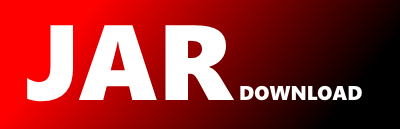
org.jclouds.azurecompute.arm.domain.AutoValue_Certificate_IssuerBundle Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jclouds-shaded Show documentation
Show all versions of jclouds-shaded Show documentation
Provides a shaded jclouds with relocated guava and guice
The newest version!
package org.jclouds.azurecompute.arm.domain;
import javax.annotation.Generated;
import org.jclouds.javax.annotation.Nullable;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_Certificate_IssuerBundle extends Certificate.IssuerBundle {
private final Certificate.IssuerAttributes attributes;
private final Certificate.IssuerCredentials credentials;
private final String id;
private final Certificate.OrganizationDetails organizationDetails;
private final String provider;
AutoValue_Certificate_IssuerBundle(
@Nullable Certificate.IssuerAttributes attributes,
@Nullable Certificate.IssuerCredentials credentials,
@Nullable String id,
@Nullable Certificate.OrganizationDetails organizationDetails,
@Nullable String provider) {
this.attributes = attributes;
this.credentials = credentials;
this.id = id;
this.organizationDetails = organizationDetails;
this.provider = provider;
}
@Nullable
@Override
public Certificate.IssuerAttributes attributes() {
return attributes;
}
@Nullable
@Override
public Certificate.IssuerCredentials credentials() {
return credentials;
}
@Nullable
@Override
public String id() {
return id;
}
@Nullable
@Override
public Certificate.OrganizationDetails organizationDetails() {
return organizationDetails;
}
@Nullable
@Override
public String provider() {
return provider;
}
@Override
public String toString() {
return "IssuerBundle{"
+ "attributes=" + attributes + ", "
+ "credentials=" + credentials + ", "
+ "id=" + id + ", "
+ "organizationDetails=" + organizationDetails + ", "
+ "provider=" + provider
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof Certificate.IssuerBundle) {
Certificate.IssuerBundle that = (Certificate.IssuerBundle) o;
return ((this.attributes == null) ? (that.attributes() == null) : this.attributes.equals(that.attributes()))
&& ((this.credentials == null) ? (that.credentials() == null) : this.credentials.equals(that.credentials()))
&& ((this.id == null) ? (that.id() == null) : this.id.equals(that.id()))
&& ((this.organizationDetails == null) ? (that.organizationDetails() == null) : this.organizationDetails.equals(that.organizationDetails()))
&& ((this.provider == null) ? (that.provider() == null) : this.provider.equals(that.provider()));
}
return false;
}
@Override
public int hashCode() {
int h = 1;
h *= 1000003;
h ^= (attributes == null) ? 0 : this.attributes.hashCode();
h *= 1000003;
h ^= (credentials == null) ? 0 : this.credentials.hashCode();
h *= 1000003;
h ^= (id == null) ? 0 : this.id.hashCode();
h *= 1000003;
h ^= (organizationDetails == null) ? 0 : this.organizationDetails.hashCode();
h *= 1000003;
h ^= (provider == null) ? 0 : this.provider.hashCode();
return h;
}
}