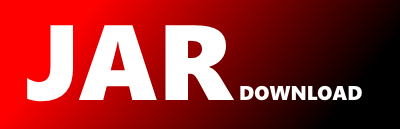
com.jn.agileway.codec.serialization.hessian.Hessians Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of agileway-codec Show documentation
Show all versions of agileway-codec Show documentation
Provide an unified codec API for hession, kryo, protostuff, fst, fes, xson, cbor, jackson, json, etc....
package com.jn.agileway.codec.serialization.hessian;
import com.caucho.hessian.io.Hessian2Input;
import com.caucho.hessian.io.Hessian2Output;
import com.jn.langx.Factory;
import com.jn.langx.annotation.NonNull;
import com.jn.langx.annotation.Nullable;
import com.jn.langx.codec.CodecException;
import com.jn.langx.text.StringTemplates;
import com.jn.langx.util.Preconditions;
import com.jn.langx.util.concurrent.threadlocal.ThreadLocalFactory;
import com.jn.langx.util.io.IOs;
import com.jn.langx.util.reflect.Reflects;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.io.OutputStream;
public class Hessians {
private Hessians() {
}
public static final ThreadLocalFactory, Hessian2Output> hessian2OutputFactory = new ThreadLocalFactory
© 2015 - 2025 Weber Informatics LLC | Privacy Policy