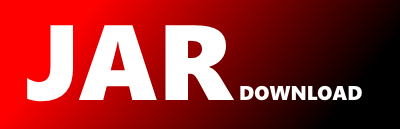
org.jclouds.azurecompute.arm.AzureComputeApi Maven / Gradle / Ivy
Show all versions of jclouds-shaded Show documentation
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.jclouds.azurecompute.arm;
import java.io.Closeable;
import javax.ws.rs.PathParam;
import org.jclouds.azurecompute.arm.domain.ServicePrincipal;
import org.jclouds.azurecompute.arm.features.AvailabilitySetApi;
import org.jclouds.azurecompute.arm.features.DeploymentApi;
import org.jclouds.azurecompute.arm.features.DiskApi;
import org.jclouds.azurecompute.arm.features.GraphRBACApi;
import org.jclouds.azurecompute.arm.features.ImageApi;
import org.jclouds.azurecompute.arm.features.JobApi;
import org.jclouds.azurecompute.arm.features.LoadBalancerApi;
import org.jclouds.azurecompute.arm.features.LocalNetworkGatewayApi;
import org.jclouds.azurecompute.arm.features.LocationApi;
import org.jclouds.azurecompute.arm.features.MetricDefinitionsApi;
import org.jclouds.azurecompute.arm.features.MetricsApi;
import org.jclouds.azurecompute.arm.features.NetworkInterfaceCardApi;
import org.jclouds.azurecompute.arm.features.NetworkSecurityGroupApi;
import org.jclouds.azurecompute.arm.features.NetworkSecurityRuleApi;
import org.jclouds.azurecompute.arm.features.OSImageApi;
import org.jclouds.azurecompute.arm.features.PublicIPAddressApi;
import org.jclouds.azurecompute.arm.features.ResourceGroupApi;
import org.jclouds.azurecompute.arm.features.ResourceProviderApi;
import org.jclouds.azurecompute.arm.features.StorageAccountApi;
import org.jclouds.azurecompute.arm.features.SubnetApi;
import org.jclouds.azurecompute.arm.features.VMSizeApi;
import org.jclouds.azurecompute.arm.features.VaultApi;
import org.jclouds.azurecompute.arm.features.VirtualMachineApi;
import org.jclouds.azurecompute.arm.features.VirtualMachineScaleSetApi;
import org.jclouds.azurecompute.arm.features.VirtualNetworkApi;
import org.jclouds.azurecompute.arm.features.VirtualNetworkGatewayApi;
import org.jclouds.azurecompute.arm.features.VirtualNetworkGatewayConnectionApi;
import org.jclouds.javax.annotation.Nullable;
import org.jclouds.rest.annotations.Delegate;
import shaded.com.google.common.base.Supplier;
import shaded.com.google.inject.Provides;
/**
* The Azure Resource Manager API is a REST API for managing your services and deployments.
*
*
* @see doc
*/
public interface AzureComputeApi extends Closeable {
/**
* The Azure Resource Manager API includes operations for managing resource groups in your subscription.
*
* @see docs
*/
@Delegate
ResourceGroupApi getResourceGroupApi();
/**
* Provides access to the Job tracking API.
*/
@Delegate
JobApi getJobApi();
/**
* This Azure Resource Manager API provides all of the locations that are available for resource providers
*
* @see docs
*/
@Delegate
LocationApi getLocationApi();
/**
* The Azure Resource Manager API includes operations for managing the storage accounts in your subscription.
*
* @see docs
*/
@Delegate
StorageAccountApi getStorageAccountApi(@PathParam("resourceGroup") String resourceGroup);
/**
* The Subnet API includes operations for managing the subnets in your virtual network.
*
* @see docs
*/
@Delegate
SubnetApi getSubnetApi(@PathParam("resourcegroup") String resourcegroup,
@PathParam("virtualnetwork") String virtualnetwork);
/**
* The Virtual Network API includes operations for managing the virtual networks in your subscription.
*
* @see docs
*/
@Delegate
VirtualNetworkApi getVirtualNetworkApi(@Nullable @PathParam("resourcegroup") String resourcegroup);
/**
* The Network Interface Card API includes operations for managing the NICs in your subscription.
*
* @see docs
*/
@Delegate
NetworkInterfaceCardApi getNetworkInterfaceCardApi(@Nullable @PathParam("resourcegroup") String resourcegroup);
/**
* The Public IP Address API includes operations for managing public ID Addresses for NICs in your subscription.
*
* @see docs
*/
@Delegate
PublicIPAddressApi getPublicIPAddressApi(@Nullable @PathParam("resourcegroup") String resourcegroup);
/**
* The Virtual Machine API includes operations for managing the virtual machines in your subscription.
*
* @see docs
*/
@Delegate
VirtualMachineApi getVirtualMachineApi(@Nullable @PathParam("resourceGroup") String resourceGroup);
/**
* The Virtual Machine Scale Set API includes operations for managing the virtual machines in your subscription.
*
* @see docs
*/
@Delegate
VirtualMachineScaleSetApi getVirtualMachineScaleSetApi(@PathParam("resourceGroup") String resourceGroup);
/**
* This Azure Resource Manager API lists all available virtual machine sizes for a subscription in a given region
*
* @see docs
*/
@Delegate
VMSizeApi getVMSizeApi(@PathParam("location") String location);
/**
* The Azure Resource Manager API gets all the OS images in your subscription.
*
* @see docs
*/
@Delegate
OSImageApi getOSImageApi(@PathParam("location") String location);
/**
* The Deployment API allows for the management of Azure Resource Manager resources through the use of templates.
*
* @see docs
*/
@Delegate
DeploymentApi getDeploymentApi(@PathParam("resourcegroup") String resourceGroup);
/**
* The NetworkSecurityGroup API includes operations for managing network security groups within your subscription.
*
* @see docs
*/
@Delegate
NetworkSecurityGroupApi getNetworkSecurityGroupApi(@Nullable @PathParam("resourcegroup") String resourcegroup);
/**
* The NetworkSecurityRule API includes operations for managing network security rules within a network security group.
*
* @see docs
*/
@Delegate
NetworkSecurityRuleApi getNetworkSecurityRuleApi(@PathParam("resourcegroup") String resourcegroup,
@PathParam("networksecuritygroup") String networksecuritygroup);
/**
* The LoadBalancer API includes operations for managing load balancers
* within your subscription.
*
* @see docs
*
*/
@Delegate
LoadBalancerApi getLoadBalancerApi(@Nullable @PathParam("resourcegroup") String resourcegroup);
/**
* The AvailabilitySet API includes operations for managing availability sets
* within your subscription.
*
* @see docs
*
*/
@Delegate
AvailabilitySetApi getAvailabilitySetApi(@PathParam("resourcegroup") String resourcegroup);
/**
* The Azure Resource Provider API provides information about a resource provider and its supported resource types.
*
* @see docs
*/
@Delegate
ResourceProviderApi getResourceProviderApi();
/**
* The ManagedDataDisk API includes operations for managing data disks within your subscription.
*
* @see docs
*/
@Delegate
DiskApi getDiskApi(@PathParam("resourcegroup") String resourcegroup);
/**
* The virtual machine image API includes operations for managing data disks within your subscription.
*
* @see docs
*/
@Delegate
ImageApi getVirtualMachineImageApi(@PathParam("resourcegroup") String resourcegroup);
/**
* The metrics API includes operations to get insights into entities within your
* subscription.
*
* @see docs
*/
@Delegate
MetricsApi getMetricsApi(@PathParam("resourceid") String resourceid);
/**
* The metric definitions API includes operations to get insights available for entities within your
* subscription.
*
* @see docs
*/
@Delegate
MetricDefinitionsApi getMetricsDefinitionsApi(@PathParam("resourceid") String resourceid);
/**
* The Azure Active Directory Graph API provides programmatic access to Azure
* AD through REST API endpoints.
*
* @see docs
*/
@Delegate
GraphRBACApi getGraphRBACApi();
/**
* Managing your key vaults as well as the keys, secrets, and certificates within your key vaults can be
* accomplished through a REST interface.
*
* @see docs
*/
@Delegate
VaultApi getVaultApi(@PathParam("resourcegroup") String resourcegroup);
/**
* Management features for Local Network Gateways.
*
* @see docs
*/
@Delegate
LocalNetworkGatewayApi getLocalNetworkGatewayApi(@PathParam("resourcegroup") String resourcegroup);
/**
* Management features for Virtual Network Gateways.
*
* @see docs
*/
@Delegate
VirtualNetworkGatewayApi getVirtualNetworkGatewayApi(@PathParam("resourcegroup") String resourcegroup);
/**
* Management features for Virtual Network Gateway Connections.
*
* @see docs
*/
@Delegate
VirtualNetworkGatewayConnectionApi getVirtualNetworkGatewayConnectionApi(@PathParam("resourcegroup") String resourcegroup);
/**
* Returns the information about the current service principal.
*/
@Provides
Supplier getServicePrincipal();
}