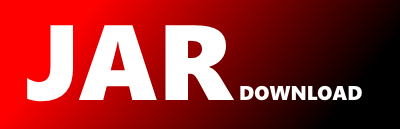
org.jclouds.azurecompute.arm.domain.AutoValue_IpConfigurationProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jclouds-shaded Show documentation
Show all versions of jclouds-shaded Show documentation
Provides a shaded jclouds with relocated guava and guice
The newest version!
package org.jclouds.azurecompute.arm.domain;
import java.util.List;
import javax.annotation.Generated;
import org.jclouds.javax.annotation.Nullable;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_IpConfigurationProperties extends IpConfigurationProperties {
private final String provisioningState;
private final String privateIPAddress;
private final String privateIPAllocationMethod;
private final IdReference subnet;
private final IdReference publicIPAddress;
private final List loadBalancerBackendAddressPools;
private final List loadBalancerInboundNatRules;
private final Boolean primary;
private AutoValue_IpConfigurationProperties(
@Nullable String provisioningState,
@Nullable String privateIPAddress,
@Nullable String privateIPAllocationMethod,
@Nullable IdReference subnet,
@Nullable IdReference publicIPAddress,
@Nullable List loadBalancerBackendAddressPools,
@Nullable List loadBalancerInboundNatRules,
@Nullable Boolean primary) {
this.provisioningState = provisioningState;
this.privateIPAddress = privateIPAddress;
this.privateIPAllocationMethod = privateIPAllocationMethod;
this.subnet = subnet;
this.publicIPAddress = publicIPAddress;
this.loadBalancerBackendAddressPools = loadBalancerBackendAddressPools;
this.loadBalancerInboundNatRules = loadBalancerInboundNatRules;
this.primary = primary;
}
@Nullable
@Override
public String provisioningState() {
return provisioningState;
}
@Nullable
@Override
public String privateIPAddress() {
return privateIPAddress;
}
@Nullable
@Override
public String privateIPAllocationMethod() {
return privateIPAllocationMethod;
}
@Nullable
@Override
public IdReference subnet() {
return subnet;
}
@Nullable
@Override
public IdReference publicIPAddress() {
return publicIPAddress;
}
@Nullable
@Override
public List loadBalancerBackendAddressPools() {
return loadBalancerBackendAddressPools;
}
@Nullable
@Override
public List loadBalancerInboundNatRules() {
return loadBalancerInboundNatRules;
}
@Nullable
@Override
public Boolean primary() {
return primary;
}
@Override
public String toString() {
return "IpConfigurationProperties{"
+ "provisioningState=" + provisioningState + ", "
+ "privateIPAddress=" + privateIPAddress + ", "
+ "privateIPAllocationMethod=" + privateIPAllocationMethod + ", "
+ "subnet=" + subnet + ", "
+ "publicIPAddress=" + publicIPAddress + ", "
+ "loadBalancerBackendAddressPools=" + loadBalancerBackendAddressPools + ", "
+ "loadBalancerInboundNatRules=" + loadBalancerInboundNatRules + ", "
+ "primary=" + primary
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof IpConfigurationProperties) {
IpConfigurationProperties that = (IpConfigurationProperties) o;
return ((this.provisioningState == null) ? (that.provisioningState() == null) : this.provisioningState.equals(that.provisioningState()))
&& ((this.privateIPAddress == null) ? (that.privateIPAddress() == null) : this.privateIPAddress.equals(that.privateIPAddress()))
&& ((this.privateIPAllocationMethod == null) ? (that.privateIPAllocationMethod() == null) : this.privateIPAllocationMethod.equals(that.privateIPAllocationMethod()))
&& ((this.subnet == null) ? (that.subnet() == null) : this.subnet.equals(that.subnet()))
&& ((this.publicIPAddress == null) ? (that.publicIPAddress() == null) : this.publicIPAddress.equals(that.publicIPAddress()))
&& ((this.loadBalancerBackendAddressPools == null) ? (that.loadBalancerBackendAddressPools() == null) : this.loadBalancerBackendAddressPools.equals(that.loadBalancerBackendAddressPools()))
&& ((this.loadBalancerInboundNatRules == null) ? (that.loadBalancerInboundNatRules() == null) : this.loadBalancerInboundNatRules.equals(that.loadBalancerInboundNatRules()))
&& ((this.primary == null) ? (that.primary() == null) : this.primary.equals(that.primary()));
}
return false;
}
@Override
public int hashCode() {
int h = 1;
h *= 1000003;
h ^= (provisioningState == null) ? 0 : this.provisioningState.hashCode();
h *= 1000003;
h ^= (privateIPAddress == null) ? 0 : this.privateIPAddress.hashCode();
h *= 1000003;
h ^= (privateIPAllocationMethod == null) ? 0 : this.privateIPAllocationMethod.hashCode();
h *= 1000003;
h ^= (subnet == null) ? 0 : this.subnet.hashCode();
h *= 1000003;
h ^= (publicIPAddress == null) ? 0 : this.publicIPAddress.hashCode();
h *= 1000003;
h ^= (loadBalancerBackendAddressPools == null) ? 0 : this.loadBalancerBackendAddressPools.hashCode();
h *= 1000003;
h ^= (loadBalancerInboundNatRules == null) ? 0 : this.loadBalancerInboundNatRules.hashCode();
h *= 1000003;
h ^= (primary == null) ? 0 : this.primary.hashCode();
return h;
}
@Override
public IpConfigurationProperties.Builder toBuilder() {
return new Builder(this);
}
static final class Builder extends IpConfigurationProperties.Builder {
private String provisioningState;
private String privateIPAddress;
private String privateIPAllocationMethod;
private IdReference subnet;
private IdReference publicIPAddress;
private List loadBalancerBackendAddressPools;
private List loadBalancerInboundNatRules;
private Boolean primary;
Builder() {
}
private Builder(IpConfigurationProperties source) {
this.provisioningState = source.provisioningState();
this.privateIPAddress = source.privateIPAddress();
this.privateIPAllocationMethod = source.privateIPAllocationMethod();
this.subnet = source.subnet();
this.publicIPAddress = source.publicIPAddress();
this.loadBalancerBackendAddressPools = source.loadBalancerBackendAddressPools();
this.loadBalancerInboundNatRules = source.loadBalancerInboundNatRules();
this.primary = source.primary();
}
@Override
public IpConfigurationProperties.Builder provisioningState(@Nullable String provisioningState) {
this.provisioningState = provisioningState;
return this;
}
@Override
public IpConfigurationProperties.Builder privateIPAddress(@Nullable String privateIPAddress) {
this.privateIPAddress = privateIPAddress;
return this;
}
@Override
public IpConfigurationProperties.Builder privateIPAllocationMethod(@Nullable String privateIPAllocationMethod) {
this.privateIPAllocationMethod = privateIPAllocationMethod;
return this;
}
@Override
public IpConfigurationProperties.Builder subnet(@Nullable IdReference subnet) {
this.subnet = subnet;
return this;
}
@Override
public IpConfigurationProperties.Builder publicIPAddress(@Nullable IdReference publicIPAddress) {
this.publicIPAddress = publicIPAddress;
return this;
}
@Override
public IpConfigurationProperties.Builder loadBalancerBackendAddressPools(@Nullable List loadBalancerBackendAddressPools) {
this.loadBalancerBackendAddressPools = loadBalancerBackendAddressPools;
return this;
}
@Override
@Nullable List loadBalancerBackendAddressPools() {
return loadBalancerBackendAddressPools;
}
@Override
public IpConfigurationProperties.Builder loadBalancerInboundNatRules(@Nullable List loadBalancerInboundNatRules) {
this.loadBalancerInboundNatRules = loadBalancerInboundNatRules;
return this;
}
@Override
@Nullable List loadBalancerInboundNatRules() {
return loadBalancerInboundNatRules;
}
@Override
public IpConfigurationProperties.Builder primary(@Nullable Boolean primary) {
this.primary = primary;
return this;
}
@Override
IpConfigurationProperties autoBuild() {
return new AutoValue_IpConfigurationProperties(
this.provisioningState,
this.privateIPAddress,
this.privateIPAllocationMethod,
this.subnet,
this.publicIPAddress,
this.loadBalancerBackendAddressPools,
this.loadBalancerInboundNatRules,
this.primary);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy