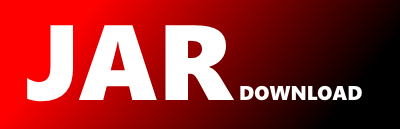
src.org.jafer.sru.bridge.SRUtoSRWBridge Maven / Gradle / Ivy
/**
* JAFER Toolkit Project. Copyright (C) 2002, JAFER Toolkit Project, Oxford
* University. This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation; either version 2.1 of the License,
* or (at your option) any later version. This library is distributed in the
* hope that it will be useful, but WITHOUT ANY WARRANTY; without even the
* implied warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See
* the GNU Lesser General Public License for more details. You should have
* received a copy of the GNU Lesser General Public License along with this
* library; if not, write to the Free Software Foundation, Inc., 59 Temple
* Place, Suite 330, Boston, MA 02111-1307 USA
*/
package org.jafer.sru.bridge;
import java.net.MalformedURLException;
import java.net.URL;
import java.util.Map;
import org.jafer.sru.SRUException;
import org.jafer.sru.SRUtoSRWConfig;
/**
* This class processes an SRU operation by calling the prefined SRW instance
* and returning the result to the caller. The SOAP envelope will be excluded
* from any responses.
*/
public class SRUtoSRWBridge extends V1Bridge
{
/**
* Stores a reference to the web service URL.
*/
private URL serviceURL = null;
/**
* Stores a reference to key used to find the requested operation
*/
private static final String OPERATION_KEY = "operation";
/**
* Stores a reference to key used to find the requested operation
*/
private static final String VERSION_KEY = "version";
/**
* Stores a reference to explain operation name
*/
private static final String EXPLAIN_OPERATION = "explain";
/**
* Stores a reference to explain operation name
*/
private static final String DEFAULT_VERSION = "1.1";
/**
* Stores a reference to configuration information for the bridge
*/
private SRUtoSRWConfig config = null;
/**
* Creates an instance of the SRUtoSRWBridge specifying the URL to use to
* find the web service
*
* @param url The url to use with this web service handler
* @throws SRUException
* @throws MalformedURLException
*/
public SRUtoSRWBridge(String url) throws SRUException, MalformedURLException
{
serviceURL = new URL(url);
this.config = new SRUtoSRWConfig();
}
/**
* Processes the request given the map of parameters
*
* @param parameters The paramaters that defines the SRU call in form Key to
* StringValue
* @return The XML response generated by the request
*/
public String processRequest(Map parameters)
{
String operation = "";
try
{
// extract out from the parameters map the operation being performed
operation = (String) parameters.get(OPERATION_KEY);
// extract out from the parameters map the version of the operation
// being performed
String version = (String) parameters.get(VERSION_KEY);
// if no operation has been specified or it is blank then default to
// 'explain'
if (operation == null || operation.length() == 0)
{
operation = EXPLAIN_OPERATION;
}
// if no version has been specified or it is blank then default to
// '1.1'
if (version == null || version.length() == 0)
{
version = DEFAULT_VERSION;
}
// create the request type for the operation
OperationBridge bridge = (OperationBridge) createOperationBridgeClass(operation, version);
// execute the operation using the bridge supplying parameters
return bridge.execute(parameters, serviceURL);
}
catch (SRUException exc)
{
// internal error so return basic explain reponse
exc.printStackTrace();
return createExplainDiagnosticResponse("1", exc.getMessage(), "", exc);
}
}
/**
* Instantiates and returns the bridge class for the specified operation and
* version
*
* @param operation The operation being performed
* @param version The operations version
* @return The intantiated bridge class
* @throws SRUException
*/
private OperationBridge createOperationBridgeClass(String operation, String version) throws SRUException
{
// get the root operation config node
String bridgeClassName = config.getOperationBridgeClass(operation, version);
if (bridgeClassName == null)
{
throw new SRUException("Unable to find supporting bridge for " + operation + " operation in configuration");
}
// construct the request type;
try
{
return (OperationBridge) (Class.forName(bridgeClassName).newInstance());
}
catch (Exception exc)
{
exc.printStackTrace();
throw new SRUException("Unable to create bridge object for operation: " + operation);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy