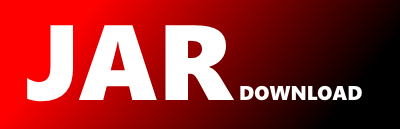
org.apache.jclouds.oneandone.rest.domain.AutoValue_ServerAppliance Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jclouds-shaded Show documentation
Show all versions of jclouds-shaded Show documentation
Provides a shaded jclouds with relocated guava and guice
The newest version!
package org.apache.jclouds.oneandone.rest.domain;
import java.util.List;
import javax.annotation.Generated;
import org.jclouds.javax.annotation.Nullable;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_ServerAppliance extends ServerAppliance {
private final String id;
private final String name;
private final List availableDataCenters;
private final String osInstallationBase;
private final Types.OSFamliyType osFamily;
private final String os;
private final String osVersion;
private final int osArchitecture;
private final Types.OSImageType osImageType;
private final int minHddSize;
private final Types.ApplianceType type;
private final String state;
private final String version;
private final List categories;
private final String eulaUrl;
AutoValue_ServerAppliance(
String id,
String name,
@Nullable List availableDataCenters,
@Nullable String osInstallationBase,
@Nullable Types.OSFamliyType osFamily,
@Nullable String os,
@Nullable String osVersion,
int osArchitecture,
@Nullable Types.OSImageType osImageType,
int minHddSize,
@Nullable Types.ApplianceType type,
@Nullable String state,
@Nullable String version,
@Nullable List categories,
@Nullable String eulaUrl) {
if (id == null) {
throw new NullPointerException("Null id");
}
this.id = id;
if (name == null) {
throw new NullPointerException("Null name");
}
this.name = name;
this.availableDataCenters = availableDataCenters;
this.osInstallationBase = osInstallationBase;
this.osFamily = osFamily;
this.os = os;
this.osVersion = osVersion;
this.osArchitecture = osArchitecture;
this.osImageType = osImageType;
this.minHddSize = minHddSize;
this.type = type;
this.state = state;
this.version = version;
this.categories = categories;
this.eulaUrl = eulaUrl;
}
@Override
public String id() {
return id;
}
@Override
public String name() {
return name;
}
@Nullable
@Override
public List availableDataCenters() {
return availableDataCenters;
}
@Nullable
@Override
public String osInstallationBase() {
return osInstallationBase;
}
@Nullable
@Override
public Types.OSFamliyType osFamily() {
return osFamily;
}
@Nullable
@Override
public String os() {
return os;
}
@Nullable
@Override
public String osVersion() {
return osVersion;
}
@Override
public int osArchitecture() {
return osArchitecture;
}
@Nullable
@Override
public Types.OSImageType osImageType() {
return osImageType;
}
@Override
public int minHddSize() {
return minHddSize;
}
@Nullable
@Override
public Types.ApplianceType type() {
return type;
}
@Nullable
@Override
public String state() {
return state;
}
@Nullable
@Override
public String version() {
return version;
}
@Nullable
@Override
public List categories() {
return categories;
}
@Nullable
@Override
public String eulaUrl() {
return eulaUrl;
}
@Override
public String toString() {
return "ServerAppliance{"
+ "id=" + id + ", "
+ "name=" + name + ", "
+ "availableDataCenters=" + availableDataCenters + ", "
+ "osInstallationBase=" + osInstallationBase + ", "
+ "osFamily=" + osFamily + ", "
+ "os=" + os + ", "
+ "osVersion=" + osVersion + ", "
+ "osArchitecture=" + osArchitecture + ", "
+ "osImageType=" + osImageType + ", "
+ "minHddSize=" + minHddSize + ", "
+ "type=" + type + ", "
+ "state=" + state + ", "
+ "version=" + version + ", "
+ "categories=" + categories + ", "
+ "eulaUrl=" + eulaUrl
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof ServerAppliance) {
ServerAppliance that = (ServerAppliance) o;
return (this.id.equals(that.id()))
&& (this.name.equals(that.name()))
&& ((this.availableDataCenters == null) ? (that.availableDataCenters() == null) : this.availableDataCenters.equals(that.availableDataCenters()))
&& ((this.osInstallationBase == null) ? (that.osInstallationBase() == null) : this.osInstallationBase.equals(that.osInstallationBase()))
&& ((this.osFamily == null) ? (that.osFamily() == null) : this.osFamily.equals(that.osFamily()))
&& ((this.os == null) ? (that.os() == null) : this.os.equals(that.os()))
&& ((this.osVersion == null) ? (that.osVersion() == null) : this.osVersion.equals(that.osVersion()))
&& (this.osArchitecture == that.osArchitecture())
&& ((this.osImageType == null) ? (that.osImageType() == null) : this.osImageType.equals(that.osImageType()))
&& (this.minHddSize == that.minHddSize())
&& ((this.type == null) ? (that.type() == null) : this.type.equals(that.type()))
&& ((this.state == null) ? (that.state() == null) : this.state.equals(that.state()))
&& ((this.version == null) ? (that.version() == null) : this.version.equals(that.version()))
&& ((this.categories == null) ? (that.categories() == null) : this.categories.equals(that.categories()))
&& ((this.eulaUrl == null) ? (that.eulaUrl() == null) : this.eulaUrl.equals(that.eulaUrl()));
}
return false;
}
@Override
public int hashCode() {
int h = 1;
h *= 1000003;
h ^= this.id.hashCode();
h *= 1000003;
h ^= this.name.hashCode();
h *= 1000003;
h ^= (availableDataCenters == null) ? 0 : this.availableDataCenters.hashCode();
h *= 1000003;
h ^= (osInstallationBase == null) ? 0 : this.osInstallationBase.hashCode();
h *= 1000003;
h ^= (osFamily == null) ? 0 : this.osFamily.hashCode();
h *= 1000003;
h ^= (os == null) ? 0 : this.os.hashCode();
h *= 1000003;
h ^= (osVersion == null) ? 0 : this.osVersion.hashCode();
h *= 1000003;
h ^= this.osArchitecture;
h *= 1000003;
h ^= (osImageType == null) ? 0 : this.osImageType.hashCode();
h *= 1000003;
h ^= this.minHddSize;
h *= 1000003;
h ^= (type == null) ? 0 : this.type.hashCode();
h *= 1000003;
h ^= (state == null) ? 0 : this.state.hashCode();
h *= 1000003;
h ^= (version == null) ? 0 : this.version.hashCode();
h *= 1000003;
h ^= (categories == null) ? 0 : this.categories.hashCode();
h *= 1000003;
h ^= (eulaUrl == null) ? 0 : this.eulaUrl.hashCode();
return h;
}
}