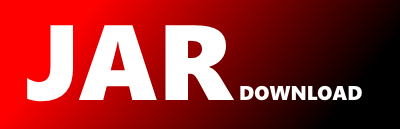
com.github.fanzezhen.oldutils.ReflectClassUtils Maven / Gradle / Ivy
package com.github.fanzezhen.oldutils;
import com.github.fanzezhen.bean.AttributeBean;
import org.springframework.beans.BeanWrapper;
import org.springframework.beans.BeanWrapperImpl;
import org.springframework.util.StringUtils;
import java.lang.reflect.Field;
import java.lang.reflect.Method;
import java.util.*;
/**
* Created by Andy on 2018/12/20.
* Desc:
*/
public class ReflectClassUtils {
private final static String TAG = "peter.log.ReflectClass";
/**
* @param bean
* @return
*/
public static Field[] getAttributeFields(Object bean) {
return bean.getClass().getDeclaredFields();
}
/**
* @param field
* @param bean
* @return
*/
public static AttributeBean getAttribute(Field field, Object bean) {
String name = field.getName();
String type = field.getGenericType().toString(); //获取属性的类型
Object value = null;
try {
//将属性的首字符大写,构造get方法
Method m = bean.getClass().getMethod("get" + name.substring(0, 1).toUpperCase() + name.substring(1));
value = m == null ? null : m.invoke(bean); //调用getter方法获取属性值
} catch (Exception e) {
e.printStackTrace();
}
return new AttributeBean(type, name, value);
}
public static HashMap javaBeanToHashMap(Object javaBean) throws Exception {
HashMap map = new HashMap<>();
Method[] methods = javaBean.getClass().getMethods(); // 获取所有方法
for (Method method : methods) {
if (method.getName().startsWith("get")) {
String field = method.getName(); // 拼接属性名
field = field.substring(field.indexOf("get") + 3);
field = field.toLowerCase().charAt(0) + field.substring(1);
Object value = method.invoke(javaBean, (Object[]) null); // 执行方法
map.put(field, value);
}
}
return map;
}
public static String[] getNullPropertyNames(Object source) {
final BeanWrapper src = new BeanWrapperImpl(source);
java.beans.PropertyDescriptor[] pds = src.getPropertyDescriptors();
Set emptyNames = new HashSet();
for (java.beans.PropertyDescriptor pd : pds) {
Object srcValue = src.getPropertyValue(pd.getName());
if (srcValue == null) emptyNames.add(pd.getName());
}
String[] result = new String[emptyNames.size()];
return emptyNames.toArray(result);
}
public static List> getDifferentPropertyList(Object oldObject, Object newObject) {
List> result = new ArrayList<>();
List oldFieldList = Arrays.asList(getAttributeFields(oldObject));
List newFieldList = Arrays.asList(getAttributeFields(newObject));
List fieldList = new ArrayList<>();
fieldList.addAll(oldFieldList);
fieldList.addAll(newFieldList);
for (Field field : new HashSet<>(fieldList)) {
HashMap hashMap = new HashMap<>();
if (!oldFieldList.contains(field)) {
Object newValue = getAttribute(field, newObject).getValue();
if (!StringUtils.isEmpty(newValue)) {
hashMap.put("name", field.getName());
hashMap.put("oldValue", null);
hashMap.put("newValue", newValue);
}
} else if (!newFieldList.contains(field)) {
Object oldValue = getAttribute(field, oldObject).getValue();
if (!StringUtils.isEmpty(oldValue)) {
hashMap.put("name", field.getName());
hashMap.put("oldValue", oldValue);
hashMap.put("newValue", null);
}
} else {
Object oldValue = getAttribute(field, oldObject).getValue();
Object newValue = getAttribute(field, newObject).getValue();
if (!StringUtils.isEmpty(oldValue)) {
if (!oldValue.equals(newValue)) {
hashMap.put("name", field.getName());
hashMap.put("oldValue", oldValue);
hashMap.put("newValue", newValue);
}
} else if (!StringUtils.isEmpty(newValue)) {
hashMap.put("name", field.getName());
hashMap.put("oldValue", "");
hashMap.put("newValue", newValue);
}
}
if (hashMap.size() > 0) {
result.add(hashMap);
}
}
return result;
}
public static void main(String[] args) {
System.out.println(getDifferentPropertyList(new AttributeBean("1", "1", "1"), new AttributeBean("2", "2", "2")));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy