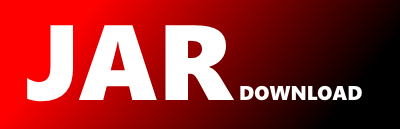
com.github.fakemongo.impl.aggregation.Lookup Maven / Gradle / Ivy
The newest version!
package com.github.fakemongo.impl.aggregation;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import org.junit.Assert;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.github.fakemongo.impl.ExpressionParser;
import com.mongodb.DB;
import com.mongodb.DBCollection;
import com.mongodb.DBCursor;
import com.mongodb.DBObject;
/**
* @author Kollivakkam Raghavan
* @created 4/22/2016
*/
public class Lookup extends PipelineKeyword {
private static final Logger LOG = LoggerFactory.getLogger(Lookup.class);
public static final Lookup INSTANCE = new Lookup();
public static final String ID = "_id";
@Override
public DBCollection apply(DB originalDB, DBCollection parentColl, DBObject object) {
DBObject lookup = ExpressionParser.toDbObject(object.get(getKeyword()));
List parentItems = performLookup(originalDB, parentColl, lookup);
return dropAndInsert(parentColl, parentItems);
}
@Override
public String getKeyword() {
return "$lookup";
}
private List performLookup(DB originalDB, DBCollection parentColl, DBObject lookup) {
String from = (String) lookup.get("from");
String localField = (String) lookup.get("localField");
String foreignField = (String) lookup.get("foreignField");
String as = (String) lookup.get("as");
LOG.debug("Value {} will be returned from {} in parent collection {}. Local field {} will be joined with {}",
as, from, parentColl.getName(), localField, foreignField);
DBCursor parentItems = parentColl.find();
// can't use lambdas
Iterator iterator = parentItems.iterator();
Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy