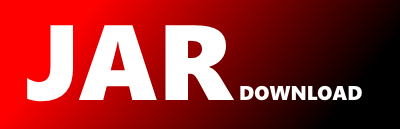
org.jclouds.azurecompute.arm.domain.AutoValue_InboundNatRuleProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jclouds-shaded Show documentation
Show all versions of jclouds-shaded Show documentation
Provides a shaded jclouds with relocated guava and guice
The newest version!
package org.jclouds.azurecompute.arm.domain;
import javax.annotation.Generated;
import org.jclouds.javax.annotation.Nullable;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_InboundNatRuleProperties extends InboundNatRuleProperties {
private final IdReference frontendIPConfiguration;
private final IdReference backendIPConfiguration;
private final InboundNatRuleProperties.Protocol protocol;
private final int backendPort;
private final int frontendPort;
private final Boolean enableFloatingIP;
private final Integer idleTimeoutInMinutes;
private final String provisioningState;
private AutoValue_InboundNatRuleProperties(
@Nullable IdReference frontendIPConfiguration,
@Nullable IdReference backendIPConfiguration,
InboundNatRuleProperties.Protocol protocol,
int backendPort,
int frontendPort,
@Nullable Boolean enableFloatingIP,
@Nullable Integer idleTimeoutInMinutes,
@Nullable String provisioningState) {
this.frontendIPConfiguration = frontendIPConfiguration;
this.backendIPConfiguration = backendIPConfiguration;
this.protocol = protocol;
this.backendPort = backendPort;
this.frontendPort = frontendPort;
this.enableFloatingIP = enableFloatingIP;
this.idleTimeoutInMinutes = idleTimeoutInMinutes;
this.provisioningState = provisioningState;
}
@Nullable
@Override
public IdReference frontendIPConfiguration() {
return frontendIPConfiguration;
}
@Nullable
@Override
public IdReference backendIPConfiguration() {
return backendIPConfiguration;
}
@Override
public InboundNatRuleProperties.Protocol protocol() {
return protocol;
}
@Override
public int backendPort() {
return backendPort;
}
@Override
public int frontendPort() {
return frontendPort;
}
@Nullable
@Override
public Boolean enableFloatingIP() {
return enableFloatingIP;
}
@Nullable
@Override
public Integer idleTimeoutInMinutes() {
return idleTimeoutInMinutes;
}
@Nullable
@Override
public String provisioningState() {
return provisioningState;
}
@Override
public String toString() {
return "InboundNatRuleProperties{"
+ "frontendIPConfiguration=" + frontendIPConfiguration + ", "
+ "backendIPConfiguration=" + backendIPConfiguration + ", "
+ "protocol=" + protocol + ", "
+ "backendPort=" + backendPort + ", "
+ "frontendPort=" + frontendPort + ", "
+ "enableFloatingIP=" + enableFloatingIP + ", "
+ "idleTimeoutInMinutes=" + idleTimeoutInMinutes + ", "
+ "provisioningState=" + provisioningState
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof InboundNatRuleProperties) {
InboundNatRuleProperties that = (InboundNatRuleProperties) o;
return ((this.frontendIPConfiguration == null) ? (that.frontendIPConfiguration() == null) : this.frontendIPConfiguration.equals(that.frontendIPConfiguration()))
&& ((this.backendIPConfiguration == null) ? (that.backendIPConfiguration() == null) : this.backendIPConfiguration.equals(that.backendIPConfiguration()))
&& (this.protocol.equals(that.protocol()))
&& (this.backendPort == that.backendPort())
&& (this.frontendPort == that.frontendPort())
&& ((this.enableFloatingIP == null) ? (that.enableFloatingIP() == null) : this.enableFloatingIP.equals(that.enableFloatingIP()))
&& ((this.idleTimeoutInMinutes == null) ? (that.idleTimeoutInMinutes() == null) : this.idleTimeoutInMinutes.equals(that.idleTimeoutInMinutes()))
&& ((this.provisioningState == null) ? (that.provisioningState() == null) : this.provisioningState.equals(that.provisioningState()));
}
return false;
}
@Override
public int hashCode() {
int h = 1;
h *= 1000003;
h ^= (frontendIPConfiguration == null) ? 0 : this.frontendIPConfiguration.hashCode();
h *= 1000003;
h ^= (backendIPConfiguration == null) ? 0 : this.backendIPConfiguration.hashCode();
h *= 1000003;
h ^= this.protocol.hashCode();
h *= 1000003;
h ^= this.backendPort;
h *= 1000003;
h ^= this.frontendPort;
h *= 1000003;
h ^= (enableFloatingIP == null) ? 0 : this.enableFloatingIP.hashCode();
h *= 1000003;
h ^= (idleTimeoutInMinutes == null) ? 0 : this.idleTimeoutInMinutes.hashCode();
h *= 1000003;
h ^= (provisioningState == null) ? 0 : this.provisioningState.hashCode();
return h;
}
@Override
public InboundNatRuleProperties.Builder toBuilder() {
return new Builder(this);
}
static final class Builder extends InboundNatRuleProperties.Builder {
private IdReference frontendIPConfiguration;
private IdReference backendIPConfiguration;
private InboundNatRuleProperties.Protocol protocol;
private Integer backendPort;
private Integer frontendPort;
private Boolean enableFloatingIP;
private Integer idleTimeoutInMinutes;
private String provisioningState;
Builder() {
}
private Builder(InboundNatRuleProperties source) {
this.frontendIPConfiguration = source.frontendIPConfiguration();
this.backendIPConfiguration = source.backendIPConfiguration();
this.protocol = source.protocol();
this.backendPort = source.backendPort();
this.frontendPort = source.frontendPort();
this.enableFloatingIP = source.enableFloatingIP();
this.idleTimeoutInMinutes = source.idleTimeoutInMinutes();
this.provisioningState = source.provisioningState();
}
@Override
public InboundNatRuleProperties.Builder frontendIPConfiguration(@Nullable IdReference frontendIPConfiguration) {
this.frontendIPConfiguration = frontendIPConfiguration;
return this;
}
@Override
public InboundNatRuleProperties.Builder backendIPConfiguration(@Nullable IdReference backendIPConfiguration) {
this.backendIPConfiguration = backendIPConfiguration;
return this;
}
@Override
public InboundNatRuleProperties.Builder protocol(InboundNatRuleProperties.Protocol protocol) {
if (protocol == null) {
throw new NullPointerException("Null protocol");
}
this.protocol = protocol;
return this;
}
@Override
public InboundNatRuleProperties.Builder backendPort(int backendPort) {
this.backendPort = backendPort;
return this;
}
@Override
public InboundNatRuleProperties.Builder frontendPort(int frontendPort) {
this.frontendPort = frontendPort;
return this;
}
@Override
public InboundNatRuleProperties.Builder enableFloatingIP(@Nullable Boolean enableFloatingIP) {
this.enableFloatingIP = enableFloatingIP;
return this;
}
@Override
public InboundNatRuleProperties.Builder idleTimeoutInMinutes(@Nullable Integer idleTimeoutInMinutes) {
this.idleTimeoutInMinutes = idleTimeoutInMinutes;
return this;
}
@Override
public InboundNatRuleProperties.Builder provisioningState(@Nullable String provisioningState) {
this.provisioningState = provisioningState;
return this;
}
@Override
public InboundNatRuleProperties build() {
String missing = "";
if (this.protocol == null) {
missing += " protocol";
}
if (this.backendPort == null) {
missing += " backendPort";
}
if (this.frontendPort == null) {
missing += " frontendPort";
}
if (!missing.isEmpty()) {
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_InboundNatRuleProperties(
this.frontendIPConfiguration,
this.backendIPConfiguration,
this.protocol,
this.backendPort,
this.frontendPort,
this.enableFloatingIP,
this.idleTimeoutInMinutes,
this.provisioningState);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy