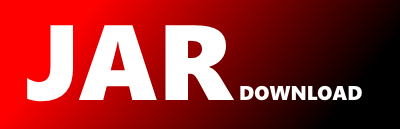
com.amazonaws.services.ec2.model.DescribeInstancesRequest Maven / Gradle / Ivy
Show all versions of aws-sdk-android Show documentation
/*
* Copyright 2010-2015 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.ec2.model;
import java.io.Serializable;
import com.amazonaws.AmazonWebServiceRequest;
import com.amazonaws.Request;
import com.amazonaws.services.ec2.model.transform.DescribeInstancesRequestMarshaller;
/**
* Container for the parameters to the {@link com.amazonaws.services.ec2.AmazonEC2#describeInstances(DescribeInstancesRequest) DescribeInstances operation}.
*
* Describes one or more of your instances.
*
*
* If you specify one or more instance IDs, Amazon EC2 returns
* information for those instances. If you do not specify instance IDs,
* Amazon EC2 returns information for all relevant instances. If you
* specify an instance ID that is not valid, an error is returned. If you
* specify an instance that you do not own, it is not included in the
* returned results.
*
*
* Recently terminated instances might appear in the returned results.
* This interval is usually less than one hour.
*
*
* @see com.amazonaws.services.ec2.AmazonEC2#describeInstances(DescribeInstancesRequest)
*/
public class DescribeInstancesRequest extends AmazonWebServiceRequest implements Serializable, DryRunSupportedRequest {
/**
* One or more instance IDs. Default: Describes all your instances.
*/
private com.amazonaws.internal.ListWithAutoConstructFlag instanceIds;
/**
* One or more filters. -
architecture
- The
* instance architecture (i386
| x86_64
).
* -
availability-zone
- The Availability Zone of the
* instance.
-
block-device-mapping.attach-time
* - The attach time for an Amazon EBS volume mapped to the instance.
*
-
block-device-mapping.delete-on-termination
* - A Boolean that indicates whether the Amazon EBS volume is deleted on
* instance termination.
-
*
block-device-mapping.device-name
- The device name for
* the Amazon EBS volume (for example, /dev/sdh
).
-
*
block-device-mapping.status
- The status for the
* Amazon EBS volume (attaching
| attached
|
* detaching
| detached
).
-
*
block-device-mapping.volume-id
- The volume ID of the
* Amazon EBS volume.
-
client-token
- The
* idempotency token you provided when you launched the instance.
* -
dns-name
- The public DNS name of the instance.
*
-
group-id
- The ID of the security group for
* the instance. If the instance is in EC2-Classic or a default VPC, you
* can use group-name
instead.
-
*
group-name
- The name of the security group for the
* instance. If the instance is in a nondefault VPC, you must use
* group-id
instead.
-
hypervisor
-
* The hypervisor type of the instance (ovm
|
* xen
).
-
image-id
- The ID of the
* image used to launch the instance.
-
*
instance-id
- The ID of the instance.
-
*
instance-lifecycle
- Indicates whether this is a Spot
* Instance (spot
).
-
*
instance-state-code
- The state of the instance, as a
* 16-bit unsigned integer. The high byte is an opaque internal value and
* should be ignored. The low byte is set based on the state represented.
* The valid values are: 0 (pending), 16 (running), 32 (shutting-down),
* 48 (terminated), 64 (stopping), and 80 (stopped).
-
*
instance-state-name
- The state of the instance
* (pending
| running
|
* shutting-down
| terminated
|
* stopping
| stopped
).
-
*
instance-type
- The type of instance (for example,
* m1.small
).
-
instance.group-id
-
* The ID of the security group for the instance. If the instance is in
* EC2-Classic or a default VPC, you can use
* instance.group-name
instead.
-
*
instance.group-name
- The name of the security group
* for the instance. If the instance is in a nondefault VPC, you must use
* instance.group-id
instead.
-
*
ip-address
- The public IP address of the instance.
*
-
kernel-id
- The kernel ID.
-
*
key-name
- The name of the key pair used when the
* instance was launched.
-
launch-index
- When
* launching multiple instances, this is the index for the instance in
* the launch group (for example, 0, 1, 2, and so on).
-
*
launch-time
- The time when the instance was launched.
*
-
monitoring-state
- Indicates whether
* monitoring is enabled for the instance (disabled
|
* enabled
).
-
owner-id
- The AWS
* account ID of the instance owner.
-
*
placement-group-name
- The name of the placement group
* for the instance.
-
platform
- The platform.
* Use windows
if you have Windows instances; otherwise,
* leave blank.
-
private-dns-name
- The private
* DNS name of the instance.
-
*
private-ip-address
- The private IP address of the
* instance.
-
product-code
- The product code
* associated with the AMI used to launch the instance.
-
*
product-code.type
- The type of product code
* (devpay
| marketplace
).
-
*
ramdisk-id
- The RAM disk ID.
-
*
reason
- The reason for the current state of the
* instance (for example, shows "User Initiated [date]" when you stop or
* terminate the instance). Similar to the state-reason-code filter.
*
-
requester-id
- The ID of the entity that
* launched the instance on your behalf (for example, AWS Management
* Console, Auto Scaling, and so on).
-
*
reservation-id
- The ID of the instance's reservation.
* A reservation ID is created any time you launch an instance. A
* reservation ID has a one-to-one relationship with an instance launch
* request, but can be associated with more than one instance if you
* launch multiple instances using the same launch request. For example,
* if you launch one instance, you'll get one reservation ID. If you
* launch ten instances using the same launch request, you'll also get
* one reservation ID.
-
root-device-name
- The
* name of the root device for the instance (for example,
* /dev/sda1
).
-
root-device-type
-
* The type of root device that the instance uses (ebs
|
* instance-store
).
-
*
source-dest-check
- Indicates whether the instance
* performs source/destination checking. A value of true
* means that checking is enabled, and false
means checking
* is disabled. The value must be false
for the instance to
* perform network address translation (NAT) in your VPC.
-
*
spot-instance-request-id
- The ID of the Spot Instance
* request.
-
state-reason-code
- The reason
* code for the state change.
-
*
state-reason-message
- A message that describes the
* state change.
-
subnet-id
- The ID of the
* subnet for the instance.
-
*
tag
:key=value - The key/value
* combination of a tag assigned to the resource, where
* tag
:key is the tag's key.
-
*
tag-key
- The key of a tag assigned to the resource.
* This filter is independent of the tag-value
filter. For
* example, if you use both the filter "tag-key=Purpose" and the filter
* "tag-value=X", you get any resources assigned both the tag key Purpose
* (regardless of what the tag's value is), and the tag value X
* (regardless of what the tag's key is). If you want to list only
* resources where Purpose is X, see the
* tag
:key=value filter.
-
*
tag-value
- The value of a tag assigned to the
* resource. This filter is independent of the tag-key
* filter.
-
virtualization-type
- The
* virtualization type of the instance (paravirtual
|
* hvm
).
-
vpc-id
- The ID of the
* VPC that the instance is running in.
-
*
network-interface.description
- The description of the
* network interface.
-
*
network-interface.subnet-id
- The ID of the subnet for
* the network interface.
-
*
network-interface.vpc-id
- The ID of the VPC for the
* network interface.
-
*
network-interface.network-interface.id
- The ID of the
* network interface.
-
*
network-interface.owner-id
- The ID of the owner of
* the network interface.
-
*
network-interface.availability-zone
- The Availability
* Zone for the network interface.
-
*
network-interface.requester-id
- The requester ID for
* the network interface.
-
*
network-interface.requester-managed
- Indicates
* whether the network interface is being managed by AWS.
-
*
network-interface.status
- The status of the network
* interface (available
) | in-use
).
-
*
network-interface.mac-address
- The MAC address of the
* network interface.
-
*
network-interface-private-dns-name
- The private DNS
* name of the network interface.
-
*
network-interface.source-destination-check
- Whether
* the network interface performs source/destination checking. A value of
* true
means checking is enabled, and false
* means checking is disabled. The value must be false
for
* the network interface to perform network address translation (NAT) in
* your VPC.
-
network-interface.group-id
- The
* ID of a security group associated with the network interface.
* -
network-interface.group-name
- The name of a
* security group associated with the network interface.
-
*
network-interface.attachment.attachment-id
- The ID of
* the interface attachment.
-
*
network-interface.attachment.instance-id
- The ID of
* the instance to which the network interface is attached.
-
*
network-interface.attachment.instance-owner-id
- The
* owner ID of the instance to which the network interface is attached.
*
-
*
network-interface.addresses.private-ip-address
- The
* private IP address associated with the network interface.
-
*
network-interface.attachment.device-index
- The device
* index to which the network interface is attached.
-
*
network-interface.attachment.status
- The status of
* the attachment (attaching
| attached
|
* detaching
| detached
).
-
*
network-interface.attachment.attach-time
- The time
* that the network interface was attached to an instance.
-
*
network-interface.attachment.delete-on-termination
-
* Specifies whether the attachment is deleted when an instance is
* terminated.
-
*
network-interface.addresses.primary
- Specifies
* whether the IP address of the network interface is the primary private
* IP address.
-
*
network-interface.addresses.association.public-ip
-
* The ID of the association of an Elastic IP address with a network
* interface.
-
*
network-interface.addresses.association.ip-owner-id
-
* The owner ID of the private IP address associated with the network
* interface.
-
association.public-ip
- The
* address of the Elastic IP address bound to the network interface.
*
-
association.ip-owner-id
- The owner of the
* Elastic IP address associated with the network interface.
-
*
association.allocation-id
- The allocation ID returned
* when you allocated the Elastic IP address for your network interface.
*
-
association.association-id
- The
* association ID returned when the network interface was associated with
* an IP address.
*/
private com.amazonaws.internal.ListWithAutoConstructFlag filters;
/**
* The token for the next set of items to return. (You received this
* token from a prior call.)
*/
private String nextToken;
/**
* The maximum number of items to return for this call. The call also
* returns a token that you can specify in a subsequent call to get the
* next set of results. If the value is greater than 1000, we return only
* 1000 items.
*/
private Integer maxResults;
/**
* One or more instance IDs. Default: Describes all your instances.
*
* @return One or more instance IDs.
Default: Describes all your instances.
*/
public java.util.List getInstanceIds() {
if (instanceIds == null) {
instanceIds = new com.amazonaws.internal.ListWithAutoConstructFlag();
instanceIds.setAutoConstruct(true);
}
return instanceIds;
}
/**
* One or more instance IDs. Default: Describes all your instances.
*
* @param instanceIds One or more instance IDs.
Default: Describes all your instances.
*/
public void setInstanceIds(java.util.Collection instanceIds) {
if (instanceIds == null) {
this.instanceIds = null;
return;
}
com.amazonaws.internal.ListWithAutoConstructFlag instanceIdsCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(instanceIds.size());
instanceIdsCopy.addAll(instanceIds);
this.instanceIds = instanceIdsCopy;
}
/**
* One or more instance IDs. Default: Describes all your instances.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param instanceIds One or more instance IDs.
Default: Describes all your instances.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public DescribeInstancesRequest withInstanceIds(String... instanceIds) {
if (getInstanceIds() == null) setInstanceIds(new java.util.ArrayList(instanceIds.length));
for (String value : instanceIds) {
getInstanceIds().add(value);
}
return this;
}
/**
* One or more instance IDs. Default: Describes all your instances.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param instanceIds One or more instance IDs.
Default: Describes all your instances.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public DescribeInstancesRequest withInstanceIds(java.util.Collection instanceIds) {
if (instanceIds == null) {
this.instanceIds = null;
} else {
com.amazonaws.internal.ListWithAutoConstructFlag instanceIdsCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(instanceIds.size());
instanceIdsCopy.addAll(instanceIds);
this.instanceIds = instanceIdsCopy;
}
return this;
}
/**
* One or more filters. -
architecture
- The
* instance architecture (i386
| x86_64
).
* -
availability-zone
- The Availability Zone of the
* instance.
-
block-device-mapping.attach-time
* - The attach time for an Amazon EBS volume mapped to the instance.
*
-
block-device-mapping.delete-on-termination
* - A Boolean that indicates whether the Amazon EBS volume is deleted on
* instance termination.
-
*
block-device-mapping.device-name
- The device name for
* the Amazon EBS volume (for example, /dev/sdh
).
-
*
block-device-mapping.status
- The status for the
* Amazon EBS volume (attaching
| attached
|
* detaching
| detached
).
-
*
block-device-mapping.volume-id
- The volume ID of the
* Amazon EBS volume.
-
client-token
- The
* idempotency token you provided when you launched the instance.
* -
dns-name
- The public DNS name of the instance.
*
-
group-id
- The ID of the security group for
* the instance. If the instance is in EC2-Classic or a default VPC, you
* can use group-name
instead.
-
*
group-name
- The name of the security group for the
* instance. If the instance is in a nondefault VPC, you must use
* group-id
instead.
-
hypervisor
-
* The hypervisor type of the instance (ovm
|
* xen
).
-
image-id
- The ID of the
* image used to launch the instance.
-
*
instance-id
- The ID of the instance.
-
*
instance-lifecycle
- Indicates whether this is a Spot
* Instance (spot
).
-
*
instance-state-code
- The state of the instance, as a
* 16-bit unsigned integer. The high byte is an opaque internal value and
* should be ignored. The low byte is set based on the state represented.
* The valid values are: 0 (pending), 16 (running), 32 (shutting-down),
* 48 (terminated), 64 (stopping), and 80 (stopped).
-
*
instance-state-name
- The state of the instance
* (pending
| running
|
* shutting-down
| terminated
|
* stopping
| stopped
).
-
*
instance-type
- The type of instance (for example,
* m1.small
).
-
instance.group-id
-
* The ID of the security group for the instance. If the instance is in
* EC2-Classic or a default VPC, you can use
* instance.group-name
instead.
-
*
instance.group-name
- The name of the security group
* for the instance. If the instance is in a nondefault VPC, you must use
* instance.group-id
instead.
-
*
ip-address
- The public IP address of the instance.
*
-
kernel-id
- The kernel ID.
-
*
key-name
- The name of the key pair used when the
* instance was launched.
-
launch-index
- When
* launching multiple instances, this is the index for the instance in
* the launch group (for example, 0, 1, 2, and so on).
-
*
launch-time
- The time when the instance was launched.
*
-
monitoring-state
- Indicates whether
* monitoring is enabled for the instance (disabled
|
* enabled
).
-
owner-id
- The AWS
* account ID of the instance owner.
-
*
placement-group-name
- The name of the placement group
* for the instance.
-
platform
- The platform.
* Use windows
if you have Windows instances; otherwise,
* leave blank.
-
private-dns-name
- The private
* DNS name of the instance.
-
*
private-ip-address
- The private IP address of the
* instance.
-
product-code
- The product code
* associated with the AMI used to launch the instance.
-
*
product-code.type
- The type of product code
* (devpay
| marketplace
).
-
*
ramdisk-id
- The RAM disk ID.
-
*
reason
- The reason for the current state of the
* instance (for example, shows "User Initiated [date]" when you stop or
* terminate the instance). Similar to the state-reason-code filter.
*
-
requester-id
- The ID of the entity that
* launched the instance on your behalf (for example, AWS Management
* Console, Auto Scaling, and so on).
-
*
reservation-id
- The ID of the instance's reservation.
* A reservation ID is created any time you launch an instance. A
* reservation ID has a one-to-one relationship with an instance launch
* request, but can be associated with more than one instance if you
* launch multiple instances using the same launch request. For example,
* if you launch one instance, you'll get one reservation ID. If you
* launch ten instances using the same launch request, you'll also get
* one reservation ID.
-
root-device-name
- The
* name of the root device for the instance (for example,
* /dev/sda1
).
-
root-device-type
-
* The type of root device that the instance uses (ebs
|
* instance-store
).
-
*
source-dest-check
- Indicates whether the instance
* performs source/destination checking. A value of true
* means that checking is enabled, and false
means checking
* is disabled. The value must be false
for the instance to
* perform network address translation (NAT) in your VPC.
-
*
spot-instance-request-id
- The ID of the Spot Instance
* request.
-
state-reason-code
- The reason
* code for the state change.
-
*
state-reason-message
- A message that describes the
* state change.
-
subnet-id
- The ID of the
* subnet for the instance.
-
*
tag
:key=value - The key/value
* combination of a tag assigned to the resource, where
* tag
:key is the tag's key.
-
*
tag-key
- The key of a tag assigned to the resource.
* This filter is independent of the tag-value
filter. For
* example, if you use both the filter "tag-key=Purpose" and the filter
* "tag-value=X", you get any resources assigned both the tag key Purpose
* (regardless of what the tag's value is), and the tag value X
* (regardless of what the tag's key is). If you want to list only
* resources where Purpose is X, see the
* tag
:key=value filter.
-
*
tag-value
- The value of a tag assigned to the
* resource. This filter is independent of the tag-key
* filter.
-
virtualization-type
- The
* virtualization type of the instance (paravirtual
|
* hvm
).
-
vpc-id
- The ID of the
* VPC that the instance is running in.
-
*
network-interface.description
- The description of the
* network interface.
-
*
network-interface.subnet-id
- The ID of the subnet for
* the network interface.
-
*
network-interface.vpc-id
- The ID of the VPC for the
* network interface.
-
*
network-interface.network-interface.id
- The ID of the
* network interface.
-
*
network-interface.owner-id
- The ID of the owner of
* the network interface.
-
*
network-interface.availability-zone
- The Availability
* Zone for the network interface.
-
*
network-interface.requester-id
- The requester ID for
* the network interface.
-
*
network-interface.requester-managed
- Indicates
* whether the network interface is being managed by AWS.
-
*
network-interface.status
- The status of the network
* interface (available
) | in-use
).
-
*
network-interface.mac-address
- The MAC address of the
* network interface.
-
*
network-interface-private-dns-name
- The private DNS
* name of the network interface.
-
*
network-interface.source-destination-check
- Whether
* the network interface performs source/destination checking. A value of
* true
means checking is enabled, and false
* means checking is disabled. The value must be false
for
* the network interface to perform network address translation (NAT) in
* your VPC.
-
network-interface.group-id
- The
* ID of a security group associated with the network interface.
* -
network-interface.group-name
- The name of a
* security group associated with the network interface.
-
*
network-interface.attachment.attachment-id
- The ID of
* the interface attachment.
-
*
network-interface.attachment.instance-id
- The ID of
* the instance to which the network interface is attached.
-
*
network-interface.attachment.instance-owner-id
- The
* owner ID of the instance to which the network interface is attached.
*
-
*
network-interface.addresses.private-ip-address
- The
* private IP address associated with the network interface.
-
*
network-interface.attachment.device-index
- The device
* index to which the network interface is attached.
-
*
network-interface.attachment.status
- The status of
* the attachment (attaching
| attached
|
* detaching
| detached
).
-
*
network-interface.attachment.attach-time
- The time
* that the network interface was attached to an instance.
-
*
network-interface.attachment.delete-on-termination
-
* Specifies whether the attachment is deleted when an instance is
* terminated.
-
*
network-interface.addresses.primary
- Specifies
* whether the IP address of the network interface is the primary private
* IP address.
-
*
network-interface.addresses.association.public-ip
-
* The ID of the association of an Elastic IP address with a network
* interface.
-
*
network-interface.addresses.association.ip-owner-id
-
* The owner ID of the private IP address associated with the network
* interface.
-
association.public-ip
- The
* address of the Elastic IP address bound to the network interface.
*
-
association.ip-owner-id
- The owner of the
* Elastic IP address associated with the network interface.
-
*
association.allocation-id
- The allocation ID returned
* when you allocated the Elastic IP address for your network interface.
*
-
association.association-id
- The
* association ID returned when the network interface was associated with
* an IP address.
*
* @return One or more filters. -
architecture
- The
* instance architecture (i386
| x86_64
).
* -
availability-zone
- The Availability Zone of the
* instance.
-
block-device-mapping.attach-time
* - The attach time for an Amazon EBS volume mapped to the instance.
*
-
block-device-mapping.delete-on-termination
* - A Boolean that indicates whether the Amazon EBS volume is deleted on
* instance termination.
-
*
block-device-mapping.device-name
- The device name for
* the Amazon EBS volume (for example, /dev/sdh
).
-
*
block-device-mapping.status
- The status for the
* Amazon EBS volume (attaching
| attached
|
* detaching
| detached
).
-
*
block-device-mapping.volume-id
- The volume ID of the
* Amazon EBS volume.
-
client-token
- The
* idempotency token you provided when you launched the instance.
* -
dns-name
- The public DNS name of the instance.
*
-
group-id
- The ID of the security group for
* the instance. If the instance is in EC2-Classic or a default VPC, you
* can use group-name
instead.
-
*
group-name
- The name of the security group for the
* instance. If the instance is in a nondefault VPC, you must use
* group-id
instead.
-
hypervisor
-
* The hypervisor type of the instance (ovm
|
* xen
).
-
image-id
- The ID of the
* image used to launch the instance.
-
*
instance-id
- The ID of the instance.
-
*
instance-lifecycle
- Indicates whether this is a Spot
* Instance (spot
).
-
*
instance-state-code
- The state of the instance, as a
* 16-bit unsigned integer. The high byte is an opaque internal value and
* should be ignored. The low byte is set based on the state represented.
* The valid values are: 0 (pending), 16 (running), 32 (shutting-down),
* 48 (terminated), 64 (stopping), and 80 (stopped).
-
*
instance-state-name
- The state of the instance
* (pending
| running
|
* shutting-down
| terminated
|
* stopping
| stopped
).
-
*
instance-type
- The type of instance (for example,
* m1.small
).
-
instance.group-id
-
* The ID of the security group for the instance. If the instance is in
* EC2-Classic or a default VPC, you can use
* instance.group-name
instead.
-
*
instance.group-name
- The name of the security group
* for the instance. If the instance is in a nondefault VPC, you must use
* instance.group-id
instead.
-
*
ip-address
- The public IP address of the instance.
*
-
kernel-id
- The kernel ID.
-
*
key-name
- The name of the key pair used when the
* instance was launched.
-
launch-index
- When
* launching multiple instances, this is the index for the instance in
* the launch group (for example, 0, 1, 2, and so on).
-
*
launch-time
- The time when the instance was launched.
*
-
monitoring-state
- Indicates whether
* monitoring is enabled for the instance (disabled
|
* enabled
).
-
owner-id
- The AWS
* account ID of the instance owner.
-
*
placement-group-name
- The name of the placement group
* for the instance.
-
platform
- The platform.
* Use windows
if you have Windows instances; otherwise,
* leave blank.
-
private-dns-name
- The private
* DNS name of the instance.
-
*
private-ip-address
- The private IP address of the
* instance.
-
product-code
- The product code
* associated with the AMI used to launch the instance.
-
*
product-code.type
- The type of product code
* (devpay
| marketplace
).
-
*
ramdisk-id
- The RAM disk ID.
-
*
reason
- The reason for the current state of the
* instance (for example, shows "User Initiated [date]" when you stop or
* terminate the instance). Similar to the state-reason-code filter.
*
-
requester-id
- The ID of the entity that
* launched the instance on your behalf (for example, AWS Management
* Console, Auto Scaling, and so on).
-
*
reservation-id
- The ID of the instance's reservation.
* A reservation ID is created any time you launch an instance. A
* reservation ID has a one-to-one relationship with an instance launch
* request, but can be associated with more than one instance if you
* launch multiple instances using the same launch request. For example,
* if you launch one instance, you'll get one reservation ID. If you
* launch ten instances using the same launch request, you'll also get
* one reservation ID.
-
root-device-name
- The
* name of the root device for the instance (for example,
* /dev/sda1
).
-
root-device-type
-
* The type of root device that the instance uses (ebs
|
* instance-store
).
-
*
source-dest-check
- Indicates whether the instance
* performs source/destination checking. A value of true
* means that checking is enabled, and false
means checking
* is disabled. The value must be false
for the instance to
* perform network address translation (NAT) in your VPC.
-
*
spot-instance-request-id
- The ID of the Spot Instance
* request.
-
state-reason-code
- The reason
* code for the state change.
-
*
state-reason-message
- A message that describes the
* state change.
-
subnet-id
- The ID of the
* subnet for the instance.
-
*
tag
:key=value - The key/value
* combination of a tag assigned to the resource, where
* tag
:key is the tag's key.
-
*
tag-key
- The key of a tag assigned to the resource.
* This filter is independent of the tag-value
filter. For
* example, if you use both the filter "tag-key=Purpose" and the filter
* "tag-value=X", you get any resources assigned both the tag key Purpose
* (regardless of what the tag's value is), and the tag value X
* (regardless of what the tag's key is). If you want to list only
* resources where Purpose is X, see the
* tag
:key=value filter.
-
*
tag-value
- The value of a tag assigned to the
* resource. This filter is independent of the tag-key
* filter.
-
virtualization-type
- The
* virtualization type of the instance (paravirtual
|
* hvm
).
-
vpc-id
- The ID of the
* VPC that the instance is running in.
-
*
network-interface.description
- The description of the
* network interface.
-
*
network-interface.subnet-id
- The ID of the subnet for
* the network interface.
-
*
network-interface.vpc-id
- The ID of the VPC for the
* network interface.
-
*
network-interface.network-interface.id
- The ID of the
* network interface.
-
*
network-interface.owner-id
- The ID of the owner of
* the network interface.
-
*
network-interface.availability-zone
- The Availability
* Zone for the network interface.
-
*
network-interface.requester-id
- The requester ID for
* the network interface.
-
*
network-interface.requester-managed
- Indicates
* whether the network interface is being managed by AWS.
-
*
network-interface.status
- The status of the network
* interface (available
) | in-use
).
-
*
network-interface.mac-address
- The MAC address of the
* network interface.
-
*
network-interface-private-dns-name
- The private DNS
* name of the network interface.
-
*
network-interface.source-destination-check
- Whether
* the network interface performs source/destination checking. A value of
* true
means checking is enabled, and false
* means checking is disabled. The value must be false
for
* the network interface to perform network address translation (NAT) in
* your VPC.
-
network-interface.group-id
- The
* ID of a security group associated with the network interface.
* -
network-interface.group-name
- The name of a
* security group associated with the network interface.
-
*
network-interface.attachment.attachment-id
- The ID of
* the interface attachment.
-
*
network-interface.attachment.instance-id
- The ID of
* the instance to which the network interface is attached.
-
*
network-interface.attachment.instance-owner-id
- The
* owner ID of the instance to which the network interface is attached.
*
-
*
network-interface.addresses.private-ip-address
- The
* private IP address associated with the network interface.
-
*
network-interface.attachment.device-index
- The device
* index to which the network interface is attached.
-
*
network-interface.attachment.status
- The status of
* the attachment (attaching
| attached
|
* detaching
| detached
).
-
*
network-interface.attachment.attach-time
- The time
* that the network interface was attached to an instance.
-
*
network-interface.attachment.delete-on-termination
-
* Specifies whether the attachment is deleted when an instance is
* terminated.
-
*
network-interface.addresses.primary
- Specifies
* whether the IP address of the network interface is the primary private
* IP address.
-
*
network-interface.addresses.association.public-ip
-
* The ID of the association of an Elastic IP address with a network
* interface.
-
*
network-interface.addresses.association.ip-owner-id
-
* The owner ID of the private IP address associated with the network
* interface.
-
association.public-ip
- The
* address of the Elastic IP address bound to the network interface.
*
-
association.ip-owner-id
- The owner of the
* Elastic IP address associated with the network interface.
-
*
association.allocation-id
- The allocation ID returned
* when you allocated the Elastic IP address for your network interface.
*
-
association.association-id
- The
* association ID returned when the network interface was associated with
* an IP address.
*/
public java.util.List getFilters() {
if (filters == null) {
filters = new com.amazonaws.internal.ListWithAutoConstructFlag();
filters.setAutoConstruct(true);
}
return filters;
}
/**
* One or more filters. -
architecture
- The
* instance architecture (i386
| x86_64
).
* -
availability-zone
- The Availability Zone of the
* instance.
-
block-device-mapping.attach-time
* - The attach time for an Amazon EBS volume mapped to the instance.
*
-
block-device-mapping.delete-on-termination
* - A Boolean that indicates whether the Amazon EBS volume is deleted on
* instance termination.
-
*
block-device-mapping.device-name
- The device name for
* the Amazon EBS volume (for example, /dev/sdh
).
-
*
block-device-mapping.status
- The status for the
* Amazon EBS volume (attaching
| attached
|
* detaching
| detached
).
-
*
block-device-mapping.volume-id
- The volume ID of the
* Amazon EBS volume.
-
client-token
- The
* idempotency token you provided when you launched the instance.
* -
dns-name
- The public DNS name of the instance.
*
-
group-id
- The ID of the security group for
* the instance. If the instance is in EC2-Classic or a default VPC, you
* can use group-name
instead.
-
*
group-name
- The name of the security group for the
* instance. If the instance is in a nondefault VPC, you must use
* group-id
instead.
-
hypervisor
-
* The hypervisor type of the instance (ovm
|
* xen
).
-
image-id
- The ID of the
* image used to launch the instance.
-
*
instance-id
- The ID of the instance.
-
*
instance-lifecycle
- Indicates whether this is a Spot
* Instance (spot
).
-
*
instance-state-code
- The state of the instance, as a
* 16-bit unsigned integer. The high byte is an opaque internal value and
* should be ignored. The low byte is set based on the state represented.
* The valid values are: 0 (pending), 16 (running), 32 (shutting-down),
* 48 (terminated), 64 (stopping), and 80 (stopped).
-
*
instance-state-name
- The state of the instance
* (pending
| running
|
* shutting-down
| terminated
|
* stopping
| stopped
).
-
*
instance-type
- The type of instance (for example,
* m1.small
).
-
instance.group-id
-
* The ID of the security group for the instance. If the instance is in
* EC2-Classic or a default VPC, you can use
* instance.group-name
instead.
-
*
instance.group-name
- The name of the security group
* for the instance. If the instance is in a nondefault VPC, you must use
* instance.group-id
instead.
-
*
ip-address
- The public IP address of the instance.
*
-
kernel-id
- The kernel ID.
-
*
key-name
- The name of the key pair used when the
* instance was launched.
-
launch-index
- When
* launching multiple instances, this is the index for the instance in
* the launch group (for example, 0, 1, 2, and so on).
-
*
launch-time
- The time when the instance was launched.
*
-
monitoring-state
- Indicates whether
* monitoring is enabled for the instance (disabled
|
* enabled
).
-
owner-id
- The AWS
* account ID of the instance owner.
-
*
placement-group-name
- The name of the placement group
* for the instance.
-
platform
- The platform.
* Use windows
if you have Windows instances; otherwise,
* leave blank.
-
private-dns-name
- The private
* DNS name of the instance.
-
*
private-ip-address
- The private IP address of the
* instance.
-
product-code
- The product code
* associated with the AMI used to launch the instance.
-
*
product-code.type
- The type of product code
* (devpay
| marketplace
).
-
*
ramdisk-id
- The RAM disk ID.
-
*
reason
- The reason for the current state of the
* instance (for example, shows "User Initiated [date]" when you stop or
* terminate the instance). Similar to the state-reason-code filter.
*
-
requester-id
- The ID of the entity that
* launched the instance on your behalf (for example, AWS Management
* Console, Auto Scaling, and so on).
-
*
reservation-id
- The ID of the instance's reservation.
* A reservation ID is created any time you launch an instance. A
* reservation ID has a one-to-one relationship with an instance launch
* request, but can be associated with more than one instance if you
* launch multiple instances using the same launch request. For example,
* if you launch one instance, you'll get one reservation ID. If you
* launch ten instances using the same launch request, you'll also get
* one reservation ID.
-
root-device-name
- The
* name of the root device for the instance (for example,
* /dev/sda1
).
-
root-device-type
-
* The type of root device that the instance uses (ebs
|
* instance-store
).
-
*
source-dest-check
- Indicates whether the instance
* performs source/destination checking. A value of true
* means that checking is enabled, and false
means checking
* is disabled. The value must be false
for the instance to
* perform network address translation (NAT) in your VPC.
-
*
spot-instance-request-id
- The ID of the Spot Instance
* request.
-
state-reason-code
- The reason
* code for the state change.
-
*
state-reason-message
- A message that describes the
* state change.
-
subnet-id
- The ID of the
* subnet for the instance.
-
*
tag
:key=value - The key/value
* combination of a tag assigned to the resource, where
* tag
:key is the tag's key.
-
*
tag-key
- The key of a tag assigned to the resource.
* This filter is independent of the tag-value
filter. For
* example, if you use both the filter "tag-key=Purpose" and the filter
* "tag-value=X", you get any resources assigned both the tag key Purpose
* (regardless of what the tag's value is), and the tag value X
* (regardless of what the tag's key is). If you want to list only
* resources where Purpose is X, see the
* tag
:key=value filter.
-
*
tag-value
- The value of a tag assigned to the
* resource. This filter is independent of the tag-key
* filter.
-
virtualization-type
- The
* virtualization type of the instance (paravirtual
|
* hvm
).
-
vpc-id
- The ID of the
* VPC that the instance is running in.
-
*
network-interface.description
- The description of the
* network interface.
-
*
network-interface.subnet-id
- The ID of the subnet for
* the network interface.
-
*
network-interface.vpc-id
- The ID of the VPC for the
* network interface.
-
*
network-interface.network-interface.id
- The ID of the
* network interface.
-
*
network-interface.owner-id
- The ID of the owner of
* the network interface.
-
*
network-interface.availability-zone
- The Availability
* Zone for the network interface.
-
*
network-interface.requester-id
- The requester ID for
* the network interface.
-
*
network-interface.requester-managed
- Indicates
* whether the network interface is being managed by AWS.
-
*
network-interface.status
- The status of the network
* interface (available
) | in-use
).
-
*
network-interface.mac-address
- The MAC address of the
* network interface.
-
*
network-interface-private-dns-name
- The private DNS
* name of the network interface.
-
*
network-interface.source-destination-check
- Whether
* the network interface performs source/destination checking. A value of
* true
means checking is enabled, and false
* means checking is disabled. The value must be false
for
* the network interface to perform network address translation (NAT) in
* your VPC.
-
network-interface.group-id
- The
* ID of a security group associated with the network interface.
* -
network-interface.group-name
- The name of a
* security group associated with the network interface.
-
*
network-interface.attachment.attachment-id
- The ID of
* the interface attachment.
-
*
network-interface.attachment.instance-id
- The ID of
* the instance to which the network interface is attached.
-
*
network-interface.attachment.instance-owner-id
- The
* owner ID of the instance to which the network interface is attached.
*
-
*
network-interface.addresses.private-ip-address
- The
* private IP address associated with the network interface.
-
*
network-interface.attachment.device-index
- The device
* index to which the network interface is attached.
-
*
network-interface.attachment.status
- The status of
* the attachment (attaching
| attached
|
* detaching
| detached
).
-
*
network-interface.attachment.attach-time
- The time
* that the network interface was attached to an instance.
-
*
network-interface.attachment.delete-on-termination
-
* Specifies whether the attachment is deleted when an instance is
* terminated.
-
*
network-interface.addresses.primary
- Specifies
* whether the IP address of the network interface is the primary private
* IP address.
-
*
network-interface.addresses.association.public-ip
-
* The ID of the association of an Elastic IP address with a network
* interface.
-
*
network-interface.addresses.association.ip-owner-id
-
* The owner ID of the private IP address associated with the network
* interface.
-
association.public-ip
- The
* address of the Elastic IP address bound to the network interface.
*
-
association.ip-owner-id
- The owner of the
* Elastic IP address associated with the network interface.
-
*
association.allocation-id
- The allocation ID returned
* when you allocated the Elastic IP address for your network interface.
*
-
association.association-id
- The
* association ID returned when the network interface was associated with
* an IP address.
*
* @param filters One or more filters. -
architecture
- The
* instance architecture (i386
| x86_64
).
* -
availability-zone
- The Availability Zone of the
* instance.
-
block-device-mapping.attach-time
* - The attach time for an Amazon EBS volume mapped to the instance.
*
-
block-device-mapping.delete-on-termination
* - A Boolean that indicates whether the Amazon EBS volume is deleted on
* instance termination.
-
*
block-device-mapping.device-name
- The device name for
* the Amazon EBS volume (for example, /dev/sdh
).
-
*
block-device-mapping.status
- The status for the
* Amazon EBS volume (attaching
| attached
|
* detaching
| detached
).
-
*
block-device-mapping.volume-id
- The volume ID of the
* Amazon EBS volume.
-
client-token
- The
* idempotency token you provided when you launched the instance.
* -
dns-name
- The public DNS name of the instance.
*
-
group-id
- The ID of the security group for
* the instance. If the instance is in EC2-Classic or a default VPC, you
* can use group-name
instead.
-
*
group-name
- The name of the security group for the
* instance. If the instance is in a nondefault VPC, you must use
* group-id
instead.
-
hypervisor
-
* The hypervisor type of the instance (ovm
|
* xen
).
-
image-id
- The ID of the
* image used to launch the instance.
-
*
instance-id
- The ID of the instance.
-
*
instance-lifecycle
- Indicates whether this is a Spot
* Instance (spot
).
-
*
instance-state-code
- The state of the instance, as a
* 16-bit unsigned integer. The high byte is an opaque internal value and
* should be ignored. The low byte is set based on the state represented.
* The valid values are: 0 (pending), 16 (running), 32 (shutting-down),
* 48 (terminated), 64 (stopping), and 80 (stopped).
-
*
instance-state-name
- The state of the instance
* (pending
| running
|
* shutting-down
| terminated
|
* stopping
| stopped
).
-
*
instance-type
- The type of instance (for example,
* m1.small
).
-
instance.group-id
-
* The ID of the security group for the instance. If the instance is in
* EC2-Classic or a default VPC, you can use
* instance.group-name
instead.
-
*
instance.group-name
- The name of the security group
* for the instance. If the instance is in a nondefault VPC, you must use
* instance.group-id
instead.
-
*
ip-address
- The public IP address of the instance.
*
-
kernel-id
- The kernel ID.
-
*
key-name
- The name of the key pair used when the
* instance was launched.
-
launch-index
- When
* launching multiple instances, this is the index for the instance in
* the launch group (for example, 0, 1, 2, and so on).
-
*
launch-time
- The time when the instance was launched.
*
-
monitoring-state
- Indicates whether
* monitoring is enabled for the instance (disabled
|
* enabled
).
-
owner-id
- The AWS
* account ID of the instance owner.
-
*
placement-group-name
- The name of the placement group
* for the instance.
-
platform
- The platform.
* Use windows
if you have Windows instances; otherwise,
* leave blank.
-
private-dns-name
- The private
* DNS name of the instance.
-
*
private-ip-address
- The private IP address of the
* instance.
-
product-code
- The product code
* associated with the AMI used to launch the instance.
-
*
product-code.type
- The type of product code
* (devpay
| marketplace
).
-
*
ramdisk-id
- The RAM disk ID.
-
*
reason
- The reason for the current state of the
* instance (for example, shows "User Initiated [date]" when you stop or
* terminate the instance). Similar to the state-reason-code filter.
*
-
requester-id
- The ID of the entity that
* launched the instance on your behalf (for example, AWS Management
* Console, Auto Scaling, and so on).
-
*
reservation-id
- The ID of the instance's reservation.
* A reservation ID is created any time you launch an instance. A
* reservation ID has a one-to-one relationship with an instance launch
* request, but can be associated with more than one instance if you
* launch multiple instances using the same launch request. For example,
* if you launch one instance, you'll get one reservation ID. If you
* launch ten instances using the same launch request, you'll also get
* one reservation ID.
-
root-device-name
- The
* name of the root device for the instance (for example,
* /dev/sda1
).
-
root-device-type
-
* The type of root device that the instance uses (ebs
|
* instance-store
).
-
*
source-dest-check
- Indicates whether the instance
* performs source/destination checking. A value of true
* means that checking is enabled, and false
means checking
* is disabled. The value must be false
for the instance to
* perform network address translation (NAT) in your VPC.
-
*
spot-instance-request-id
- The ID of the Spot Instance
* request.
-
state-reason-code
- The reason
* code for the state change.
-
*
state-reason-message
- A message that describes the
* state change.
-
subnet-id
- The ID of the
* subnet for the instance.
-
*
tag
:key=value - The key/value
* combination of a tag assigned to the resource, where
* tag
:key is the tag's key.
-
*
tag-key
- The key of a tag assigned to the resource.
* This filter is independent of the tag-value
filter. For
* example, if you use both the filter "tag-key=Purpose" and the filter
* "tag-value=X", you get any resources assigned both the tag key Purpose
* (regardless of what the tag's value is), and the tag value X
* (regardless of what the tag's key is). If you want to list only
* resources where Purpose is X, see the
* tag
:key=value filter.
-
*
tag-value
- The value of a tag assigned to the
* resource. This filter is independent of the tag-key
* filter.
-
virtualization-type
- The
* virtualization type of the instance (paravirtual
|
* hvm
).
-
vpc-id
- The ID of the
* VPC that the instance is running in.
-
*
network-interface.description
- The description of the
* network interface.
-
*
network-interface.subnet-id
- The ID of the subnet for
* the network interface.
-
*
network-interface.vpc-id
- The ID of the VPC for the
* network interface.
-
*
network-interface.network-interface.id
- The ID of the
* network interface.
-
*
network-interface.owner-id
- The ID of the owner of
* the network interface.
-
*
network-interface.availability-zone
- The Availability
* Zone for the network interface.
-
*
network-interface.requester-id
- The requester ID for
* the network interface.
-
*
network-interface.requester-managed
- Indicates
* whether the network interface is being managed by AWS.
-
*
network-interface.status
- The status of the network
* interface (available
) | in-use
).
-
*
network-interface.mac-address
- The MAC address of the
* network interface.
-
*
network-interface-private-dns-name
- The private DNS
* name of the network interface.
-
*
network-interface.source-destination-check
- Whether
* the network interface performs source/destination checking. A value of
* true
means checking is enabled, and false
* means checking is disabled. The value must be false
for
* the network interface to perform network address translation (NAT) in
* your VPC.
-
network-interface.group-id
- The
* ID of a security group associated with the network interface.
* -
network-interface.group-name
- The name of a
* security group associated with the network interface.
-
*
network-interface.attachment.attachment-id
- The ID of
* the interface attachment.
-
*
network-interface.attachment.instance-id
- The ID of
* the instance to which the network interface is attached.
-
*
network-interface.attachment.instance-owner-id
- The
* owner ID of the instance to which the network interface is attached.
*
-
*
network-interface.addresses.private-ip-address
- The
* private IP address associated with the network interface.
-
*
network-interface.attachment.device-index
- The device
* index to which the network interface is attached.
-
*
network-interface.attachment.status
- The status of
* the attachment (attaching
| attached
|
* detaching
| detached
).
-
*
network-interface.attachment.attach-time
- The time
* that the network interface was attached to an instance.
-
*
network-interface.attachment.delete-on-termination
-
* Specifies whether the attachment is deleted when an instance is
* terminated.
-
*
network-interface.addresses.primary
- Specifies
* whether the IP address of the network interface is the primary private
* IP address.
-
*
network-interface.addresses.association.public-ip
-
* The ID of the association of an Elastic IP address with a network
* interface.
-
*
network-interface.addresses.association.ip-owner-id
-
* The owner ID of the private IP address associated with the network
* interface.
-
association.public-ip
- The
* address of the Elastic IP address bound to the network interface.
*
-
association.ip-owner-id
- The owner of the
* Elastic IP address associated with the network interface.
-
*
association.allocation-id
- The allocation ID returned
* when you allocated the Elastic IP address for your network interface.
*
-
association.association-id
- The
* association ID returned when the network interface was associated with
* an IP address.
*/
public void setFilters(java.util.Collection filters) {
if (filters == null) {
this.filters = null;
return;
}
com.amazonaws.internal.ListWithAutoConstructFlag filtersCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(filters.size());
filtersCopy.addAll(filters);
this.filters = filtersCopy;
}
/**
* One or more filters. -
architecture
- The
* instance architecture (i386
| x86_64
).
* -
availability-zone
- The Availability Zone of the
* instance.
-
block-device-mapping.attach-time
* - The attach time for an Amazon EBS volume mapped to the instance.
*
-
block-device-mapping.delete-on-termination
* - A Boolean that indicates whether the Amazon EBS volume is deleted on
* instance termination.
-
*
block-device-mapping.device-name
- The device name for
* the Amazon EBS volume (for example, /dev/sdh
).
-
*
block-device-mapping.status
- The status for the
* Amazon EBS volume (attaching
| attached
|
* detaching
| detached
).
-
*
block-device-mapping.volume-id
- The volume ID of the
* Amazon EBS volume.
-
client-token
- The
* idempotency token you provided when you launched the instance.
* -
dns-name
- The public DNS name of the instance.
*
-
group-id
- The ID of the security group for
* the instance. If the instance is in EC2-Classic or a default VPC, you
* can use group-name
instead.
-
*
group-name
- The name of the security group for the
* instance. If the instance is in a nondefault VPC, you must use
* group-id
instead.
-
hypervisor
-
* The hypervisor type of the instance (ovm
|
* xen
).
-
image-id
- The ID of the
* image used to launch the instance.
-
*
instance-id
- The ID of the instance.
-
*
instance-lifecycle
- Indicates whether this is a Spot
* Instance (spot
).
-
*
instance-state-code
- The state of the instance, as a
* 16-bit unsigned integer. The high byte is an opaque internal value and
* should be ignored. The low byte is set based on the state represented.
* The valid values are: 0 (pending), 16 (running), 32 (shutting-down),
* 48 (terminated), 64 (stopping), and 80 (stopped).
-
*
instance-state-name
- The state of the instance
* (pending
| running
|
* shutting-down
| terminated
|
* stopping
| stopped
).
-
*
instance-type
- The type of instance (for example,
* m1.small
).
-
instance.group-id
-
* The ID of the security group for the instance. If the instance is in
* EC2-Classic or a default VPC, you can use
* instance.group-name
instead.
-
*
instance.group-name
- The name of the security group
* for the instance. If the instance is in a nondefault VPC, you must use
* instance.group-id
instead.
-
*
ip-address
- The public IP address of the instance.
*
-
kernel-id
- The kernel ID.
-
*
key-name
- The name of the key pair used when the
* instance was launched.
-
launch-index
- When
* launching multiple instances, this is the index for the instance in
* the launch group (for example, 0, 1, 2, and so on).
-
*
launch-time
- The time when the instance was launched.
*
-
monitoring-state
- Indicates whether
* monitoring is enabled for the instance (disabled
|
* enabled
).
-
owner-id
- The AWS
* account ID of the instance owner.
-
*
placement-group-name
- The name of the placement group
* for the instance.
-
platform
- The platform.
* Use windows
if you have Windows instances; otherwise,
* leave blank.
-
private-dns-name
- The private
* DNS name of the instance.
-
*
private-ip-address
- The private IP address of the
* instance.
-
product-code
- The product code
* associated with the AMI used to launch the instance.
-
*
product-code.type
- The type of product code
* (devpay
| marketplace
).
-
*
ramdisk-id
- The RAM disk ID.
-
*
reason
- The reason for the current state of the
* instance (for example, shows "User Initiated [date]" when you stop or
* terminate the instance). Similar to the state-reason-code filter.
*
-
requester-id
- The ID of the entity that
* launched the instance on your behalf (for example, AWS Management
* Console, Auto Scaling, and so on).
-
*
reservation-id
- The ID of the instance's reservation.
* A reservation ID is created any time you launch an instance. A
* reservation ID has a one-to-one relationship with an instance launch
* request, but can be associated with more than one instance if you
* launch multiple instances using the same launch request. For example,
* if you launch one instance, you'll get one reservation ID. If you
* launch ten instances using the same launch request, you'll also get
* one reservation ID.
-
root-device-name
- The
* name of the root device for the instance (for example,
* /dev/sda1
).
-
root-device-type
-
* The type of root device that the instance uses (ebs
|
* instance-store
).
-
*
source-dest-check
- Indicates whether the instance
* performs source/destination checking. A value of true
* means that checking is enabled, and false
means checking
* is disabled. The value must be false
for the instance to
* perform network address translation (NAT) in your VPC.
-
*
spot-instance-request-id
- The ID of the Spot Instance
* request.
-
state-reason-code
- The reason
* code for the state change.
-
*
state-reason-message
- A message that describes the
* state change.
-
subnet-id
- The ID of the
* subnet for the instance.
-
*
tag
:key=value - The key/value
* combination of a tag assigned to the resource, where
* tag
:key is the tag's key.
-
*
tag-key
- The key of a tag assigned to the resource.
* This filter is independent of the tag-value
filter. For
* example, if you use both the filter "tag-key=Purpose" and the filter
* "tag-value=X", you get any resources assigned both the tag key Purpose
* (regardless of what the tag's value is), and the tag value X
* (regardless of what the tag's key is). If you want to list only
* resources where Purpose is X, see the
* tag
:key=value filter.
-
*
tag-value
- The value of a tag assigned to the
* resource. This filter is independent of the tag-key
* filter.
-
virtualization-type
- The
* virtualization type of the instance (paravirtual
|
* hvm
).
-
vpc-id
- The ID of the
* VPC that the instance is running in.
-
*
network-interface.description
- The description of the
* network interface.
-
*
network-interface.subnet-id
- The ID of the subnet for
* the network interface.
-
*
network-interface.vpc-id
- The ID of the VPC for the
* network interface.
-
*
network-interface.network-interface.id
- The ID of the
* network interface.
-
*
network-interface.owner-id
- The ID of the owner of
* the network interface.
-
*
network-interface.availability-zone
- The Availability
* Zone for the network interface.
-
*
network-interface.requester-id
- The requester ID for
* the network interface.
-
*
network-interface.requester-managed
- Indicates
* whether the network interface is being managed by AWS.
-
*
network-interface.status
- The status of the network
* interface (available
) | in-use
).
-
*
network-interface.mac-address
- The MAC address of the
* network interface.
-
*
network-interface-private-dns-name
- The private DNS
* name of the network interface.
-
*
network-interface.source-destination-check
- Whether
* the network interface performs source/destination checking. A value of
* true
means checking is enabled, and false
* means checking is disabled. The value must be false
for
* the network interface to perform network address translation (NAT) in
* your VPC.
-
network-interface.group-id
- The
* ID of a security group associated with the network interface.
* -
network-interface.group-name
- The name of a
* security group associated with the network interface.
-
*
network-interface.attachment.attachment-id
- The ID of
* the interface attachment.
-
*
network-interface.attachment.instance-id
- The ID of
* the instance to which the network interface is attached.
-
*
network-interface.attachment.instance-owner-id
- The
* owner ID of the instance to which the network interface is attached.
*
-
*
network-interface.addresses.private-ip-address
- The
* private IP address associated with the network interface.
-
*
network-interface.attachment.device-index
- The device
* index to which the network interface is attached.
-
*
network-interface.attachment.status
- The status of
* the attachment (attaching
| attached
|
* detaching
| detached
).
-
*
network-interface.attachment.attach-time
- The time
* that the network interface was attached to an instance.
-
*
network-interface.attachment.delete-on-termination
-
* Specifies whether the attachment is deleted when an instance is
* terminated.
-
*
network-interface.addresses.primary
- Specifies
* whether the IP address of the network interface is the primary private
* IP address.
-
*
network-interface.addresses.association.public-ip
-
* The ID of the association of an Elastic IP address with a network
* interface.
-
*
network-interface.addresses.association.ip-owner-id
-
* The owner ID of the private IP address associated with the network
* interface.
-
association.public-ip
- The
* address of the Elastic IP address bound to the network interface.
*
-
association.ip-owner-id
- The owner of the
* Elastic IP address associated with the network interface.
-
*
association.allocation-id
- The allocation ID returned
* when you allocated the Elastic IP address for your network interface.
*
-
association.association-id
- The
* association ID returned when the network interface was associated with
* an IP address.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param filters One or more filters.
-
architecture
- The
* instance architecture (i386
| x86_64
).
* -
availability-zone
- The Availability Zone of the
* instance.
-
block-device-mapping.attach-time
* - The attach time for an Amazon EBS volume mapped to the instance.
*
-
block-device-mapping.delete-on-termination
* - A Boolean that indicates whether the Amazon EBS volume is deleted on
* instance termination.
-
*
block-device-mapping.device-name
- The device name for
* the Amazon EBS volume (for example, /dev/sdh
).
-
*
block-device-mapping.status
- The status for the
* Amazon EBS volume (attaching
| attached
|
* detaching
| detached
).
-
*
block-device-mapping.volume-id
- The volume ID of the
* Amazon EBS volume.
-
client-token
- The
* idempotency token you provided when you launched the instance.
* -
dns-name
- The public DNS name of the instance.
*
-
group-id
- The ID of the security group for
* the instance. If the instance is in EC2-Classic or a default VPC, you
* can use group-name
instead.
-
*
group-name
- The name of the security group for the
* instance. If the instance is in a nondefault VPC, you must use
* group-id
instead.
-
hypervisor
-
* The hypervisor type of the instance (ovm
|
* xen
).
-
image-id
- The ID of the
* image used to launch the instance.
-
*
instance-id
- The ID of the instance.
-
*
instance-lifecycle
- Indicates whether this is a Spot
* Instance (spot
).
-
*
instance-state-code
- The state of the instance, as a
* 16-bit unsigned integer. The high byte is an opaque internal value and
* should be ignored. The low byte is set based on the state represented.
* The valid values are: 0 (pending), 16 (running), 32 (shutting-down),
* 48 (terminated), 64 (stopping), and 80 (stopped).
-
*
instance-state-name
- The state of the instance
* (pending
| running
|
* shutting-down
| terminated
|
* stopping
| stopped
).
-
*
instance-type
- The type of instance (for example,
* m1.small
).
-
instance.group-id
-
* The ID of the security group for the instance. If the instance is in
* EC2-Classic or a default VPC, you can use
* instance.group-name
instead.
-
*
instance.group-name
- The name of the security group
* for the instance. If the instance is in a nondefault VPC, you must use
* instance.group-id
instead.
-
*
ip-address
- The public IP address of the instance.
*
-
kernel-id
- The kernel ID.
-
*
key-name
- The name of the key pair used when the
* instance was launched.
-
launch-index
- When
* launching multiple instances, this is the index for the instance in
* the launch group (for example, 0, 1, 2, and so on).
-
*
launch-time
- The time when the instance was launched.
*
-
monitoring-state
- Indicates whether
* monitoring is enabled for the instance (disabled
|
* enabled
).
-
owner-id
- The AWS
* account ID of the instance owner.
-
*
placement-group-name
- The name of the placement group
* for the instance.
-
platform
- The platform.
* Use windows
if you have Windows instances; otherwise,
* leave blank.
-
private-dns-name
- The private
* DNS name of the instance.
-
*
private-ip-address
- The private IP address of the
* instance.
-
product-code
- The product code
* associated with the AMI used to launch the instance.
-
*
product-code.type
- The type of product code
* (devpay
| marketplace
).
-
*
ramdisk-id
- The RAM disk ID.
-
*
reason
- The reason for the current state of the
* instance (for example, shows "User Initiated [date]" when you stop or
* terminate the instance). Similar to the state-reason-code filter.
*
-
requester-id
- The ID of the entity that
* launched the instance on your behalf (for example, AWS Management
* Console, Auto Scaling, and so on).
-
*
reservation-id
- The ID of the instance's reservation.
* A reservation ID is created any time you launch an instance. A
* reservation ID has a one-to-one relationship with an instance launch
* request, but can be associated with more than one instance if you
* launch multiple instances using the same launch request. For example,
* if you launch one instance, you'll get one reservation ID. If you
* launch ten instances using the same launch request, you'll also get
* one reservation ID.
-
root-device-name
- The
* name of the root device for the instance (for example,
* /dev/sda1
).
-
root-device-type
-
* The type of root device that the instance uses (ebs
|
* instance-store
).
-
*
source-dest-check
- Indicates whether the instance
* performs source/destination checking. A value of true
* means that checking is enabled, and false
means checking
* is disabled. The value must be false
for the instance to
* perform network address translation (NAT) in your VPC.
-
*
spot-instance-request-id
- The ID of the Spot Instance
* request.
-
state-reason-code
- The reason
* code for the state change.
-
*
state-reason-message
- A message that describes the
* state change.
-
subnet-id
- The ID of the
* subnet for the instance.
-
*
tag
:key=value - The key/value
* combination of a tag assigned to the resource, where
* tag
:key is the tag's key.
-
*
tag-key
- The key of a tag assigned to the resource.
* This filter is independent of the tag-value
filter. For
* example, if you use both the filter "tag-key=Purpose" and the filter
* "tag-value=X", you get any resources assigned both the tag key Purpose
* (regardless of what the tag's value is), and the tag value X
* (regardless of what the tag's key is). If you want to list only
* resources where Purpose is X, see the
* tag
:key=value filter.
-
*
tag-value
- The value of a tag assigned to the
* resource. This filter is independent of the tag-key
* filter.
-
virtualization-type
- The
* virtualization type of the instance (paravirtual
|
* hvm
).
-
vpc-id
- The ID of the
* VPC that the instance is running in.
-
*
network-interface.description
- The description of the
* network interface.
-
*
network-interface.subnet-id
- The ID of the subnet for
* the network interface.
-
*
network-interface.vpc-id
- The ID of the VPC for the
* network interface.
-
*
network-interface.network-interface.id
- The ID of the
* network interface.
-
*
network-interface.owner-id
- The ID of the owner of
* the network interface.
-
*
network-interface.availability-zone
- The Availability
* Zone for the network interface.
-
*
network-interface.requester-id
- The requester ID for
* the network interface.
-
*
network-interface.requester-managed
- Indicates
* whether the network interface is being managed by AWS.
-
*
network-interface.status
- The status of the network
* interface (available
) | in-use
).
-
*
network-interface.mac-address
- The MAC address of the
* network interface.
-
*
network-interface-private-dns-name
- The private DNS
* name of the network interface.
-
*
network-interface.source-destination-check
- Whether
* the network interface performs source/destination checking. A value of
* true
means checking is enabled, and false
* means checking is disabled. The value must be false
for
* the network interface to perform network address translation (NAT) in
* your VPC.
-
network-interface.group-id
- The
* ID of a security group associated with the network interface.
* -
network-interface.group-name
- The name of a
* security group associated with the network interface.
-
*
network-interface.attachment.attachment-id
- The ID of
* the interface attachment.
-
*
network-interface.attachment.instance-id
- The ID of
* the instance to which the network interface is attached.
-
*
network-interface.attachment.instance-owner-id
- The
* owner ID of the instance to which the network interface is attached.
*
-
*
network-interface.addresses.private-ip-address
- The
* private IP address associated with the network interface.
-
*
network-interface.attachment.device-index
- The device
* index to which the network interface is attached.
-
*
network-interface.attachment.status
- The status of
* the attachment (attaching
| attached
|
* detaching
| detached
).
-
*
network-interface.attachment.attach-time
- The time
* that the network interface was attached to an instance.
-
*
network-interface.attachment.delete-on-termination
-
* Specifies whether the attachment is deleted when an instance is
* terminated.
-
*
network-interface.addresses.primary
- Specifies
* whether the IP address of the network interface is the primary private
* IP address.
-
*
network-interface.addresses.association.public-ip
-
* The ID of the association of an Elastic IP address with a network
* interface.
-
*
network-interface.addresses.association.ip-owner-id
-
* The owner ID of the private IP address associated with the network
* interface.
-
association.public-ip
- The
* address of the Elastic IP address bound to the network interface.
*
-
association.ip-owner-id
- The owner of the
* Elastic IP address associated with the network interface.
-
*
association.allocation-id
- The allocation ID returned
* when you allocated the Elastic IP address for your network interface.
*
-
association.association-id
- The
* association ID returned when the network interface was associated with
* an IP address.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public DescribeInstancesRequest withFilters(Filter... filters) {
if (getFilters() == null) setFilters(new java.util.ArrayList(filters.length));
for (Filter value : filters) {
getFilters().add(value);
}
return this;
}
/**
* One or more filters. -
architecture
- The
* instance architecture (i386
| x86_64
).
* -
availability-zone
- The Availability Zone of the
* instance.
-
block-device-mapping.attach-time
* - The attach time for an Amazon EBS volume mapped to the instance.
*
-
block-device-mapping.delete-on-termination
* - A Boolean that indicates whether the Amazon EBS volume is deleted on
* instance termination.
-
*
block-device-mapping.device-name
- The device name for
* the Amazon EBS volume (for example, /dev/sdh
).
-
*
block-device-mapping.status
- The status for the
* Amazon EBS volume (attaching
| attached
|
* detaching
| detached
).
-
*
block-device-mapping.volume-id
- The volume ID of the
* Amazon EBS volume.
-
client-token
- The
* idempotency token you provided when you launched the instance.
* -
dns-name
- The public DNS name of the instance.
*
-
group-id
- The ID of the security group for
* the instance. If the instance is in EC2-Classic or a default VPC, you
* can use group-name
instead.
-
*
group-name
- The name of the security group for the
* instance. If the instance is in a nondefault VPC, you must use
* group-id
instead.
-
hypervisor
-
* The hypervisor type of the instance (ovm
|
* xen
).
-
image-id
- The ID of the
* image used to launch the instance.
-
*
instance-id
- The ID of the instance.
-
*
instance-lifecycle
- Indicates whether this is a Spot
* Instance (spot
).
-
*
instance-state-code
- The state of the instance, as a
* 16-bit unsigned integer. The high byte is an opaque internal value and
* should be ignored. The low byte is set based on the state represented.
* The valid values are: 0 (pending), 16 (running), 32 (shutting-down),
* 48 (terminated), 64 (stopping), and 80 (stopped).
-
*
instance-state-name
- The state of the instance
* (pending
| running
|
* shutting-down
| terminated
|
* stopping
| stopped
).
-
*
instance-type
- The type of instance (for example,
* m1.small
).
-
instance.group-id
-
* The ID of the security group for the instance. If the instance is in
* EC2-Classic or a default VPC, you can use
* instance.group-name
instead.
-
*
instance.group-name
- The name of the security group
* for the instance. If the instance is in a nondefault VPC, you must use
* instance.group-id
instead.
-
*
ip-address
- The public IP address of the instance.
*
-
kernel-id
- The kernel ID.
-
*
key-name
- The name of the key pair used when the
* instance was launched.
-
launch-index
- When
* launching multiple instances, this is the index for the instance in
* the launch group (for example, 0, 1, 2, and so on).
-
*
launch-time
- The time when the instance was launched.
*
-
monitoring-state
- Indicates whether
* monitoring is enabled for the instance (disabled
|
* enabled
).
-
owner-id
- The AWS
* account ID of the instance owner.
-
*
placement-group-name
- The name of the placement group
* for the instance.
-
platform
- The platform.
* Use windows
if you have Windows instances; otherwise,
* leave blank.
-
private-dns-name
- The private
* DNS name of the instance.
-
*
private-ip-address
- The private IP address of the
* instance.
-
product-code
- The product code
* associated with the AMI used to launch the instance.
-
*
product-code.type
- The type of product code
* (devpay
| marketplace
).
-
*
ramdisk-id
- The RAM disk ID.
-
*
reason
- The reason for the current state of the
* instance (for example, shows "User Initiated [date]" when you stop or
* terminate the instance). Similar to the state-reason-code filter.
*
-
requester-id
- The ID of the entity that
* launched the instance on your behalf (for example, AWS Management
* Console, Auto Scaling, and so on).
-
*
reservation-id
- The ID of the instance's reservation.
* A reservation ID is created any time you launch an instance. A
* reservation ID has a one-to-one relationship with an instance launch
* request, but can be associated with more than one instance if you
* launch multiple instances using the same launch request. For example,
* if you launch one instance, you'll get one reservation ID. If you
* launch ten instances using the same launch request, you'll also get
* one reservation ID.
-
root-device-name
- The
* name of the root device for the instance (for example,
* /dev/sda1
).
-
root-device-type
-
* The type of root device that the instance uses (ebs
|
* instance-store
).
-
*
source-dest-check
- Indicates whether the instance
* performs source/destination checking. A value of true
* means that checking is enabled, and false
means checking
* is disabled. The value must be false
for the instance to
* perform network address translation (NAT) in your VPC.
-
*
spot-instance-request-id
- The ID of the Spot Instance
* request.
-
state-reason-code
- The reason
* code for the state change.
-
*
state-reason-message
- A message that describes the
* state change.
-
subnet-id
- The ID of the
* subnet for the instance.
-
*
tag
:key=value - The key/value
* combination of a tag assigned to the resource, where
* tag
:key is the tag's key.
-
*
tag-key
- The key of a tag assigned to the resource.
* This filter is independent of the tag-value
filter. For
* example, if you use both the filter "tag-key=Purpose" and the filter
* "tag-value=X", you get any resources assigned both the tag key Purpose
* (regardless of what the tag's value is), and the tag value X
* (regardless of what the tag's key is). If you want to list only
* resources where Purpose is X, see the
* tag
:key=value filter.
-
*
tag-value
- The value of a tag assigned to the
* resource. This filter is independent of the tag-key
* filter.
-
virtualization-type
- The
* virtualization type of the instance (paravirtual
|
* hvm
).
-
vpc-id
- The ID of the
* VPC that the instance is running in.
-
*
network-interface.description
- The description of the
* network interface.
-
*
network-interface.subnet-id
- The ID of the subnet for
* the network interface.
-
*
network-interface.vpc-id
- The ID of the VPC for the
* network interface.
-
*
network-interface.network-interface.id
- The ID of the
* network interface.
-
*
network-interface.owner-id
- The ID of the owner of
* the network interface.
-
*
network-interface.availability-zone
- The Availability
* Zone for the network interface.
-
*
network-interface.requester-id
- The requester ID for
* the network interface.
-
*
network-interface.requester-managed
- Indicates
* whether the network interface is being managed by AWS.
-
*
network-interface.status
- The status of the network
* interface (available
) | in-use
).
-
*
network-interface.mac-address
- The MAC address of the
* network interface.
-
*
network-interface-private-dns-name
- The private DNS
* name of the network interface.
-
*
network-interface.source-destination-check
- Whether
* the network interface performs source/destination checking. A value of
* true
means checking is enabled, and false
* means checking is disabled. The value must be false
for
* the network interface to perform network address translation (NAT) in
* your VPC.
-
network-interface.group-id
- The
* ID of a security group associated with the network interface.
* -
network-interface.group-name
- The name of a
* security group associated with the network interface.
-
*
network-interface.attachment.attachment-id
- The ID of
* the interface attachment.
-
*
network-interface.attachment.instance-id
- The ID of
* the instance to which the network interface is attached.
-
*
network-interface.attachment.instance-owner-id
- The
* owner ID of the instance to which the network interface is attached.
*
-
*
network-interface.addresses.private-ip-address
- The
* private IP address associated with the network interface.
-
*
network-interface.attachment.device-index
- The device
* index to which the network interface is attached.
-
*
network-interface.attachment.status
- The status of
* the attachment (attaching
| attached
|
* detaching
| detached
).
-
*
network-interface.attachment.attach-time
- The time
* that the network interface was attached to an instance.
-
*
network-interface.attachment.delete-on-termination
-
* Specifies whether the attachment is deleted when an instance is
* terminated.
-
*
network-interface.addresses.primary
- Specifies
* whether the IP address of the network interface is the primary private
* IP address.
-
*
network-interface.addresses.association.public-ip
-
* The ID of the association of an Elastic IP address with a network
* interface.
-
*
network-interface.addresses.association.ip-owner-id
-
* The owner ID of the private IP address associated with the network
* interface.
-
association.public-ip
- The
* address of the Elastic IP address bound to the network interface.
*
-
association.ip-owner-id
- The owner of the
* Elastic IP address associated with the network interface.
-
*
association.allocation-id
- The allocation ID returned
* when you allocated the Elastic IP address for your network interface.
*
-
association.association-id
- The
* association ID returned when the network interface was associated with
* an IP address.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param filters One or more filters.
-
architecture
- The
* instance architecture (i386
| x86_64
).
* -
availability-zone
- The Availability Zone of the
* instance.
-
block-device-mapping.attach-time
* - The attach time for an Amazon EBS volume mapped to the instance.
*
-
block-device-mapping.delete-on-termination
* - A Boolean that indicates whether the Amazon EBS volume is deleted on
* instance termination.
-
*
block-device-mapping.device-name
- The device name for
* the Amazon EBS volume (for example, /dev/sdh
).
-
*
block-device-mapping.status
- The status for the
* Amazon EBS volume (attaching
| attached
|
* detaching
| detached
).
-
*
block-device-mapping.volume-id
- The volume ID of the
* Amazon EBS volume.
-
client-token
- The
* idempotency token you provided when you launched the instance.
* -
dns-name
- The public DNS name of the instance.
*
-
group-id
- The ID of the security group for
* the instance. If the instance is in EC2-Classic or a default VPC, you
* can use group-name
instead.
-
*
group-name
- The name of the security group for the
* instance. If the instance is in a nondefault VPC, you must use
* group-id
instead.
-
hypervisor
-
* The hypervisor type of the instance (ovm
|
* xen
).
-
image-id
- The ID of the
* image used to launch the instance.
-
*
instance-id
- The ID of the instance.
-
*
instance-lifecycle
- Indicates whether this is a Spot
* Instance (spot
).
-
*
instance-state-code
- The state of the instance, as a
* 16-bit unsigned integer. The high byte is an opaque internal value and
* should be ignored. The low byte is set based on the state represented.
* The valid values are: 0 (pending), 16 (running), 32 (shutting-down),
* 48 (terminated), 64 (stopping), and 80 (stopped).
-
*
instance-state-name
- The state of the instance
* (pending
| running
|
* shutting-down
| terminated
|
* stopping
| stopped
).
-
*
instance-type
- The type of instance (for example,
* m1.small
).
-
instance.group-id
-
* The ID of the security group for the instance. If the instance is in
* EC2-Classic or a default VPC, you can use
* instance.group-name
instead.
-
*
instance.group-name
- The name of the security group
* for the instance. If the instance is in a nondefault VPC, you must use
* instance.group-id
instead.
-
*
ip-address
- The public IP address of the instance.
*
-
kernel-id
- The kernel ID.
-
*
key-name
- The name of the key pair used when the
* instance was launched.
-
launch-index
- When
* launching multiple instances, this is the index for the instance in
* the launch group (for example, 0, 1, 2, and so on).
-
*
launch-time
- The time when the instance was launched.
*
-
monitoring-state
- Indicates whether
* monitoring is enabled for the instance (disabled
|
* enabled
).
-
owner-id
- The AWS
* account ID of the instance owner.
-
*
placement-group-name
- The name of the placement group
* for the instance.
-
platform
- The platform.
* Use windows
if you have Windows instances; otherwise,
* leave blank.
-
private-dns-name
- The private
* DNS name of the instance.
-
*
private-ip-address
- The private IP address of the
* instance.
-
product-code
- The product code
* associated with the AMI used to launch the instance.
-
*
product-code.type
- The type of product code
* (devpay
| marketplace
).
-
*
ramdisk-id
- The RAM disk ID.
-
*
reason
- The reason for the current state of the
* instance (for example, shows "User Initiated [date]" when you stop or
* terminate the instance). Similar to the state-reason-code filter.
*
-
requester-id
- The ID of the entity that
* launched the instance on your behalf (for example, AWS Management
* Console, Auto Scaling, and so on).
-
*
reservation-id
- The ID of the instance's reservation.
* A reservation ID is created any time you launch an instance. A
* reservation ID has a one-to-one relationship with an instance launch
* request, but can be associated with more than one instance if you
* launch multiple instances using the same launch request. For example,
* if you launch one instance, you'll get one reservation ID. If you
* launch ten instances using the same launch request, you'll also get
* one reservation ID.
-
root-device-name
- The
* name of the root device for the instance (for example,
* /dev/sda1
).
-
root-device-type
-
* The type of root device that the instance uses (ebs
|
* instance-store
).
-
*
source-dest-check
- Indicates whether the instance
* performs source/destination checking. A value of true
* means that checking is enabled, and false
means checking
* is disabled. The value must be false
for the instance to
* perform network address translation (NAT) in your VPC.
-
*
spot-instance-request-id
- The ID of the Spot Instance
* request.
-
state-reason-code
- The reason
* code for the state change.
-
*
state-reason-message
- A message that describes the
* state change.
-
subnet-id
- The ID of the
* subnet for the instance.
-
*
tag
:key=value - The key/value
* combination of a tag assigned to the resource, where
* tag
:key is the tag's key.
-
*
tag-key
- The key of a tag assigned to the resource.
* This filter is independent of the tag-value
filter. For
* example, if you use both the filter "tag-key=Purpose" and the filter
* "tag-value=X", you get any resources assigned both the tag key Purpose
* (regardless of what the tag's value is), and the tag value X
* (regardless of what the tag's key is). If you want to list only
* resources where Purpose is X, see the
* tag
:key=value filter.
-
*
tag-value
- The value of a tag assigned to the
* resource. This filter is independent of the tag-key
* filter.
-
virtualization-type
- The
* virtualization type of the instance (paravirtual
|
* hvm
).
-
vpc-id
- The ID of the
* VPC that the instance is running in.
-
*
network-interface.description
- The description of the
* network interface.
-
*
network-interface.subnet-id
- The ID of the subnet for
* the network interface.
-
*
network-interface.vpc-id
- The ID of the VPC for the
* network interface.
-
*
network-interface.network-interface.id
- The ID of the
* network interface.
-
*
network-interface.owner-id
- The ID of the owner of
* the network interface.
-
*
network-interface.availability-zone
- The Availability
* Zone for the network interface.
-
*
network-interface.requester-id
- The requester ID for
* the network interface.
-
*
network-interface.requester-managed
- Indicates
* whether the network interface is being managed by AWS.
-
*
network-interface.status
- The status of the network
* interface (available
) | in-use
).
-
*
network-interface.mac-address
- The MAC address of the
* network interface.
-
*
network-interface-private-dns-name
- The private DNS
* name of the network interface.
-
*
network-interface.source-destination-check
- Whether
* the network interface performs source/destination checking. A value of
* true
means checking is enabled, and false
* means checking is disabled. The value must be false
for
* the network interface to perform network address translation (NAT) in
* your VPC.
-
network-interface.group-id
- The
* ID of a security group associated with the network interface.
* -
network-interface.group-name
- The name of a
* security group associated with the network interface.
-
*
network-interface.attachment.attachment-id
- The ID of
* the interface attachment.
-
*
network-interface.attachment.instance-id
- The ID of
* the instance to which the network interface is attached.
-
*
network-interface.attachment.instance-owner-id
- The
* owner ID of the instance to which the network interface is attached.
*
-
*
network-interface.addresses.private-ip-address
- The
* private IP address associated with the network interface.
-
*
network-interface.attachment.device-index
- The device
* index to which the network interface is attached.
-
*
network-interface.attachment.status
- The status of
* the attachment (attaching
| attached
|
* detaching
| detached
).
-
*
network-interface.attachment.attach-time
- The time
* that the network interface was attached to an instance.
-
*
network-interface.attachment.delete-on-termination
-
* Specifies whether the attachment is deleted when an instance is
* terminated.
-
*
network-interface.addresses.primary
- Specifies
* whether the IP address of the network interface is the primary private
* IP address.
-
*
network-interface.addresses.association.public-ip
-
* The ID of the association of an Elastic IP address with a network
* interface.
-
*
network-interface.addresses.association.ip-owner-id
-
* The owner ID of the private IP address associated with the network
* interface.
-
association.public-ip
- The
* address of the Elastic IP address bound to the network interface.
*
-
association.ip-owner-id
- The owner of the
* Elastic IP address associated with the network interface.
-
*
association.allocation-id
- The allocation ID returned
* when you allocated the Elastic IP address for your network interface.
*
-
association.association-id
- The
* association ID returned when the network interface was associated with
* an IP address.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public DescribeInstancesRequest withFilters(java.util.Collection filters) {
if (filters == null) {
this.filters = null;
} else {
com.amazonaws.internal.ListWithAutoConstructFlag filtersCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(filters.size());
filtersCopy.addAll(filters);
this.filters = filtersCopy;
}
return this;
}
/**
* The token for the next set of items to return. (You received this
* token from a prior call.)
*
* @return The token for the next set of items to return. (You received this
* token from a prior call.)
*/
public String getNextToken() {
return nextToken;
}
/**
* The token for the next set of items to return. (You received this
* token from a prior call.)
*
* @param nextToken The token for the next set of items to return. (You received this
* token from a prior call.)
*/
public void setNextToken(String nextToken) {
this.nextToken = nextToken;
}
/**
* The token for the next set of items to return. (You received this
* token from a prior call.)
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param nextToken The token for the next set of items to return. (You received this
* token from a prior call.)
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public DescribeInstancesRequest withNextToken(String nextToken) {
this.nextToken = nextToken;
return this;
}
/**
* The maximum number of items to return for this call. The call also
* returns a token that you can specify in a subsequent call to get the
* next set of results. If the value is greater than 1000, we return only
* 1000 items.
*
* @return The maximum number of items to return for this call. The call also
* returns a token that you can specify in a subsequent call to get the
* next set of results. If the value is greater than 1000, we return only
* 1000 items.
*/
public Integer getMaxResults() {
return maxResults;
}
/**
* The maximum number of items to return for this call. The call also
* returns a token that you can specify in a subsequent call to get the
* next set of results. If the value is greater than 1000, we return only
* 1000 items.
*
* @param maxResults The maximum number of items to return for this call. The call also
* returns a token that you can specify in a subsequent call to get the
* next set of results. If the value is greater than 1000, we return only
* 1000 items.
*/
public void setMaxResults(Integer maxResults) {
this.maxResults = maxResults;
}
/**
* The maximum number of items to return for this call. The call also
* returns a token that you can specify in a subsequent call to get the
* next set of results. If the value is greater than 1000, we return only
* 1000 items.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param maxResults The maximum number of items to return for this call. The call also
* returns a token that you can specify in a subsequent call to get the
* next set of results. If the value is greater than 1000, we return only
* 1000 items.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public DescribeInstancesRequest withMaxResults(Integer maxResults) {
this.maxResults = maxResults;
return this;
}
/**
* This method is intended for internal use only.
* Returns the marshaled request configured with additional parameters to
* enable operation dry-run.
*/
@Override
public Request getDryRunRequest() {
Request request = new DescribeInstancesRequestMarshaller().marshall(this);
request.addParameter("DryRun", Boolean.toString(true));
return request;
}
/**
* Returns a string representation of this object; useful for testing and
* debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getInstanceIds() != null) sb.append("InstanceIds: " + getInstanceIds() + ",");
if (getFilters() != null) sb.append("Filters: " + getFilters() + ",");
if (getNextToken() != null) sb.append("NextToken: " + getNextToken() + ",");
if (getMaxResults() != null) sb.append("MaxResults: " + getMaxResults() );
sb.append("}");
return sb.toString();
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getInstanceIds() == null) ? 0 : getInstanceIds().hashCode());
hashCode = prime * hashCode + ((getFilters() == null) ? 0 : getFilters().hashCode());
hashCode = prime * hashCode + ((getNextToken() == null) ? 0 : getNextToken().hashCode());
hashCode = prime * hashCode + ((getMaxResults() == null) ? 0 : getMaxResults().hashCode());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (obj == null) return false;
if (obj instanceof DescribeInstancesRequest == false) return false;
DescribeInstancesRequest other = (DescribeInstancesRequest)obj;
if (other.getInstanceIds() == null ^ this.getInstanceIds() == null) return false;
if (other.getInstanceIds() != null && other.getInstanceIds().equals(this.getInstanceIds()) == false) return false;
if (other.getFilters() == null ^ this.getFilters() == null) return false;
if (other.getFilters() != null && other.getFilters().equals(this.getFilters()) == false) return false;
if (other.getNextToken() == null ^ this.getNextToken() == null) return false;
if (other.getNextToken() != null && other.getNextToken().equals(this.getNextToken()) == false) return false;
if (other.getMaxResults() == null ^ this.getMaxResults() == null) return false;
if (other.getMaxResults() != null && other.getMaxResults().equals(this.getMaxResults()) == false) return false;
return true;
}
}