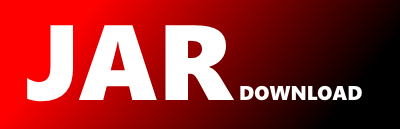
org.everit.json.schema.SchemaException Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of everit-json-schema Show documentation
Show all versions of everit-json-schema Show documentation
Implementation of the JSON Schema Core Draft v4 specification built with the org.json API
package org.everit.json.schema;
import static java.lang.String.format;
import static java.util.Arrays.asList;
import static java.util.Objects.requireNonNull;
import static java.util.stream.Collectors.joining;
import java.util.Collection;
import java.util.List;
import org.json.JSONObject;
/**
* Thrown by {@link org.everit.json.schema.loader.SchemaLoader#load()} when it encounters
* un-parseable schema JSON definition.
*
* @author erosb
*/
public class SchemaException extends RuntimeException {
private static final long serialVersionUID = 5987489689035036987L;
private static Object typeOfValue(final Object actualValue) {
return actualValue == null ? "null" : actualValue.getClass().getSimpleName();
}
static String buildMessage(String pointer, Class> actualType, Class> expectedType, Class>... furtherExpectedTypes) {
requireNonNull(pointer, "pointer cannot be null");
String actualTypeDescr = actualTypeDescr(actualType);
if (furtherExpectedTypes != null && furtherExpectedTypes.length > 0) {
Class>[] allExpecteds = new Class>[furtherExpectedTypes.length + 1];
allExpecteds[0] = expectedType;
System.arraycopy(furtherExpectedTypes, 0, allExpecteds, 1, furtherExpectedTypes.length);
return buildMessage(pointer, actualTypeDescr, asList(allExpecteds));
}
return format("%s: expected type: %s, found: %s", pointer,
expectedType.getSimpleName(),
actualTypeDescr);
}
private static String actualTypeDescr(Class> actualType) {
return actualType == null ? "null" : actualType.getSimpleName();
}
static String buildMessage(String formattedPointer, String actualTypeDescr, Collection> expectedTypes) {
String fmtExpectedTypes = expectedTypes.stream()
.map(Class::getSimpleName)
.collect(joining(" or "));
return format("%s: expected type is one of %s, found: %s", formattedPointer,
fmtExpectedTypes,
actualTypeDescr);
}
private static String buildMessage(String pointer, Class> actualType, Collection> expectedTypes) {
return buildMessage(pointer, actualTypeDescr(actualType), expectedTypes);
}
private static String joinClassNames(final List> expectedTypes) {
return expectedTypes.stream().map(Class::getSimpleName).collect(joining(", "));
}
private final String schemaLocation;
public SchemaException(String schemaLocation, String message) {
super(schemaLocation == null
? ": " + message
: schemaLocation + ": " + message);
this.schemaLocation = schemaLocation;
}
public SchemaException(String schemaLocation, Class> actualType, Class> expectedType, Class>... furtherExpectedTypes) {
super(buildMessage(schemaLocation, actualType, expectedType, furtherExpectedTypes));
this.schemaLocation = schemaLocation;
}
public SchemaException(String schemaLocation, Class> actualType, Collection> expectedTypes) {
super(buildMessage(schemaLocation, actualType, expectedTypes));
this.schemaLocation = schemaLocation;
}
public SchemaException(String schemaLocation, Exception cause) {
super(cause.getMessage(), cause);
this.schemaLocation = schemaLocation;
}
@Deprecated
public SchemaException(String message) {
this((String) null, message);
}
@Deprecated
public SchemaException(String key, Class> expectedType, Object actualValue) {
this(format("key %s : expected type: %s , found : %s", key, expectedType
.getSimpleName(), typeOfValue(actualValue)));
}
@Deprecated
public SchemaException(String key, List> expectedTypes,
final Object actualValue) {
this(format("key %s: expected type is one of %s, found: %s",
key, joinClassNames(expectedTypes), typeOfValue(actualValue)));
}
@Deprecated
public SchemaException(String message, Throwable cause) {
super(message, cause);
this.schemaLocation = null;
}
@Override public boolean equals(Object o) {
if (this == o)
return true;
if (o == null || getClass() != o.getClass())
return false;
SchemaException that = (SchemaException) o;
return toString().equals(that.toString());
}
@Override public int hashCode() {
return toString().hashCode();
}
public String getSchemaLocation() {
return schemaLocation;
}
public JSONObject toJSON() {
JSONObject rval = new JSONObject();
rval.put("schemaLocation", schemaLocation);
rval.put("message", getMessage());
return rval;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy