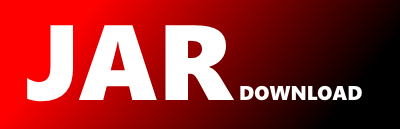
org.apache.jclouds.profitbricks.rest.compute.function.ProvisionableToImage Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jclouds-shaded Show documentation
Show all versions of jclouds-shaded Show documentation
Provides a shaded jclouds with relocated guava and guice
The newest version!
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.jclouds.profitbricks.rest.compute.function;
import static shaded.com.google.common.base.Preconditions.checkArgument;
import static shaded.com.google.common.base.Preconditions.checkNotNull;
import static shaded.com.google.common.collect.Iterables.find;
import static org.jclouds.location.predicates.LocationPredicates.idEquals;
import java.util.Set;
import java.util.regex.Pattern;
import org.apache.jclouds.profitbricks.rest.domain.LicenceType;
import org.apache.jclouds.profitbricks.rest.domain.Provisionable;
import org.apache.jclouds.profitbricks.rest.domain.Snapshot;
import org.jclouds.collect.Memoized;
import org.jclouds.compute.domain.Image;
import org.jclouds.compute.domain.ImageBuilder;
import org.jclouds.compute.domain.OperatingSystem;
import org.jclouds.compute.domain.OsFamily;
import org.jclouds.domain.Location;
import shaded.com.google.common.base.Function;
import shaded.com.google.common.base.Strings;
import shaded.com.google.common.base.Supplier;
import shaded.com.google.common.collect.ImmutableMap;
import shaded.com.google.inject.Inject;
public class ProvisionableToImage implements Function {
public static final String KEY_PROVISIONABLE_TYPE = "provisionableType";
private final ImageToImage fnImageToImage;
private final SnapshotToImage fnSnapshotToImage;
@Inject
ProvisionableToImage(@Memoized Supplier> locations) {
this.fnImageToImage = new ImageToImage(locations);
this.fnSnapshotToImage = new SnapshotToImage(locations);
}
@Override
public Image apply(Provisionable input) {
checkNotNull(input, "Cannot convert null input");
if (input instanceof org.apache.jclouds.profitbricks.rest.domain.Image) {
return fnImageToImage.apply((org.apache.jclouds.profitbricks.rest.domain.Image) input);
} else if (input instanceof Snapshot) {
return fnSnapshotToImage.apply((Snapshot) input);
} else {
throw new UnsupportedOperationException("No implementation found for provisionable of concrete type '"
+ input.getClass().getCanonicalName() + "'");
}
}
private static OsFamily mapOsFamily(LicenceType osType) {
if (osType == null) {
return OsFamily.UNRECOGNIZED;
}
switch (osType) {
case WINDOWS:
return OsFamily.WINDOWS;
case LINUX:
return OsFamily.LINUX;
case UNRECOGNIZED:
case OTHER:
default:
return OsFamily.UNRECOGNIZED;
}
}
private static class ImageToImage implements ImageFunction {
private static final Pattern HAS_NUMBERS = Pattern.compile(".*\\d+.*");
private final Supplier> locations;
ImageToImage(Supplier> locations) {
this.locations = locations;
}
@Override
public Image apply(org.apache.jclouds.profitbricks.rest.domain.Image from) {
String desc = from.properties().name();
OsFamily osFamily = parseOsFamily(desc, from.properties().licenceType());
Location location = find(locations.get(), idEquals(from.properties().location().getId()));
OperatingSystem os = OperatingSystem.builder()
.description(osFamily.value())
.family(osFamily)
.version(parseVersion(desc))
.is64Bit(is64Bit(desc, from.properties().imageType()))
.build();
return addTypeMetadata(new ImageBuilder()
.ids(from.id())
.name(desc)
.location(location)
.status(Image.Status.AVAILABLE)
.operatingSystem(os))
.build();
}
private OsFamily parseOsFamily(String from, LicenceType fallbackValue) {
if (from != null) {
try {
// ProfitBricks images names are usually in format:
// [osType]-[version]-[subversion]-..-[date-created]
String desc = from.toUpperCase().split("-")[0];
OsFamily osFamily = OsFamily.fromValue(desc);
checkArgument(osFamily != OsFamily.UNRECOGNIZED);
return osFamily;
} catch (Exception ex) {
// do nothing
}
}
return mapOsFamily(fallbackValue);
}
private String parseVersion(String from) {
if (from != null) {
String[] split = from.toLowerCase().split("-");
if (split.length >= 2) {
int i = 1; // usually on second token
String version = split[i];
while (!HAS_NUMBERS.matcher(version).matches()) {
version = split[++i];
}
return version;
}
}
return "";
}
private boolean is64Bit(String from, org.apache.jclouds.profitbricks.rest.domain.Image.Type type) {
switch (type) {
case CDROM:
if (!Strings.isNullOrEmpty(from)) {
return from.matches("x86_64|amd64");
}
case HDD: // HDD provided by ProfitBricks are always 64-bit
default:
return true;
}
}
@Override
public ImageBuilder addTypeMetadata(ImageBuilder builder) {
return builder.userMetadata(ImmutableMap.of(KEY_PROVISIONABLE_TYPE, Provisionable.Type.IMAGE.toString()));
}
}
private static class SnapshotToImage implements ImageFunction {
private final Supplier> locations;
SnapshotToImage(Supplier> locations) {
this.locations = locations;
}
@Override
public Image apply(Snapshot from) {
String textToParse = from.properties().name() + from.properties().description();
OsFamily osFamily = parseOsFamily(textToParse, from.properties().licenceType());
Location location = find(locations.get(), idEquals(from.properties().location().getId()));
OperatingSystem os = OperatingSystem.builder()
.description(osFamily.value())
.family(osFamily)
.is64Bit(true)
.version("00.00")
.build();
return addTypeMetadata(new ImageBuilder()
.ids(from.id())
.name(from.properties().name())
.description(from.properties().description())
.location(location)
.status(mapStatus(from.metadata().state()))
.operatingSystem(os))
.build();
}
private OsFamily parseOsFamily(String text, LicenceType fallbackValue) {
if (text != null) {
try {
// Attempt parsing OsFamily by scanning name and description
// @see ProfitBricksComputeServiceAdapter#L190
OsFamily[] families = OsFamily.values();
for (OsFamily family : families) {
if (text.contains(family.value())) {
return family;
}
}
} catch (Exception ex) {
// do nothing
}
}
return mapOsFamily(fallbackValue);
}
static Image.Status mapStatus(org.apache.jclouds.profitbricks.rest.domain.ProvisioningState state) {
if (state == null) {
return Image.Status.UNRECOGNIZED;
}
switch (state) {
case AVAILABLE:
return Image.Status.AVAILABLE;
case DELETED:
return Image.Status.DELETED;
case ERROR:
return Image.Status.ERROR;
case INACTIVE:
case INPROCESS:
return Image.Status.PENDING;
default:
return Image.Status.UNRECOGNIZED;
}
}
@Override
public ImageBuilder addTypeMetadata(ImageBuilder builder) {
return builder.userMetadata(ImmutableMap.of(KEY_PROVISIONABLE_TYPE, org.apache.jclouds.profitbricks.rest.domain.Provisionable.Type.SNAPSHOT.toString()));
}
}
private interface ImageFunction extends Function {
ImageBuilder addTypeMetadata(ImageBuilder builder);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy