orElse(null);
return this;
}
/**
* Initializes the optional value {@link AbstractOperation#getExternalDocs() externalDocs} to externalDocs.
* @param externalDocs The value for externalDocs, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
@CanIgnoreReturnValue
public final Builder setExternalDocs(@Nullable ExternalDocumentation externalDocs) {
this.externalDocs = externalDocs;
return this;
}
/**
* Initializes the optional value {@link AbstractOperation#getExternalDocs() externalDocs} to externalDocs.
* @param externalDocs The value for externalDocs
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder setExternalDocs(Optional extends ExternalDocumentation> externalDocs) {
this.externalDocs = externalDocs.orElse(null);
return this;
}
/**
* Initializes the optional value {@link AbstractOperation#getOperationId() operationId} to operationId.
* @param operationId The value for operationId, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
@CanIgnoreReturnValue
public final Builder setOperationId(@Nullable String operationId) {
this.operationId = operationId;
return this;
}
/**
* Initializes the optional value {@link AbstractOperation#getOperationId() operationId} to operationId.
* @param operationId The value for operationId
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder setOperationId(Optional operationId) {
this.operationId = operationId.orElse(null);
return this;
}
/**
* Adds one element to {@link AbstractOperation#getTags() tags} list.
* @param element A tags element
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addTags(String element) {
this.tags.add(element);
return this;
}
/**
* Adds elements to {@link AbstractOperation#getTags() tags} list.
* @param elements An array of tags elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addTags(String... elements) {
this.tags.add(elements);
return this;
}
/**
* Sets or replaces all elements for {@link AbstractOperation#getTags() tags} list.
* @param elements An iterable of tags elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder setTags(Iterable elements) {
this.tags = ImmutableList.builder();
return addAllTags(elements);
}
/**
* Adds elements to {@link AbstractOperation#getTags() tags} list.
* @param elements An iterable of tags elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addAllTags(Iterable elements) {
this.tags.addAll(elements);
return this;
}
/**
* Adds one element to {@link AbstractOperation#getParameters() parameters} list.
* @param element A parameters element
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addParameters(Referenceable element) {
this.parameters.add(element);
return this;
}
/**
* Adds elements to {@link AbstractOperation#getParameters() parameters} list.
* @param elements An array of parameters elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@SafeVarargs
public final Builder addParameters(Referenceable... elements) {
this.parameters.add(elements);
return this;
}
/**
* Sets or replaces all elements for {@link AbstractOperation#getParameters() parameters} list.
* @param elements An iterable of parameters elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder setParameters(Iterable extends Referenceable> elements) {
this.parameters = ImmutableList.builder();
return addAllParameters(elements);
}
/**
* Adds elements to {@link AbstractOperation#getParameters() parameters} list.
* @param elements An iterable of parameters elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addAllParameters(Iterable extends Referenceable> elements) {
this.parameters.addAll(elements);
return this;
}
/**
* Adds one element to {@link AbstractOperation#getServers() servers} list.
* @param element A servers element
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addServers(Server element) {
this.servers.add(element);
return this;
}
/**
* Adds elements to {@link AbstractOperation#getServers() servers} list.
* @param elements An array of servers elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addServers(Server... elements) {
this.servers.add(elements);
return this;
}
/**
* Sets or replaces all elements for {@link AbstractOperation#getServers() servers} list.
* @param elements An iterable of servers elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder setServers(Iterable extends Server> elements) {
this.servers = ImmutableList.builder();
return addAllServers(elements);
}
/**
* Adds elements to {@link AbstractOperation#getServers() servers} list.
* @param elements An iterable of servers elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addAllServers(Iterable extends Server> elements) {
this.servers.addAll(elements);
return this;
}
/**
* Adds one element to {@link AbstractOperation#getSecurity() security} list.
* @param element A security element
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addSecurity(SecurityRequirement element) {
this.security.add(element);
return this;
}
/**
* Adds elements to {@link AbstractOperation#getSecurity() security} list.
* @param elements An array of security elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addSecurity(SecurityRequirement... elements) {
this.security.add(elements);
return this;
}
/**
* Sets or replaces all elements for {@link AbstractOperation#getSecurity() security} list.
* @param elements An iterable of security elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder setSecurity(Iterable extends SecurityRequirement> elements) {
this.security = ImmutableList.builder();
return addAllSecurity(elements);
}
/**
* Adds elements to {@link AbstractOperation#getSecurity() security} list.
* @param elements An iterable of security elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addAllSecurity(Iterable extends SecurityRequirement> elements) {
this.security.addAll(elements);
return this;
}
/**
* Put one entry to the {@link AbstractOperation#getCallbacks() callbacks} map.
* @param key The key in the callbacks map
* @param value The associated value in the callbacks map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putCallbacks(String key, Object value) {
this.callbacks.put(key, value);
return this;
}
/**
* Put one entry to the {@link AbstractOperation#getCallbacks() callbacks} map. Nulls are not permitted
* @param entry The key and value entry
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putCallbacks(Map.Entry entry) {
this.callbacks.put(entry);
return this;
}
/**
* Sets or replaces all mappings from the specified map as entries for the {@link AbstractOperation#getCallbacks() callbacks} map. Nulls are not permitted
* @param callbacks The entries that will be added to the callbacks map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder setCallbacks(Map callbacks) {
this.callbacks = ImmutableMap.builder();
return putAllCallbacks(callbacks);
}
/**
* Put all mappings from the specified map as entries to {@link AbstractOperation#getCallbacks() callbacks} map. Nulls are not permitted
* @param callbacks The entries that will be added to the callbacks map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putAllCallbacks(Map callbacks) {
this.callbacks.putAll(callbacks);
return this;
}
/**
* Initializes the optional value {@link AbstractOperation#getSummary() summary} to summary.
* @param summary The value for summary, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
@CanIgnoreReturnValue
public final Builder setSummary(@Nullable String summary) {
this.summary = summary;
return this;
}
/**
* Initializes the optional value {@link AbstractOperation#getSummary() summary} to summary.
* @param summary The value for summary
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder setSummary(Optional summary) {
this.summary = summary.orElse(null);
return this;
}
/**
* Initializes the optional value {@link AbstractOperation#getDescription() description} to description.
* @param description The value for description, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
@CanIgnoreReturnValue
public final Builder setDescription(@Nullable String description) {
this.description = description;
return this;
}
/**
* Initializes the optional value {@link AbstractOperation#getDescription() description} to description.
* @param description The value for description
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder setDescription(Optional description) {
this.description = description.orElse(null);
return this;
}
/**
* Put one entry to the {@link AbstractOperation#getExtensions() extensions} map.
* @param key The key in the extensions map
* @param value The associated value in the extensions map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonAnySetter
public final Builder putExtensions(String key, Object value) {
this.extensions.put(key, value);
return this;
}
/**
* Put one entry to the {@link AbstractOperation#getExtensions() extensions} map. Nulls are not permitted
* @param entry The key and value entry
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putExtensions(Map.Entry entry) {
this.extensions.put(entry);
return this;
}
/**
* Sets or replaces all mappings from the specified map as entries for the {@link AbstractOperation#getExtensions() extensions} map. Nulls are not permitted
* @param extensions The entries that will be added to the extensions map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder setExtensions(Map extensions) {
this.extensions = ImmutableMap.builder();
return putAllExtensions(extensions);
}
/**
* Put all mappings from the specified map as entries to {@link AbstractOperation#getExtensions() extensions} map. Nulls are not permitted
* @param extensions The entries that will be added to the extensions map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putAllExtensions(Map extensions) {
this.extensions.putAll(extensions);
return this;
}
/**
* Initializes the optional value {@link AbstractOperation#getDeprecated() deprecated} to deprecated.
* @param deprecated The value for deprecated, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
@CanIgnoreReturnValue
public final Builder setDeprecated(@Nullable Boolean deprecated) {
this.deprecated = deprecated;
return this;
}
/**
* Initializes the optional value {@link AbstractOperation#getDeprecated() deprecated} to deprecated.
* @param deprecated The value for deprecated
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder setDeprecated(Optional deprecated) {
this.deprecated = deprecated.orElse(null);
return this;
}
/**
* Builds a new {@link Operation Operation}.
* @return An immutable instance of Operation
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public Operation build() {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
return Operation.validate(new Operation(
responses,
requestBody,
externalDocs,
operationId,
tags.build(),
parameters.build(),
servers.build(),
security.build(),
callbacks.build(),
summary,
description,
extensions.build(),
deprecated));
}
private String formatRequiredAttributesMessage() {
List attributes = Lists.newArrayList();
if ((initBits & INIT_BIT_RESPONSES) != 0) attributes.add("responses");
return "Cannot build Operation, some of required attributes are not set " + attributes;
}
}
}