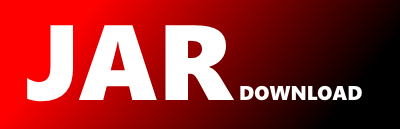
com.github.fanavarro.graphlib.SimpleGraphImpl Maven / Gradle / Ivy
package com.github.fanavarro.graphlib;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Map;
import java.util.Set;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
/**
* Map based graph implementation.
*
* @author fabad
* @param
* the node type
* @param
* the edge type
*/
public class SimpleGraphImpl extends AbstractGraph {
/**
*
*/
private static final long serialVersionUID = 2726268195016138010L;
/**
* The adjacent nodes. Key is a node, value is a map where the key is an
* edge and value is a set of nodes connected thought the edge.
*/
private Map>> adjacentNodes;
/**
* Instantiates a new simple graph impl.
*/
public SimpleGraphImpl() {
adjacentNodes = new HashMap>>();
}
/*
* (non-Javadoc)
*
* @see es.um.dis.graphlib.AbstractGraph#getNodes()
*/
@Override
public Set getNodes() {
return adjacentNodes.keySet();
}
/*
* (non-Javadoc)
*
* @see
* es.um.dis.graphlib.AbstractGraph#getAdjacentNodesWithEdges(java.lang.
* Object)
*/
@Override
public Map> getAdjacentNodesWithEdges(N node) {
return adjacentNodes.get(node);
}
/**
* Adds the node.
*
* @param node
* the node
*/
public void addNode(N node) {
if (!adjacentNodes.containsKey(node)) {
adjacentNodes.put(node, new HashMap>());
}
}
/**
* Adds the node.
*
* @param node
* the node
* @param edge
* the edge
* @param adjacentNode
* the adjacent node
*/
public void addNode(N node, E edge, N adjacentNode) {
if (!adjacentNodes.containsKey(node)) {
this.addNode(node);
}
if (!adjacentNodes.containsKey(adjacentNode)) {
this.addNode(adjacentNode);
}
if (!adjacentNodes.get(node).containsKey(edge)) {
adjacentNodes.get(node).put(edge, new HashSet());
}
adjacentNodes.get(node).get(edge).add(adjacentNode);
}
/**
* Adds the node.
*
* @param node
* the node
* @param edge
* the edge
* @param adjacentNodes
* the adjacent nodes
*/
public void addNode(N node, E edge, Set adjacentNodes) {
for (N adjacentNode : adjacentNodes) {
this.addNode(node, edge, adjacentNode);
}
}
/**
* {@inheritDoc}
*/
@Override
public boolean equals(final Object other) {
if (this == other) {
return true;
}
if (!(other instanceof SimpleGraphImpl)) {
return false;
}
SimpleGraphImpl, ?> castOther = (SimpleGraphImpl, ?>) other;
return new EqualsBuilder().append(adjacentNodes, castOther.adjacentNodes).isEquals();
}
/**
* {@inheritDoc}
*/
@Override
public int hashCode() {
return new HashCodeBuilder().append(adjacentNodes).toHashCode();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy