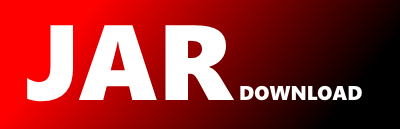
org.jclouds.azurecompute.arm.domain.AutoValue_Key_KeyAttributes Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jclouds-shaded Show documentation
Show all versions of jclouds-shaded Show documentation
Provides a shaded jclouds with relocated guava and guice
The newest version!
package org.jclouds.azurecompute.arm.domain;
import javax.annotation.Generated;
import org.jclouds.javax.annotation.Nullable;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_Key_KeyAttributes extends Key.KeyAttributes {
private final Boolean enabled;
private final Integer created;
private final Integer expires;
private final Integer notBefore;
private final String recoveryLevel;
private final Integer updated;
AutoValue_Key_KeyAttributes(
@Nullable Boolean enabled,
@Nullable Integer created,
@Nullable Integer expires,
@Nullable Integer notBefore,
@Nullable String recoveryLevel,
@Nullable Integer updated) {
this.enabled = enabled;
this.created = created;
this.expires = expires;
this.notBefore = notBefore;
this.recoveryLevel = recoveryLevel;
this.updated = updated;
}
@Nullable
@Override
public Boolean enabled() {
return enabled;
}
@Nullable
@Override
public Integer created() {
return created;
}
@Nullable
@Override
public Integer expires() {
return expires;
}
@Nullable
@Override
public Integer notBefore() {
return notBefore;
}
@Nullable
@Override
public String recoveryLevel() {
return recoveryLevel;
}
@Nullable
@Override
public Integer updated() {
return updated;
}
@Override
public String toString() {
return "KeyAttributes{"
+ "enabled=" + enabled + ", "
+ "created=" + created + ", "
+ "expires=" + expires + ", "
+ "notBefore=" + notBefore + ", "
+ "recoveryLevel=" + recoveryLevel + ", "
+ "updated=" + updated
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof Key.KeyAttributes) {
Key.KeyAttributes that = (Key.KeyAttributes) o;
return ((this.enabled == null) ? (that.enabled() == null) : this.enabled.equals(that.enabled()))
&& ((this.created == null) ? (that.created() == null) : this.created.equals(that.created()))
&& ((this.expires == null) ? (that.expires() == null) : this.expires.equals(that.expires()))
&& ((this.notBefore == null) ? (that.notBefore() == null) : this.notBefore.equals(that.notBefore()))
&& ((this.recoveryLevel == null) ? (that.recoveryLevel() == null) : this.recoveryLevel.equals(that.recoveryLevel()))
&& ((this.updated == null) ? (that.updated() == null) : this.updated.equals(that.updated()));
}
return false;
}
@Override
public int hashCode() {
int h = 1;
h *= 1000003;
h ^= (enabled == null) ? 0 : this.enabled.hashCode();
h *= 1000003;
h ^= (created == null) ? 0 : this.created.hashCode();
h *= 1000003;
h ^= (expires == null) ? 0 : this.expires.hashCode();
h *= 1000003;
h ^= (notBefore == null) ? 0 : this.notBefore.hashCode();
h *= 1000003;
h ^= (recoveryLevel == null) ? 0 : this.recoveryLevel.hashCode();
h *= 1000003;
h ^= (updated == null) ? 0 : this.updated.hashCode();
return h;
}
}