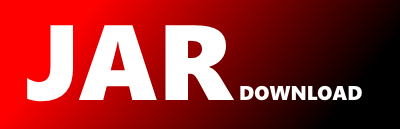
org.jclouds.azurecompute.arm.compute.options.AutoValue_IpOptions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jclouds-shaded Show documentation
Show all versions of jclouds-shaded Show documentation
Provides a shaded jclouds with relocated guava and guice
The newest version!
package org.jclouds.azurecompute.arm.compute.options;
import shaded.com.google.common.base.Optional;
import javax.annotation.Generated;
import org.jclouds.javax.annotation.Nullable;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_IpOptions extends IpOptions {
private final String subnet;
private final Optional address;
private final boolean allocateNewPublicIp;
private final String publicIpId;
private AutoValue_IpOptions(
String subnet,
Optional address,
boolean allocateNewPublicIp,
@Nullable String publicIpId) {
this.subnet = subnet;
this.address = address;
this.allocateNewPublicIp = allocateNewPublicIp;
this.publicIpId = publicIpId;
}
@Override
public String subnet() {
return subnet;
}
@Override
public Optional address() {
return address;
}
@Override
public boolean allocateNewPublicIp() {
return allocateNewPublicIp;
}
@Nullable
@Override
public String publicIpId() {
return publicIpId;
}
@Override
public String toString() {
return "IpOptions{"
+ "subnet=" + subnet + ", "
+ "address=" + address + ", "
+ "allocateNewPublicIp=" + allocateNewPublicIp + ", "
+ "publicIpId=" + publicIpId
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof IpOptions) {
IpOptions that = (IpOptions) o;
return (this.subnet.equals(that.subnet()))
&& (this.address.equals(that.address()))
&& (this.allocateNewPublicIp == that.allocateNewPublicIp())
&& ((this.publicIpId == null) ? (that.publicIpId() == null) : this.publicIpId.equals(that.publicIpId()));
}
return false;
}
@Override
public int hashCode() {
int h = 1;
h *= 1000003;
h ^= this.subnet.hashCode();
h *= 1000003;
h ^= this.address.hashCode();
h *= 1000003;
h ^= this.allocateNewPublicIp ? 1231 : 1237;
h *= 1000003;
h ^= (publicIpId == null) ? 0 : this.publicIpId.hashCode();
return h;
}
@Override
public IpOptions.Builder toBuilder() {
return new Builder(this);
}
static final class Builder extends IpOptions.Builder {
private String subnet;
private Optional address = Optional.absent();
private Boolean allocateNewPublicIp;
private String publicIpId;
Builder() {
}
private Builder(IpOptions source) {
this.subnet = source.subnet();
this.address = source.address();
this.allocateNewPublicIp = source.allocateNewPublicIp();
this.publicIpId = source.publicIpId();
}
@Override
public IpOptions.Builder subnet(String subnet) {
if (subnet == null) {
throw new NullPointerException("Null subnet");
}
this.subnet = subnet;
return this;
}
@Override
IpOptions.Builder address(Optional address) {
if (address == null) {
throw new NullPointerException("Null address");
}
this.address = address;
return this;
}
@Override
public IpOptions.Builder allocateNewPublicIp(boolean allocateNewPublicIp) {
this.allocateNewPublicIp = allocateNewPublicIp;
return this;
}
@Override
public IpOptions.Builder publicIpId(@Nullable String publicIpId) {
this.publicIpId = publicIpId;
return this;
}
@Override
public IpOptions build() {
String missing = "";
if (this.subnet == null) {
missing += " subnet";
}
if (this.allocateNewPublicIp == null) {
missing += " allocateNewPublicIp";
}
if (!missing.isEmpty()) {
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_IpOptions(
this.subnet,
this.address,
this.allocateNewPublicIp,
this.publicIpId);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy