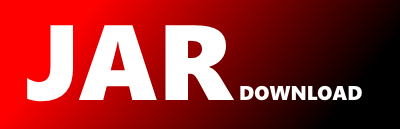
org.jclouds.azurecompute.arm.domain.AutoValue_DeploymentTemplate Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jclouds-shaded Show documentation
Show all versions of jclouds-shaded Show documentation
Provides a shaded jclouds with relocated guava and guice
The newest version!
package org.jclouds.azurecompute.arm.domain;
import java.util.List;
import java.util.Map;
import javax.annotation.Generated;
import org.jclouds.javax.annotation.Nullable;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_DeploymentTemplate extends DeploymentTemplate {
private final String schema;
private final String contentVersion;
private final DeploymentTemplate.TemplateParameters parameters;
private final Map variables;
private final List resources;
private final List> outputs;
private AutoValue_DeploymentTemplate(
String schema,
String contentVersion,
DeploymentTemplate.TemplateParameters parameters,
Map variables,
List resources,
@Nullable List> outputs) {
this.schema = schema;
this.contentVersion = contentVersion;
this.parameters = parameters;
this.variables = variables;
this.resources = resources;
this.outputs = outputs;
}
@Override
public String schema() {
return schema;
}
@Override
public String contentVersion() {
return contentVersion;
}
@Override
public DeploymentTemplate.TemplateParameters parameters() {
return parameters;
}
@Override
public Map variables() {
return variables;
}
@Override
public List resources() {
return resources;
}
@Nullable
@Override
public List> outputs() {
return outputs;
}
@Override
public String toString() {
return "DeploymentTemplate{"
+ "schema=" + schema + ", "
+ "contentVersion=" + contentVersion + ", "
+ "parameters=" + parameters + ", "
+ "variables=" + variables + ", "
+ "resources=" + resources + ", "
+ "outputs=" + outputs
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof DeploymentTemplate) {
DeploymentTemplate that = (DeploymentTemplate) o;
return (this.schema.equals(that.schema()))
&& (this.contentVersion.equals(that.contentVersion()))
&& (this.parameters.equals(that.parameters()))
&& (this.variables.equals(that.variables()))
&& (this.resources.equals(that.resources()))
&& ((this.outputs == null) ? (that.outputs() == null) : this.outputs.equals(that.outputs()));
}
return false;
}
@Override
public int hashCode() {
int h = 1;
h *= 1000003;
h ^= this.schema.hashCode();
h *= 1000003;
h ^= this.contentVersion.hashCode();
h *= 1000003;
h ^= this.parameters.hashCode();
h *= 1000003;
h ^= this.variables.hashCode();
h *= 1000003;
h ^= this.resources.hashCode();
h *= 1000003;
h ^= (outputs == null) ? 0 : this.outputs.hashCode();
return h;
}
@Override
public DeploymentTemplate.Builder toBuilder() {
return new Builder(this);
}
static final class Builder extends DeploymentTemplate.Builder {
private String schema;
private String contentVersion;
private DeploymentTemplate.TemplateParameters parameters;
private Map variables;
private List resources;
private List> outputs;
Builder() {
}
private Builder(DeploymentTemplate source) {
this.schema = source.schema();
this.contentVersion = source.contentVersion();
this.parameters = source.parameters();
this.variables = source.variables();
this.resources = source.resources();
this.outputs = source.outputs();
}
@Override
public DeploymentTemplate.Builder schema(String schema) {
if (schema == null) {
throw new NullPointerException("Null schema");
}
this.schema = schema;
return this;
}
@Override
public DeploymentTemplate.Builder contentVersion(String contentVersion) {
if (contentVersion == null) {
throw new NullPointerException("Null contentVersion");
}
this.contentVersion = contentVersion;
return this;
}
@Override
public DeploymentTemplate.Builder parameters(DeploymentTemplate.TemplateParameters parameters) {
if (parameters == null) {
throw new NullPointerException("Null parameters");
}
this.parameters = parameters;
return this;
}
@Override
public DeploymentTemplate.Builder variables(Map variables) {
if (variables == null) {
throw new NullPointerException("Null variables");
}
this.variables = variables;
return this;
}
@Override
Map variables() {
if (variables == null) {
throw new IllegalStateException("Property \"variables\" has not been set");
}
return variables;
}
@Override
public DeploymentTemplate.Builder resources(List resources) {
if (resources == null) {
throw new NullPointerException("Null resources");
}
this.resources = resources;
return this;
}
@Override
List resources() {
if (resources == null) {
throw new IllegalStateException("Property \"resources\" has not been set");
}
return resources;
}
@Override
public DeploymentTemplate.Builder outputs(@Nullable List> outputs) {
this.outputs = outputs;
return this;
}
@Override
DeploymentTemplate autoBuild() {
String missing = "";
if (this.schema == null) {
missing += " schema";
}
if (this.contentVersion == null) {
missing += " contentVersion";
}
if (this.parameters == null) {
missing += " parameters";
}
if (this.variables == null) {
missing += " variables";
}
if (this.resources == null) {
missing += " resources";
}
if (!missing.isEmpty()) {
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_DeploymentTemplate(
this.schema,
this.contentVersion,
this.parameters,
this.variables,
this.resources,
this.outputs);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy