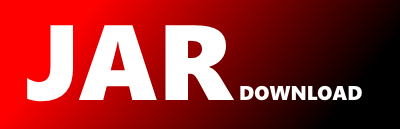
io.github.emm035.openapi.immutables.v3.PathItem Maven / Gradle / Ivy
package io.github.emm035.openapi.immutables.v3;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonAutoDetect;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.google.common.base.MoreObjects;
import com.google.common.collect.ImmutableList;
import com.google.common.collect.ImmutableMap;
import com.google.errorprone.annotations.CanIgnoreReturnValue;
import io.github.emm035.openapi.immutables.v3.parameters.Parameter;
import io.github.emm035.openapi.immutables.v3.references.Referenceable;
import io.github.emm035.openapi.immutables.v3.servers.Server;
import io.github.emm035.openapi.immutables.v3.shared.Describable;
import io.github.emm035.openapi.immutables.v3.shared.Extensible;
import io.github.emm035.openapi.immutables.v3.shared.HttpMethod;
import io.github.emm035.openapi.immutables.v3.shared.Summarizable;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.CheckReturnValue;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
/**
* Immutable implementation of {@link AbstractPathItem}.
*
* Use the builder to create immutable instances:
* {@code PathItem.builder()}.
*/
@SuppressWarnings({"all"})
@ParametersAreNonnullByDefault
@Immutable
@CheckReturnValue
public final class PathItem extends AbstractPathItem {
private final ImmutableList servers;
private final ImmutableList> parameters;
private final @Nullable Operation get;
private final @Nullable Operation put;
private final @Nullable Operation post;
private final @Nullable Operation patch;
private final @Nullable Operation delete;
private final @Nullable Operation options;
private final @Nullable Operation head;
private final @Nullable Operation trace;
private final Map operations;
private final @Nullable String summary;
private final @Nullable String description;
private final ImmutableMap extensions;
private PathItem(
ImmutableList servers,
ImmutableList> parameters,
@Nullable Operation get,
@Nullable Operation put,
@Nullable Operation post,
@Nullable Operation patch,
@Nullable Operation delete,
@Nullable Operation options,
@Nullable Operation head,
@Nullable Operation trace,
@Nullable String summary,
@Nullable String description,
ImmutableMap extensions) {
this.servers = servers;
this.parameters = parameters;
this.get = get;
this.put = put;
this.post = post;
this.patch = patch;
this.delete = delete;
this.options = options;
this.head = head;
this.trace = trace;
this.summary = summary;
this.description = description;
this.extensions = extensions;
this.operations = Objects.requireNonNull(super.getOperations(), "operations");
}
/**
* @return The value of the {@code servers} attribute
*/
@JsonProperty("servers")
@Override
public ImmutableList getServers() {
return servers;
}
/**
* @return The value of the {@code parameters} attribute
*/
@JsonProperty("parameters")
@Override
public ImmutableList> getParameters() {
return parameters;
}
/**
* @return The value of the {@code get} attribute
*/
@JsonProperty("get")
@Override
public Optional getGet() {
return Optional.ofNullable(get);
}
/**
* @return The value of the {@code put} attribute
*/
@JsonProperty("put")
@Override
public Optional getPut() {
return Optional.ofNullable(put);
}
/**
* @return The value of the {@code post} attribute
*/
@JsonProperty("post")
@Override
public Optional getPost() {
return Optional.ofNullable(post);
}
/**
* @return The value of the {@code patch} attribute
*/
@JsonProperty("patch")
@Override
public Optional getPatch() {
return Optional.ofNullable(patch);
}
/**
* @return The value of the {@code delete} attribute
*/
@JsonProperty("delete")
@Override
public Optional getDelete() {
return Optional.ofNullable(delete);
}
/**
* @return The value of the {@code options} attribute
*/
@JsonProperty("options")
@Override
public Optional getOptions() {
return Optional.ofNullable(options);
}
/**
* @return The value of the {@code head} attribute
*/
@JsonProperty("head")
@Override
public Optional getHead() {
return Optional.ofNullable(head);
}
/**
* @return The value of the {@code trace} attribute
*/
@JsonProperty("trace")
@Override
public Optional getTrace() {
return Optional.ofNullable(trace);
}
/**
* @return The computed-at-construction value of the {@code operations} attribute
*/
@JsonProperty("operations")
@JsonIgnore
@Override
public Map getOperations() {
return operations;
}
/**
* @return The value of the {@code summary} attribute
*/
@JsonProperty("summary")
@Override
public Optional getSummary() {
return Optional.ofNullable(summary);
}
/**
* @return The value of the {@code description} attribute
*/
@JsonProperty("description")
@Override
public Optional getDescription() {
return Optional.ofNullable(description);
}
/**
* @return The value of the {@code extensions} attribute
*/
@JsonProperty("extensions")
@JsonAnyGetter
@Override
public ImmutableMap getExtensions() {
return extensions;
}
/**
* Copy the current immutable object with elements that replace the content of {@link AbstractPathItem#getServers() servers}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final PathItem withServers(Server... elements) {
ImmutableList newValue = ImmutableList.copyOf(elements);
return validate(new PathItem(
newValue,
this.parameters,
this.get,
this.put,
this.post,
this.patch,
this.delete,
this.options,
this.head,
this.trace,
this.summary,
this.description,
this.extensions));
}
/**
* Copy the current immutable object with elements that replace the content of {@link AbstractPathItem#getServers() servers}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of servers elements to set
* @return A modified copy of {@code this} object
*/
public final PathItem withServers(Iterable extends Server> elements) {
if (this.servers == elements) return this;
ImmutableList newValue = ImmutableList.copyOf(elements);
return validate(new PathItem(
newValue,
this.parameters,
this.get,
this.put,
this.post,
this.patch,
this.delete,
this.options,
this.head,
this.trace,
this.summary,
this.description,
this.extensions));
}
/**
* Copy the current immutable object with elements that replace the content of {@link AbstractPathItem#getParameters() parameters}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
@SafeVarargs
public final PathItem withParameters(Referenceable... elements) {
ImmutableList> newValue = ImmutableList.copyOf(elements);
return validate(new PathItem(
this.servers,
newValue,
this.get,
this.put,
this.post,
this.patch,
this.delete,
this.options,
this.head,
this.trace,
this.summary,
this.description,
this.extensions));
}
/**
* Copy the current immutable object with elements that replace the content of {@link AbstractPathItem#getParameters() parameters}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of parameters elements to set
* @return A modified copy of {@code this} object
*/
public final PathItem withParameters(Iterable extends Referenceable> elements) {
if (this.parameters == elements) return this;
ImmutableList> newValue = ImmutableList.copyOf(elements);
return validate(new PathItem(
this.servers,
newValue,
this.get,
this.put,
this.post,
this.patch,
this.delete,
this.options,
this.head,
this.trace,
this.summary,
this.description,
this.extensions));
}
/**
* Copy the current immutable object by setting a present value for the optional {@link AbstractPathItem#getGet() get} attribute.
* @param value The value for get, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final PathItem withGet(@Nullable Operation value) {
@Nullable Operation newValue = value;
if (this.get == newValue) return this;
return validate(new PathItem(
this.servers,
this.parameters,
newValue,
this.put,
this.post,
this.patch,
this.delete,
this.options,
this.head,
this.trace,
this.summary,
this.description,
this.extensions));
}
/**
* Copy the current immutable object by setting an optional value for the {@link AbstractPathItem#getGet() get} attribute.
* A shallow reference equality check is used on unboxed optional value to prevent copying of the same value by returning {@code this}.
* @param optional A value for get
* @return A modified copy of {@code this} object
*/
public final PathItem withGet(Optional extends Operation> optional) {
@Nullable Operation value = optional.orElse(null);
if (this.get == value) return this;
return validate(new PathItem(
this.servers,
this.parameters,
value,
this.put,
this.post,
this.patch,
this.delete,
this.options,
this.head,
this.trace,
this.summary,
this.description,
this.extensions));
}
/**
* Copy the current immutable object by setting a present value for the optional {@link AbstractPathItem#getPut() put} attribute.
* @param value The value for put, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final PathItem withPut(@Nullable Operation value) {
@Nullable Operation newValue = value;
if (this.put == newValue) return this;
return validate(new PathItem(
this.servers,
this.parameters,
this.get,
newValue,
this.post,
this.patch,
this.delete,
this.options,
this.head,
this.trace,
this.summary,
this.description,
this.extensions));
}
/**
* Copy the current immutable object by setting an optional value for the {@link AbstractPathItem#getPut() put} attribute.
* A shallow reference equality check is used on unboxed optional value to prevent copying of the same value by returning {@code this}.
* @param optional A value for put
* @return A modified copy of {@code this} object
*/
public final PathItem withPut(Optional extends Operation> optional) {
@Nullable Operation value = optional.orElse(null);
if (this.put == value) return this;
return validate(new PathItem(
this.servers,
this.parameters,
this.get,
value,
this.post,
this.patch,
this.delete,
this.options,
this.head,
this.trace,
this.summary,
this.description,
this.extensions));
}
/**
* Copy the current immutable object by setting a present value for the optional {@link AbstractPathItem#getPost() post} attribute.
* @param value The value for post, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final PathItem withPost(@Nullable Operation value) {
@Nullable Operation newValue = value;
if (this.post == newValue) return this;
return validate(new PathItem(
this.servers,
this.parameters,
this.get,
this.put,
newValue,
this.patch,
this.delete,
this.options,
this.head,
this.trace,
this.summary,
this.description,
this.extensions));
}
/**
* Copy the current immutable object by setting an optional value for the {@link AbstractPathItem#getPost() post} attribute.
* A shallow reference equality check is used on unboxed optional value to prevent copying of the same value by returning {@code this}.
* @param optional A value for post
* @return A modified copy of {@code this} object
*/
public final PathItem withPost(Optional extends Operation> optional) {
@Nullable Operation value = optional.orElse(null);
if (this.post == value) return this;
return validate(new PathItem(
this.servers,
this.parameters,
this.get,
this.put,
value,
this.patch,
this.delete,
this.options,
this.head,
this.trace,
this.summary,
this.description,
this.extensions));
}
/**
* Copy the current immutable object by setting a present value for the optional {@link AbstractPathItem#getPatch() patch} attribute.
* @param value The value for patch, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final PathItem withPatch(@Nullable Operation value) {
@Nullable Operation newValue = value;
if (this.patch == newValue) return this;
return validate(new PathItem(
this.servers,
this.parameters,
this.get,
this.put,
this.post,
newValue,
this.delete,
this.options,
this.head,
this.trace,
this.summary,
this.description,
this.extensions));
}
/**
* Copy the current immutable object by setting an optional value for the {@link AbstractPathItem#getPatch() patch} attribute.
* A shallow reference equality check is used on unboxed optional value to prevent copying of the same value by returning {@code this}.
* @param optional A value for patch
* @return A modified copy of {@code this} object
*/
public final PathItem withPatch(Optional extends Operation> optional) {
@Nullable Operation value = optional.orElse(null);
if (this.patch == value) return this;
return validate(new PathItem(
this.servers,
this.parameters,
this.get,
this.put,
this.post,
value,
this.delete,
this.options,
this.head,
this.trace,
this.summary,
this.description,
this.extensions));
}
/**
* Copy the current immutable object by setting a present value for the optional {@link AbstractPathItem#getDelete() delete} attribute.
* @param value The value for delete, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final PathItem withDelete(@Nullable Operation value) {
@Nullable Operation newValue = value;
if (this.delete == newValue) return this;
return validate(new PathItem(
this.servers,
this.parameters,
this.get,
this.put,
this.post,
this.patch,
newValue,
this.options,
this.head,
this.trace,
this.summary,
this.description,
this.extensions));
}
/**
* Copy the current immutable object by setting an optional value for the {@link AbstractPathItem#getDelete() delete} attribute.
* A shallow reference equality check is used on unboxed optional value to prevent copying of the same value by returning {@code this}.
* @param optional A value for delete
* @return A modified copy of {@code this} object
*/
public final PathItem withDelete(Optional extends Operation> optional) {
@Nullable Operation value = optional.orElse(null);
if (this.delete == value) return this;
return validate(new PathItem(
this.servers,
this.parameters,
this.get,
this.put,
this.post,
this.patch,
value,
this.options,
this.head,
this.trace,
this.summary,
this.description,
this.extensions));
}
/**
* Copy the current immutable object by setting a present value for the optional {@link AbstractPathItem#getOptions() options} attribute.
* @param value The value for options, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final PathItem withOptions(@Nullable Operation value) {
@Nullable Operation newValue = value;
if (this.options == newValue) return this;
return validate(new PathItem(
this.servers,
this.parameters,
this.get,
this.put,
this.post,
this.patch,
this.delete,
newValue,
this.head,
this.trace,
this.summary,
this.description,
this.extensions));
}
/**
* Copy the current immutable object by setting an optional value for the {@link AbstractPathItem#getOptions() options} attribute.
* A shallow reference equality check is used on unboxed optional value to prevent copying of the same value by returning {@code this}.
* @param optional A value for options
* @return A modified copy of {@code this} object
*/
public final PathItem withOptions(Optional extends Operation> optional) {
@Nullable Operation value = optional.orElse(null);
if (this.options == value) return this;
return validate(new PathItem(
this.servers,
this.parameters,
this.get,
this.put,
this.post,
this.patch,
this.delete,
value,
this.head,
this.trace,
this.summary,
this.description,
this.extensions));
}
/**
* Copy the current immutable object by setting a present value for the optional {@link AbstractPathItem#getHead() head} attribute.
* @param value The value for head, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final PathItem withHead(@Nullable Operation value) {
@Nullable Operation newValue = value;
if (this.head == newValue) return this;
return validate(new PathItem(
this.servers,
this.parameters,
this.get,
this.put,
this.post,
this.patch,
this.delete,
this.options,
newValue,
this.trace,
this.summary,
this.description,
this.extensions));
}
/**
* Copy the current immutable object by setting an optional value for the {@link AbstractPathItem#getHead() head} attribute.
* A shallow reference equality check is used on unboxed optional value to prevent copying of the same value by returning {@code this}.
* @param optional A value for head
* @return A modified copy of {@code this} object
*/
public final PathItem withHead(Optional extends Operation> optional) {
@Nullable Operation value = optional.orElse(null);
if (this.head == value) return this;
return validate(new PathItem(
this.servers,
this.parameters,
this.get,
this.put,
this.post,
this.patch,
this.delete,
this.options,
value,
this.trace,
this.summary,
this.description,
this.extensions));
}
/**
* Copy the current immutable object by setting a present value for the optional {@link AbstractPathItem#getTrace() trace} attribute.
* @param value The value for trace, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final PathItem withTrace(@Nullable Operation value) {
@Nullable Operation newValue = value;
if (this.trace == newValue) return this;
return validate(new PathItem(
this.servers,
this.parameters,
this.get,
this.put,
this.post,
this.patch,
this.delete,
this.options,
this.head,
newValue,
this.summary,
this.description,
this.extensions));
}
/**
* Copy the current immutable object by setting an optional value for the {@link AbstractPathItem#getTrace() trace} attribute.
* A shallow reference equality check is used on unboxed optional value to prevent copying of the same value by returning {@code this}.
* @param optional A value for trace
* @return A modified copy of {@code this} object
*/
public final PathItem withTrace(Optional extends Operation> optional) {
@Nullable Operation value = optional.orElse(null);
if (this.trace == value) return this;
return validate(new PathItem(
this.servers,
this.parameters,
this.get,
this.put,
this.post,
this.patch,
this.delete,
this.options,
this.head,
value,
this.summary,
this.description,
this.extensions));
}
/**
* Copy the current immutable object by setting a present value for the optional {@link AbstractPathItem#getSummary() summary} attribute.
* @param value The value for summary, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final PathItem withSummary(@Nullable String value) {
@Nullable String newValue = value;
if (Objects.equals(this.summary, newValue)) return this;
return validate(new PathItem(
this.servers,
this.parameters,
this.get,
this.put,
this.post,
this.patch,
this.delete,
this.options,
this.head,
this.trace,
newValue,
this.description,
this.extensions));
}
/**
* Copy the current immutable object by setting an optional value for the {@link AbstractPathItem#getSummary() summary} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for summary
* @return A modified copy of {@code this} object
*/
public final PathItem withSummary(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.summary, value)) return this;
return validate(new PathItem(
this.servers,
this.parameters,
this.get,
this.put,
this.post,
this.patch,
this.delete,
this.options,
this.head,
this.trace,
value,
this.description,
this.extensions));
}
/**
* Copy the current immutable object by setting a present value for the optional {@link AbstractPathItem#getDescription() description} attribute.
* @param value The value for description, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final PathItem withDescription(@Nullable String value) {
@Nullable String newValue = value;
if (Objects.equals(this.description, newValue)) return this;
return validate(new PathItem(
this.servers,
this.parameters,
this.get,
this.put,
this.post,
this.patch,
this.delete,
this.options,
this.head,
this.trace,
this.summary,
newValue,
this.extensions));
}
/**
* Copy the current immutable object by setting an optional value for the {@link AbstractPathItem#getDescription() description} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for description
* @return A modified copy of {@code this} object
*/
public final PathItem withDescription(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.description, value)) return this;
return validate(new PathItem(
this.servers,
this.parameters,
this.get,
this.put,
this.post,
this.patch,
this.delete,
this.options,
this.head,
this.trace,
this.summary,
value,
this.extensions));
}
/**
* Copy the current immutable object by replacing the {@link AbstractPathItem#getExtensions() extensions} map with the specified map.
* Nulls are not permitted as keys or values.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param entries The entries to be added to the extensions map
* @return A modified copy of {@code this} object
*/
public final PathItem withExtensions(Map entries) {
if (this.extensions == entries) return this;
ImmutableMap newValue = ImmutableMap.copyOf(entries);
return validate(new PathItem(
this.servers,
this.parameters,
this.get,
this.put,
this.post,
this.patch,
this.delete,
this.options,
this.head,
this.trace,
this.summary,
this.description,
newValue));
}
/**
* This instance is equal to all instances of {@code PathItem} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof PathItem
&& equalTo((PathItem) another);
}
private boolean equalTo(PathItem another) {
return servers.equals(another.servers)
&& parameters.equals(another.parameters)
&& Objects.equals(get, another.get)
&& Objects.equals(put, another.put)
&& Objects.equals(post, another.post)
&& Objects.equals(patch, another.patch)
&& Objects.equals(delete, another.delete)
&& Objects.equals(options, another.options)
&& Objects.equals(head, another.head)
&& Objects.equals(trace, another.trace)
&& operations.equals(another.operations)
&& Objects.equals(summary, another.summary)
&& Objects.equals(description, another.description)
&& extensions.equals(another.extensions);
}
/**
* Computes a hash code from attributes: {@code servers}, {@code parameters}, {@code get}, {@code put}, {@code post}, {@code patch}, {@code delete}, {@code options}, {@code head}, {@code trace}, {@code operations}, {@code summary}, {@code description}, {@code extensions}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + servers.hashCode();
h += (h << 5) + parameters.hashCode();
h += (h << 5) + Objects.hashCode(get);
h += (h << 5) + Objects.hashCode(put);
h += (h << 5) + Objects.hashCode(post);
h += (h << 5) + Objects.hashCode(patch);
h += (h << 5) + Objects.hashCode(delete);
h += (h << 5) + Objects.hashCode(options);
h += (h << 5) + Objects.hashCode(head);
h += (h << 5) + Objects.hashCode(trace);
h += (h << 5) + operations.hashCode();
h += (h << 5) + Objects.hashCode(summary);
h += (h << 5) + Objects.hashCode(description);
h += (h << 5) + extensions.hashCode();
return h;
}
/**
* Prints the immutable value {@code PathItem} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return MoreObjects.toStringHelper("PathItem")
.omitNullValues()
.add("servers", servers)
.add("parameters", parameters)
.add("get", get)
.add("put", put)
.add("post", post)
.add("patch", patch)
.add("delete", delete)
.add("options", options)
.add("head", head)
.add("trace", trace)
.add("operations", operations)
.add("summary", summary)
.add("description", description)
.add("extensions", extensions)
.toString();
}
/**
* Utility type used to correctly read immutable object from JSON representation.
* @deprecated Do not use this type directly, it exists only for the Jackson-binding infrastructure
*/
@Deprecated
@JsonAutoDetect(fieldVisibility = JsonAutoDetect.Visibility.NONE)
static final class Json extends AbstractPathItem {
@Nullable List servers = ImmutableList.of();
@Nullable List> parameters = ImmutableList.of();
@Nullable Optional get = Optional.empty();
@Nullable Optional put = Optional.empty();
@Nullable Optional post = Optional.empty();
@Nullable Optional patch = Optional.empty();
@Nullable Optional delete = Optional.empty();
@Nullable Optional options = Optional.empty();
@Nullable Optional head = Optional.empty();
@Nullable Optional trace = Optional.empty();
@Nullable Optional summary = Optional.empty();
@Nullable Optional description = Optional.empty();
final Map extensions = new HashMap();
@JsonProperty("servers")
public void setServers(List servers) {
this.servers = servers;
}
@JsonProperty("parameters")
public void setParameters(List> parameters) {
this.parameters = parameters;
}
@JsonProperty("get")
public void setGet(Optional get) {
this.get = get;
}
@JsonProperty("put")
public void setPut(Optional put) {
this.put = put;
}
@JsonProperty("post")
public void setPost(Optional post) {
this.post = post;
}
@JsonProperty("patch")
public void setPatch(Optional patch) {
this.patch = patch;
}
@JsonProperty("delete")
public void setDelete(Optional delete) {
this.delete = delete;
}
@JsonProperty("options")
public void setOptions(Optional options) {
this.options = options;
}
@JsonProperty("head")
public void setHead(Optional head) {
this.head = head;
}
@JsonProperty("trace")
public void setTrace(Optional trace) {
this.trace = trace;
}
@JsonProperty("summary")
public void setSummary(Optional summary) {
this.summary = summary;
}
@JsonProperty("description")
public void setDescription(Optional description) {
this.description = description;
}
@JsonAnySetter
public void setExtensions(String key, Object value) {
this.extensions.put(key, value);
}
@Override
public List getServers() { throw new UnsupportedOperationException(); }
@Override
public List> getParameters() { throw new UnsupportedOperationException(); }
@Override
public Optional getGet() { throw new UnsupportedOperationException(); }
@Override
public Optional getPut() { throw new UnsupportedOperationException(); }
@Override
public Optional getPost() { throw new UnsupportedOperationException(); }
@Override
public Optional getPatch() { throw new UnsupportedOperationException(); }
@Override
public Optional getDelete() { throw new UnsupportedOperationException(); }
@Override
public Optional getOptions() { throw new UnsupportedOperationException(); }
@Override
public Optional getHead() { throw new UnsupportedOperationException(); }
@Override
public Optional getTrace() { throw new UnsupportedOperationException(); }
@Override
public Map getOperations() { throw new UnsupportedOperationException(); }
@Override
public Optional getSummary() { throw new UnsupportedOperationException(); }
@Override
public Optional getDescription() { throw new UnsupportedOperationException(); }
@Override
public Map getExtensions() { throw new UnsupportedOperationException(); }
}
/**
* @param json A JSON-bindable data structure
* @return An immutable value type
* @deprecated Do not use this method directly, it exists only for the Jackson-binding infrastructure
*/
@Deprecated
@JsonCreator(mode = JsonCreator.Mode.DELEGATING)
static PathItem fromJson(Json json) {
PathItem.Builder builder = PathItem.builder();
if (json.servers != null) {
builder.addAllServers(json.servers);
}
if (json.parameters != null) {
builder.addAllParameters(json.parameters);
}
if (json.get != null) {
builder.setGet(json.get);
}
if (json.put != null) {
builder.setPut(json.put);
}
if (json.post != null) {
builder.setPost(json.post);
}
if (json.patch != null) {
builder.setPatch(json.patch);
}
if (json.delete != null) {
builder.setDelete(json.delete);
}
if (json.options != null) {
builder.setOptions(json.options);
}
if (json.head != null) {
builder.setHead(json.head);
}
if (json.trace != null) {
builder.setTrace(json.trace);
}
if (json.summary != null) {
builder.setSummary(json.summary);
}
if (json.description != null) {
builder.setDescription(json.description);
}
if (json.extensions != null) {
builder.putAllExtensions(json.extensions);
}
return builder.build();
}
private static PathItem validate(PathItem instance) {
instance = (PathItem) instance.normalizeExtensions();
return instance;
}
/**
* Creates an immutable copy of a {@link AbstractPathItem} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable PathItem instance
*/
public static PathItem copyOf(AbstractPathItem instance) {
if (instance instanceof PathItem) {
return (PathItem) instance;
}
return PathItem.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link PathItem PathItem}.
* @return A new PathItem builder
*/
public static PathItem.Builder builder() {
return new PathItem.Builder();
}
/**
* Builds instances of type {@link PathItem PathItem}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@NotThreadSafe
public static final class Builder {
private ImmutableList.Builder servers = ImmutableList.builder();
private ImmutableList.Builder> parameters = ImmutableList.builder();
private @Nullable Operation get;
private @Nullable Operation put;
private @Nullable Operation post;
private @Nullable Operation patch;
private @Nullable Operation delete;
private @Nullable Operation options;
private @Nullable Operation head;
private @Nullable Operation trace;
private @Nullable String summary;
private @Nullable String description;
private ImmutableMap.Builder extensions = ImmutableMap.builder();
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code io.github.emm035.openapi.immutables.v3.shared.Summarizable} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder from(Summarizable instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return this;
}
/**
* Fill a builder with attribute values from the provided {@code io.github.emm035.openapi.immutables.v3.AbstractPathItem} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder from(AbstractPathItem instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return this;
}
/**
* Fill a builder with attribute values from the provided {@code io.github.emm035.openapi.immutables.v3.shared.Extensible} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder from(Extensible instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return this;
}
/**
* Fill a builder with attribute values from the provided {@code io.github.emm035.openapi.immutables.v3.shared.Describable} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder from(Describable instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return this;
}
private void from(Object object) {
if (object instanceof Summarizable) {
Summarizable instance = (Summarizable) object;
Optional summaryOptional = instance.getSummary();
if (summaryOptional.isPresent()) {
setSummary(summaryOptional);
}
}
if (object instanceof AbstractPathItem) {
AbstractPathItem instance = (AbstractPathItem) object;
Optional patchOptional = instance.getPatch();
if (patchOptional.isPresent()) {
setPatch(patchOptional);
}
Optional headOptional = instance.getHead();
if (headOptional.isPresent()) {
setHead(headOptional);
}
Optional traceOptional = instance.getTrace();
if (traceOptional.isPresent()) {
setTrace(traceOptional);
}
addAllServers(instance.getServers());
Optional postOptional = instance.getPost();
if (postOptional.isPresent()) {
setPost(postOptional);
}
Optional getOptional = instance.getGet();
if (getOptional.isPresent()) {
setGet(getOptional);
}
Optional optionsOptional = instance.getOptions();
if (optionsOptional.isPresent()) {
setOptions(optionsOptional);
}
Optional deleteOptional = instance.getDelete();
if (deleteOptional.isPresent()) {
setDelete(deleteOptional);
}
addAllParameters(instance.getParameters());
Optional putOptional = instance.getPut();
if (putOptional.isPresent()) {
setPut(putOptional);
}
}
if (object instanceof Extensible) {
Extensible instance = (Extensible) object;
putAllExtensions(instance.getExtensions());
}
if (object instanceof Describable) {
Describable instance = (Describable) object;
Optional descriptionOptional = instance.getDescription();
if (descriptionOptional.isPresent()) {
setDescription(descriptionOptional);
}
}
}
/**
* Adds one element to {@link AbstractPathItem#getServers() servers} list.
* @param element A servers element
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addServers(Server element) {
this.servers.add(element);
return this;
}
/**
* Adds elements to {@link AbstractPathItem#getServers() servers} list.
* @param elements An array of servers elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addServers(Server... elements) {
this.servers.add(elements);
return this;
}
/**
* Sets or replaces all elements for {@link AbstractPathItem#getServers() servers} list.
* @param elements An iterable of servers elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder setServers(Iterable extends Server> elements) {
this.servers = ImmutableList.builder();
return addAllServers(elements);
}
/**
* Adds elements to {@link AbstractPathItem#getServers() servers} list.
* @param elements An iterable of servers elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addAllServers(Iterable extends Server> elements) {
this.servers.addAll(elements);
return this;
}
/**
* Adds one element to {@link AbstractPathItem#getParameters() parameters} list.
* @param element A parameters element
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addParameters(Referenceable element) {
this.parameters.add(element);
return this;
}
/**
* Adds elements to {@link AbstractPathItem#getParameters() parameters} list.
* @param elements An array of parameters elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@SafeVarargs
public final Builder addParameters(Referenceable... elements) {
this.parameters.add(elements);
return this;
}
/**
* Sets or replaces all elements for {@link AbstractPathItem#getParameters() parameters} list.
* @param elements An iterable of parameters elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder setParameters(Iterable extends Referenceable> elements) {
this.parameters = ImmutableList.builder();
return addAllParameters(elements);
}
/**
* Adds elements to {@link AbstractPathItem#getParameters() parameters} list.
* @param elements An iterable of parameters elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addAllParameters(Iterable extends Referenceable> elements) {
this.parameters.addAll(elements);
return this;
}
/**
* Initializes the optional value {@link AbstractPathItem#getGet() get} to get.
* @param get The value for get, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
@CanIgnoreReturnValue
public final Builder setGet(@Nullable Operation get) {
this.get = get;
return this;
}
/**
* Initializes the optional value {@link AbstractPathItem#getGet() get} to get.
* @param get The value for get
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder setGet(Optional extends Operation> get) {
this.get = get.orElse(null);
return this;
}
/**
* Initializes the optional value {@link AbstractPathItem#getPut() put} to put.
* @param put The value for put, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
@CanIgnoreReturnValue
public final Builder setPut(@Nullable Operation put) {
this.put = put;
return this;
}
/**
* Initializes the optional value {@link AbstractPathItem#getPut() put} to put.
* @param put The value for put
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder setPut(Optional extends Operation> put) {
this.put = put.orElse(null);
return this;
}
/**
* Initializes the optional value {@link AbstractPathItem#getPost() post} to post.
* @param post The value for post, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
@CanIgnoreReturnValue
public final Builder setPost(@Nullable Operation post) {
this.post = post;
return this;
}
/**
* Initializes the optional value {@link AbstractPathItem#getPost() post} to post.
* @param post The value for post
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder setPost(Optional extends Operation> post) {
this.post = post.orElse(null);
return this;
}
/**
* Initializes the optional value {@link AbstractPathItem#getPatch() patch} to patch.
* @param patch The value for patch, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
@CanIgnoreReturnValue
public final Builder setPatch(@Nullable Operation patch) {
this.patch = patch;
return this;
}
/**
* Initializes the optional value {@link AbstractPathItem#getPatch() patch} to patch.
* @param patch The value for patch
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder setPatch(Optional extends Operation> patch) {
this.patch = patch.orElse(null);
return this;
}
/**
* Initializes the optional value {@link AbstractPathItem#getDelete() delete} to delete.
* @param delete The value for delete, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
@CanIgnoreReturnValue
public final Builder setDelete(@Nullable Operation delete) {
this.delete = delete;
return this;
}
/**
* Initializes the optional value {@link AbstractPathItem#getDelete() delete} to delete.
* @param delete The value for delete
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder setDelete(Optional extends Operation> delete) {
this.delete = delete.orElse(null);
return this;
}
/**
* Initializes the optional value {@link AbstractPathItem#getOptions() options} to options.
* @param options The value for options, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
@CanIgnoreReturnValue
public final Builder setOptions(@Nullable Operation options) {
this.options = options;
return this;
}
/**
* Initializes the optional value {@link AbstractPathItem#getOptions() options} to options.
* @param options The value for options
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder setOptions(Optional extends Operation> options) {
this.options = options.orElse(null);
return this;
}
/**
* Initializes the optional value {@link AbstractPathItem#getHead() head} to head.
* @param head The value for head, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
@CanIgnoreReturnValue
public final Builder setHead(@Nullable Operation head) {
this.head = head;
return this;
}
/**
* Initializes the optional value {@link AbstractPathItem#getHead() head} to head.
* @param head The value for head
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder setHead(Optional extends Operation> head) {
this.head = head.orElse(null);
return this;
}
/**
* Initializes the optional value {@link AbstractPathItem#getTrace() trace} to trace.
* @param trace The value for trace, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
@CanIgnoreReturnValue
public final Builder setTrace(@Nullable Operation trace) {
this.trace = trace;
return this;
}
/**
* Initializes the optional value {@link AbstractPathItem#getTrace() trace} to trace.
* @param trace The value for trace
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder setTrace(Optional extends Operation> trace) {
this.trace = trace.orElse(null);
return this;
}
/**
* Initializes the optional value {@link AbstractPathItem#getSummary() summary} to summary.
* @param summary The value for summary, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
@CanIgnoreReturnValue
public final Builder setSummary(@Nullable String summary) {
this.summary = summary;
return this;
}
/**
* Initializes the optional value {@link AbstractPathItem#getSummary() summary} to summary.
* @param summary The value for summary
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder setSummary(Optional summary) {
this.summary = summary.orElse(null);
return this;
}
/**
* Initializes the optional value {@link AbstractPathItem#getDescription() description} to description.
* @param description The value for description, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
@CanIgnoreReturnValue
public final Builder setDescription(@Nullable String description) {
this.description = description;
return this;
}
/**
* Initializes the optional value {@link AbstractPathItem#getDescription() description} to description.
* @param description The value for description
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder setDescription(Optional description) {
this.description = description.orElse(null);
return this;
}
/**
* Put one entry to the {@link AbstractPathItem#getExtensions() extensions} map.
* @param key The key in the extensions map
* @param value The associated value in the extensions map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonAnySetter
public final Builder putExtensions(String key, Object value) {
this.extensions.put(key, value);
return this;
}
/**
* Put one entry to the {@link AbstractPathItem#getExtensions() extensions} map. Nulls are not permitted
* @param entry The key and value entry
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putExtensions(Map.Entry entry) {
this.extensions.put(entry);
return this;
}
/**
* Sets or replaces all mappings from the specified map as entries for the {@link AbstractPathItem#getExtensions() extensions} map. Nulls are not permitted
* @param extensions The entries that will be added to the extensions map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder setExtensions(Map extensions) {
this.extensions = ImmutableMap.builder();
return putAllExtensions(extensions);
}
/**
* Put all mappings from the specified map as entries to {@link AbstractPathItem#getExtensions() extensions} map. Nulls are not permitted
* @param extensions The entries that will be added to the extensions map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putAllExtensions(Map extensions) {
this.extensions.putAll(extensions);
return this;
}
/**
* Builds a new {@link PathItem PathItem}.
* @return An immutable instance of PathItem
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public PathItem build() {
return PathItem.validate(new PathItem(
servers.build(),
parameters.build(),
get,
put,
post,
patch,
delete,
options,
head,
trace,
summary,
description,
extensions.build()));
}
}
}