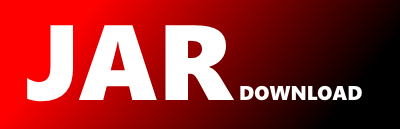
org.apache.jclouds.oneandone.rest.domain.AutoValue_Vpn Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jclouds-shaded Show documentation
Show all versions of jclouds-shaded Show documentation
Provides a shaded jclouds with relocated guava and guice
The newest version!
package org.apache.jclouds.oneandone.rest.domain;
import java.util.List;
import javax.annotation.Generated;
import org.jclouds.javax.annotation.Nullable;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_Vpn extends Vpn {
private final String id;
private final String name;
private final String description;
private final Types.GenericState state;
private final DataCenter datacenter;
private final Types.VPNType type;
private final String creationDate;
private final String cloudpanelId;
private final List ips;
AutoValue_Vpn(
String id,
String name,
@Nullable String description,
@Nullable Types.GenericState state,
@Nullable DataCenter datacenter,
@Nullable Types.VPNType type,
@Nullable String creationDate,
@Nullable String cloudpanelId,
@Nullable List ips) {
if (id == null) {
throw new NullPointerException("Null id");
}
this.id = id;
if (name == null) {
throw new NullPointerException("Null name");
}
this.name = name;
this.description = description;
this.state = state;
this.datacenter = datacenter;
this.type = type;
this.creationDate = creationDate;
this.cloudpanelId = cloudpanelId;
this.ips = ips;
}
@Override
public String id() {
return id;
}
@Override
public String name() {
return name;
}
@Nullable
@Override
public String description() {
return description;
}
@Nullable
@Override
public Types.GenericState state() {
return state;
}
@Nullable
@Override
public DataCenter datacenter() {
return datacenter;
}
@Nullable
@Override
public Types.VPNType type() {
return type;
}
@Nullable
@Override
public String creationDate() {
return creationDate;
}
@Nullable
@Override
public String cloudpanelId() {
return cloudpanelId;
}
@Nullable
@Override
public List ips() {
return ips;
}
@Override
public String toString() {
return "Vpn{"
+ "id=" + id + ", "
+ "name=" + name + ", "
+ "description=" + description + ", "
+ "state=" + state + ", "
+ "datacenter=" + datacenter + ", "
+ "type=" + type + ", "
+ "creationDate=" + creationDate + ", "
+ "cloudpanelId=" + cloudpanelId + ", "
+ "ips=" + ips
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof Vpn) {
Vpn that = (Vpn) o;
return (this.id.equals(that.id()))
&& (this.name.equals(that.name()))
&& ((this.description == null) ? (that.description() == null) : this.description.equals(that.description()))
&& ((this.state == null) ? (that.state() == null) : this.state.equals(that.state()))
&& ((this.datacenter == null) ? (that.datacenter() == null) : this.datacenter.equals(that.datacenter()))
&& ((this.type == null) ? (that.type() == null) : this.type.equals(that.type()))
&& ((this.creationDate == null) ? (that.creationDate() == null) : this.creationDate.equals(that.creationDate()))
&& ((this.cloudpanelId == null) ? (that.cloudpanelId() == null) : this.cloudpanelId.equals(that.cloudpanelId()))
&& ((this.ips == null) ? (that.ips() == null) : this.ips.equals(that.ips()));
}
return false;
}
@Override
public int hashCode() {
int h = 1;
h *= 1000003;
h ^= this.id.hashCode();
h *= 1000003;
h ^= this.name.hashCode();
h *= 1000003;
h ^= (description == null) ? 0 : this.description.hashCode();
h *= 1000003;
h ^= (state == null) ? 0 : this.state.hashCode();
h *= 1000003;
h ^= (datacenter == null) ? 0 : this.datacenter.hashCode();
h *= 1000003;
h ^= (type == null) ? 0 : this.type.hashCode();
h *= 1000003;
h ^= (creationDate == null) ? 0 : this.creationDate.hashCode();
h *= 1000003;
h ^= (cloudpanelId == null) ? 0 : this.cloudpanelId.hashCode();
h *= 1000003;
h ^= (ips == null) ? 0 : this.ips.hashCode();
return h;
}
}