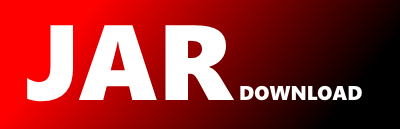
org.apache.jclouds.oneandone.rest.domain.AutoValue_LoadBalancer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jclouds-shaded Show documentation
Show all versions of jclouds-shaded Show documentation
Provides a shaded jclouds with relocated guava and guice
The newest version!
package org.apache.jclouds.oneandone.rest.domain;
import java.util.List;
import javax.annotation.Generated;
import org.jclouds.javax.annotation.Nullable;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_LoadBalancer extends LoadBalancer {
private final String id;
private final String name;
private final String description;
private final String state;
private final String creationDate;
private final String ip;
private final Types.HealthCheckTestTypes healthCheckTest;
private final int healthCheckInterval;
private final String healthCheckPath;
private final String healthCheckPathParser;
private final Boolean persistence;
private final Integer persistenceTime;
private final Types.LoadBalancerMethod method;
private final DataCenter datacenter;
private final List rules;
private final List serverIps;
AutoValue_LoadBalancer(
String id,
String name,
@Nullable String description,
@Nullable String state,
@Nullable String creationDate,
@Nullable String ip,
@Nullable Types.HealthCheckTestTypes healthCheckTest,
int healthCheckInterval,
@Nullable String healthCheckPath,
@Nullable String healthCheckPathParser,
Boolean persistence,
@Nullable Integer persistenceTime,
Types.LoadBalancerMethod method,
DataCenter datacenter,
List rules,
List serverIps) {
if (id == null) {
throw new NullPointerException("Null id");
}
this.id = id;
if (name == null) {
throw new NullPointerException("Null name");
}
this.name = name;
this.description = description;
this.state = state;
this.creationDate = creationDate;
this.ip = ip;
this.healthCheckTest = healthCheckTest;
this.healthCheckInterval = healthCheckInterval;
this.healthCheckPath = healthCheckPath;
this.healthCheckPathParser = healthCheckPathParser;
if (persistence == null) {
throw new NullPointerException("Null persistence");
}
this.persistence = persistence;
this.persistenceTime = persistenceTime;
if (method == null) {
throw new NullPointerException("Null method");
}
this.method = method;
if (datacenter == null) {
throw new NullPointerException("Null datacenter");
}
this.datacenter = datacenter;
if (rules == null) {
throw new NullPointerException("Null rules");
}
this.rules = rules;
if (serverIps == null) {
throw new NullPointerException("Null serverIps");
}
this.serverIps = serverIps;
}
@Override
public String id() {
return id;
}
@Override
public String name() {
return name;
}
@Nullable
@Override
public String description() {
return description;
}
@Nullable
@Override
public String state() {
return state;
}
@Nullable
@Override
public String creationDate() {
return creationDate;
}
@Nullable
@Override
public String ip() {
return ip;
}
@Nullable
@Override
public Types.HealthCheckTestTypes healthCheckTest() {
return healthCheckTest;
}
@Override
public int healthCheckInterval() {
return healthCheckInterval;
}
@Nullable
@Override
public String healthCheckPath() {
return healthCheckPath;
}
@Nullable
@Override
public String healthCheckPathParser() {
return healthCheckPathParser;
}
@Override
public Boolean persistence() {
return persistence;
}
@Nullable
@Override
public Integer persistenceTime() {
return persistenceTime;
}
@Override
public Types.LoadBalancerMethod method() {
return method;
}
@Override
public DataCenter datacenter() {
return datacenter;
}
@Override
public List rules() {
return rules;
}
@Override
public List serverIps() {
return serverIps;
}
@Override
public String toString() {
return "LoadBalancer{"
+ "id=" + id + ", "
+ "name=" + name + ", "
+ "description=" + description + ", "
+ "state=" + state + ", "
+ "creationDate=" + creationDate + ", "
+ "ip=" + ip + ", "
+ "healthCheckTest=" + healthCheckTest + ", "
+ "healthCheckInterval=" + healthCheckInterval + ", "
+ "healthCheckPath=" + healthCheckPath + ", "
+ "healthCheckPathParser=" + healthCheckPathParser + ", "
+ "persistence=" + persistence + ", "
+ "persistenceTime=" + persistenceTime + ", "
+ "method=" + method + ", "
+ "datacenter=" + datacenter + ", "
+ "rules=" + rules + ", "
+ "serverIps=" + serverIps
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof LoadBalancer) {
LoadBalancer that = (LoadBalancer) o;
return (this.id.equals(that.id()))
&& (this.name.equals(that.name()))
&& ((this.description == null) ? (that.description() == null) : this.description.equals(that.description()))
&& ((this.state == null) ? (that.state() == null) : this.state.equals(that.state()))
&& ((this.creationDate == null) ? (that.creationDate() == null) : this.creationDate.equals(that.creationDate()))
&& ((this.ip == null) ? (that.ip() == null) : this.ip.equals(that.ip()))
&& ((this.healthCheckTest == null) ? (that.healthCheckTest() == null) : this.healthCheckTest.equals(that.healthCheckTest()))
&& (this.healthCheckInterval == that.healthCheckInterval())
&& ((this.healthCheckPath == null) ? (that.healthCheckPath() == null) : this.healthCheckPath.equals(that.healthCheckPath()))
&& ((this.healthCheckPathParser == null) ? (that.healthCheckPathParser() == null) : this.healthCheckPathParser.equals(that.healthCheckPathParser()))
&& (this.persistence.equals(that.persistence()))
&& ((this.persistenceTime == null) ? (that.persistenceTime() == null) : this.persistenceTime.equals(that.persistenceTime()))
&& (this.method.equals(that.method()))
&& (this.datacenter.equals(that.datacenter()))
&& (this.rules.equals(that.rules()))
&& (this.serverIps.equals(that.serverIps()));
}
return false;
}
@Override
public int hashCode() {
int h = 1;
h *= 1000003;
h ^= this.id.hashCode();
h *= 1000003;
h ^= this.name.hashCode();
h *= 1000003;
h ^= (description == null) ? 0 : this.description.hashCode();
h *= 1000003;
h ^= (state == null) ? 0 : this.state.hashCode();
h *= 1000003;
h ^= (creationDate == null) ? 0 : this.creationDate.hashCode();
h *= 1000003;
h ^= (ip == null) ? 0 : this.ip.hashCode();
h *= 1000003;
h ^= (healthCheckTest == null) ? 0 : this.healthCheckTest.hashCode();
h *= 1000003;
h ^= this.healthCheckInterval;
h *= 1000003;
h ^= (healthCheckPath == null) ? 0 : this.healthCheckPath.hashCode();
h *= 1000003;
h ^= (healthCheckPathParser == null) ? 0 : this.healthCheckPathParser.hashCode();
h *= 1000003;
h ^= this.persistence.hashCode();
h *= 1000003;
h ^= (persistenceTime == null) ? 0 : this.persistenceTime.hashCode();
h *= 1000003;
h ^= this.method.hashCode();
h *= 1000003;
h ^= this.datacenter.hashCode();
h *= 1000003;
h ^= this.rules.hashCode();
h *= 1000003;
h ^= this.serverIps.hashCode();
return h;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy