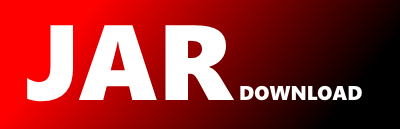
io.github.emm035.openapi.immutables.v3.responses.Responses Maven / Gradle / Ivy
Show all versions of openapi-immutables Show documentation
package io.github.emm035.openapi.immutables.v3.responses;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonAutoDetect;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonUnwrapped;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.google.common.base.MoreObjects;
import com.google.common.collect.ImmutableMap;
import com.google.errorprone.annotations.CanIgnoreReturnValue;
import io.github.emm035.openapi.immutables.v3.references.Referenceable;
import io.github.emm035.openapi.immutables.v3.shared.Extensible;
import java.util.HashMap;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.CheckReturnValue;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
/**
* Immutable implementation of {@link AbstractResponses}.
*
* Use the builder to create immutable instances:
* {@code Responses.builder()}.
*/
@SuppressWarnings({"all"})
@ParametersAreNonnullByDefault
@Immutable
@CheckReturnValue
public final class Responses extends AbstractResponses {
private final @Nullable Referenceable getDefault;
private final ImmutableMap> responses;
private final ImmutableMap extensions;
private Responses(
@Nullable Referenceable getDefault,
ImmutableMap> responses,
ImmutableMap extensions) {
this.getDefault = getDefault;
this.responses = responses;
this.extensions = extensions;
}
/**
* @return The value of the {@code getDefault} attribute
*/
@JsonProperty("default")
@Override
public Optional> getDefault() {
return Optional.ofNullable(getDefault);
}
/**
* @return The value of the {@code responses} attribute
*/
@JsonProperty("responses")
@JsonUnwrapped
@Override
public ImmutableMap> getResponses() {
return responses;
}
/**
* @return The value of the {@code extensions} attribute
*/
@JsonProperty("extensions")
@JsonAnyGetter
@Override
public ImmutableMap getExtensions() {
return extensions;
}
/**
* Copy the current immutable object by setting a present value for the optional {@link AbstractResponses#getDefault() default} attribute.
* @param value The value for getDefault, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final Responses withDefault(@Nullable Referenceable value) {
@Nullable Referenceable newValue = value;
if (this.getDefault == newValue) return this;
return validate(new Responses(newValue, this.responses, this.extensions));
}
/**
* Copy the current immutable object by setting an optional value for the {@link AbstractResponses#getDefault() default} attribute.
* A shallow reference equality check is used on unboxed optional value to prevent copying of the same value by returning {@code this}.
* @param optional A value for getDefault
* @return A modified copy of {@code this} object
*/
public final Responses withDefault(Optional> optional) {
@Nullable Referenceable value = optional.orElse(null);
if (this.getDefault == value) return this;
return validate(new Responses(value, this.responses, this.extensions));
}
/**
* Copy the current immutable object by replacing the {@link AbstractResponses#getResponses() responses} map with the specified map.
* Nulls are not permitted as keys or values.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param entries The entries to be added to the responses map
* @return A modified copy of {@code this} object
*/
public final Responses withResponses(Map> entries) {
if (this.responses == entries) return this;
ImmutableMap> newValue = ImmutableMap.copyOf(entries);
return validate(new Responses(this.getDefault, newValue, this.extensions));
}
/**
* Copy the current immutable object by replacing the {@link AbstractResponses#getExtensions() extensions} map with the specified map.
* Nulls are not permitted as keys or values.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param entries The entries to be added to the extensions map
* @return A modified copy of {@code this} object
*/
public final Responses withExtensions(Map entries) {
if (this.extensions == entries) return this;
ImmutableMap newValue = ImmutableMap.copyOf(entries);
return validate(new Responses(this.getDefault, this.responses, newValue));
}
/**
* This instance is equal to all instances of {@code Responses} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof Responses
&& equalTo((Responses) another);
}
private boolean equalTo(Responses another) {
return Objects.equals(getDefault, another.getDefault)
&& responses.equals(another.responses)
&& extensions.equals(another.extensions);
}
/**
* Computes a hash code from attributes: {@code getDefault}, {@code responses}, {@code extensions}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + Objects.hashCode(getDefault);
h += (h << 5) + responses.hashCode();
h += (h << 5) + extensions.hashCode();
return h;
}
/**
* Prints the immutable value {@code Responses} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return MoreObjects.toStringHelper("Responses")
.omitNullValues()
.add("default", getDefault)
.add("responses", responses)
.add("extensions", extensions)
.toString();
}
/**
* Utility type used to correctly read immutable object from JSON representation.
* @deprecated Do not use this type directly, it exists only for the Jackson-binding infrastructure
*/
@Deprecated
@JsonDeserialize
@JsonAutoDetect(fieldVisibility = JsonAutoDetect.Visibility.NONE)
static final class Json extends AbstractResponses {
@Nullable Optional> getDefault = Optional.empty();
@Nullable Map> responses = ImmutableMap.of();
final Map extensions = new HashMap();
@JsonProperty("default")
public void setDefault(Optional> getDefault) {
this.getDefault = getDefault;
}
@JsonProperty("responses")
@JsonUnwrapped
public void setResponses(Map> responses) {
this.responses = responses;
}
@JsonAnySetter
public void setExtensions(String key, Object value) {
this.extensions.put(key, value);
}
@Override
public Optional> getDefault() { throw new UnsupportedOperationException(); }
@Override
public Map> getResponses() { throw new UnsupportedOperationException(); }
@Override
public Map getExtensions() { throw new UnsupportedOperationException(); }
}
/**
* @param json A JSON-bindable data structure
* @return An immutable value type
* @deprecated Do not use this method directly, it exists only for the Jackson-binding infrastructure
*/
@Deprecated
@JsonCreator(mode = JsonCreator.Mode.DELEGATING)
static Responses fromJson(Json json) {
Responses.Builder builder = Responses.builder();
if (json.getDefault != null) {
builder.setDefault(json.getDefault);
}
if (json.responses != null) {
builder.putAllResponses(json.responses);
}
if (json.extensions != null) {
builder.putAllExtensions(json.extensions);
}
return builder.build();
}
private static Responses validate(Responses instance) {
instance = (Responses) instance.normalizeExtensions();
return instance;
}
/**
* Creates an immutable copy of a {@link AbstractResponses} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable Responses instance
*/
public static Responses copyOf(AbstractResponses instance) {
if (instance instanceof Responses) {
return (Responses) instance;
}
return Responses.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link Responses Responses}.
* @return A new Responses builder
*/
public static Responses.Builder builder() {
return new Responses.Builder();
}
/**
* Builds instances of type {@link Responses Responses}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
*
{@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@NotThreadSafe
public static final class Builder {
private @Nullable Referenceable getDefault;
private ImmutableMap.Builder> responses = ImmutableMap.builder();
private ImmutableMap.Builder extensions = ImmutableMap.builder();
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code io.github.emm035.openapi.immutables.v3.responses.AbstractResponses} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder from(AbstractResponses instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return this;
}
/**
* Fill a builder with attribute values from the provided {@code io.github.emm035.openapi.immutables.v3.shared.Extensible} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder from(Extensible instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return this;
}
private void from(Object object) {
if (object instanceof AbstractResponses) {
AbstractResponses instance = (AbstractResponses) object;
putAllResponses(instance.getResponses());
Optional> getDefaultOptional = instance.getDefault();
if (getDefaultOptional.isPresent()) {
setDefault(getDefaultOptional);
}
}
if (object instanceof Extensible) {
Extensible instance = (Extensible) object;
putAllExtensions(instance.getExtensions());
}
}
/**
* Initializes the optional value {@link AbstractResponses#getDefault() default} to getDefault.
* @param getDefault The value for getDefault, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
@CanIgnoreReturnValue
public final Builder setDefault(@Nullable Referenceable getDefault) {
this.getDefault = getDefault;
return this;
}
/**
* Initializes the optional value {@link AbstractResponses#getDefault() default} to getDefault.
* @param getDefault The value for getDefault
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("default")
public final Builder setDefault(Optional> getDefault) {
this.getDefault = getDefault.orElse(null);
return this;
}
/**
* Put one entry to the {@link AbstractResponses#getResponses() responses} map.
* @param key The key in the responses map
* @param value The associated value in the responses map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putResponses(int key, Referenceable value) {
this.responses.put(key, value);
return this;
}
/**
* Put one entry to the {@link AbstractResponses#getResponses() responses} map. Nulls are not permitted
* @param entry The key and value entry
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putResponses(Map.Entry> entry) {
this.responses.put(entry);
return this;
}
/**
* Sets or replaces all mappings from the specified map as entries for the {@link AbstractResponses#getResponses() responses} map. Nulls are not permitted
* @param responses The entries that will be added to the responses map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("responses")
@JsonUnwrapped
public final Builder setResponses(Map> responses) {
this.responses = ImmutableMap.builder();
return putAllResponses(responses);
}
/**
* Put all mappings from the specified map as entries to {@link AbstractResponses#getResponses() responses} map. Nulls are not permitted
* @param responses The entries that will be added to the responses map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putAllResponses(Map> responses) {
this.responses.putAll(responses);
return this;
}
/**
* Put one entry to the {@link AbstractResponses#getExtensions() extensions} map.
* @param key The key in the extensions map
* @param value The associated value in the extensions map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonAnySetter
public final Builder putExtensions(String key, Object value) {
this.extensions.put(key, value);
return this;
}
/**
* Put one entry to the {@link AbstractResponses#getExtensions() extensions} map. Nulls are not permitted
* @param entry The key and value entry
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putExtensions(Map.Entry entry) {
this.extensions.put(entry);
return this;
}
/**
* Sets or replaces all mappings from the specified map as entries for the {@link AbstractResponses#getExtensions() extensions} map. Nulls are not permitted
* @param extensions The entries that will be added to the extensions map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("extensions")
public final Builder setExtensions(Map extensions) {
this.extensions = ImmutableMap.builder();
return putAllExtensions(extensions);
}
/**
* Put all mappings from the specified map as entries to {@link AbstractResponses#getExtensions() extensions} map. Nulls are not permitted
* @param extensions The entries that will be added to the extensions map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putAllExtensions(Map extensions) {
this.extensions.putAll(extensions);
return this;
}
/**
* Builds a new {@link Responses Responses}.
* @return An immutable instance of Responses
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public Responses build() {
return Responses.validate(new Responses(getDefault, responses.build(), extensions.build()));
}
}
}