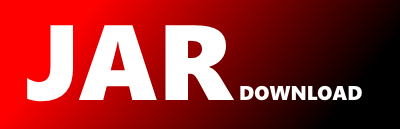
org.everit.json.schema.internal.JsonPointerFormatValidator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of everit-json-schema Show documentation
Show all versions of everit-json-schema Show documentation
Implementation of the JSON Schema Core Draft v4 specification built with the org.json API
package org.everit.json.schema.internal;
import static java.lang.String.format;
import java.util.Optional;
import org.everit.json.schema.FormatValidator;
import org.everit.json.schema.JSONPointer;
public class JsonPointerFormatValidator implements FormatValidator {
@Override public Optional validate(String subject) {
if ("".equals(subject)) {
return Optional.empty();
}
try {
new JSONPointer(subject);
if (subject.startsWith("#")) {
return failure(subject);
}
return checkEscaping(subject);
} catch (IllegalArgumentException e) {
return failure(subject);
}
}
protected Optional failure(String subject) {
return Optional.of(format("[%s] is not a valid JSON pointer", subject));
}
protected Optional checkEscaping(String subject) {
for (int i = 0; i < subject.length() - 1; ++i) {
char c = subject.charAt(i);
if (c == '~') {
char next = subject.charAt(i + 1);
if (next == '1' || next == '0') {
continue;
}
return failure(subject);
}
}
if (subject.charAt(subject.length() - 1) == '~') {
return failure(subject);
}
return Optional.empty();
}
@Override public String formatName() {
return "json-pointer";
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy