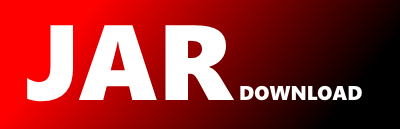
org.jclouds.azurecompute.arm.domain.AutoValue_NetworkSecurityRuleProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jclouds-shaded Show documentation
Show all versions of jclouds-shaded Show documentation
Provides a shaded jclouds with relocated guava and guice
The newest version!
package org.jclouds.azurecompute.arm.domain;
import javax.annotation.Generated;
import org.jclouds.javax.annotation.Nullable;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_NetworkSecurityRuleProperties extends NetworkSecurityRuleProperties {
private final String description;
private final NetworkSecurityRuleProperties.Protocol protocol;
private final String sourcePortRange;
private final String destinationPortRange;
private final String sourceAddressPrefix;
private final String destinationAddressPrefix;
private final NetworkSecurityRuleProperties.Access access;
private final Integer priority;
private final NetworkSecurityRuleProperties.Direction direction;
private final String provisioningState;
private AutoValue_NetworkSecurityRuleProperties(
@Nullable String description,
NetworkSecurityRuleProperties.Protocol protocol,
@Nullable String sourcePortRange,
@Nullable String destinationPortRange,
String sourceAddressPrefix,
String destinationAddressPrefix,
NetworkSecurityRuleProperties.Access access,
@Nullable Integer priority,
NetworkSecurityRuleProperties.Direction direction,
@Nullable String provisioningState) {
this.description = description;
this.protocol = protocol;
this.sourcePortRange = sourcePortRange;
this.destinationPortRange = destinationPortRange;
this.sourceAddressPrefix = sourceAddressPrefix;
this.destinationAddressPrefix = destinationAddressPrefix;
this.access = access;
this.priority = priority;
this.direction = direction;
this.provisioningState = provisioningState;
}
@Nullable
@Override
public String description() {
return description;
}
@Override
public NetworkSecurityRuleProperties.Protocol protocol() {
return protocol;
}
@Nullable
@Override
public String sourcePortRange() {
return sourcePortRange;
}
@Nullable
@Override
public String destinationPortRange() {
return destinationPortRange;
}
@Override
public String sourceAddressPrefix() {
return sourceAddressPrefix;
}
@Override
public String destinationAddressPrefix() {
return destinationAddressPrefix;
}
@Override
public NetworkSecurityRuleProperties.Access access() {
return access;
}
@Nullable
@Override
public Integer priority() {
return priority;
}
@Override
public NetworkSecurityRuleProperties.Direction direction() {
return direction;
}
@Nullable
@Override
public String provisioningState() {
return provisioningState;
}
@Override
public String toString() {
return "NetworkSecurityRuleProperties{"
+ "description=" + description + ", "
+ "protocol=" + protocol + ", "
+ "sourcePortRange=" + sourcePortRange + ", "
+ "destinationPortRange=" + destinationPortRange + ", "
+ "sourceAddressPrefix=" + sourceAddressPrefix + ", "
+ "destinationAddressPrefix=" + destinationAddressPrefix + ", "
+ "access=" + access + ", "
+ "priority=" + priority + ", "
+ "direction=" + direction + ", "
+ "provisioningState=" + provisioningState
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof NetworkSecurityRuleProperties) {
NetworkSecurityRuleProperties that = (NetworkSecurityRuleProperties) o;
return ((this.description == null) ? (that.description() == null) : this.description.equals(that.description()))
&& (this.protocol.equals(that.protocol()))
&& ((this.sourcePortRange == null) ? (that.sourcePortRange() == null) : this.sourcePortRange.equals(that.sourcePortRange()))
&& ((this.destinationPortRange == null) ? (that.destinationPortRange() == null) : this.destinationPortRange.equals(that.destinationPortRange()))
&& (this.sourceAddressPrefix.equals(that.sourceAddressPrefix()))
&& (this.destinationAddressPrefix.equals(that.destinationAddressPrefix()))
&& (this.access.equals(that.access()))
&& ((this.priority == null) ? (that.priority() == null) : this.priority.equals(that.priority()))
&& (this.direction.equals(that.direction()))
&& ((this.provisioningState == null) ? (that.provisioningState() == null) : this.provisioningState.equals(that.provisioningState()));
}
return false;
}
@Override
public int hashCode() {
int h = 1;
h *= 1000003;
h ^= (description == null) ? 0 : this.description.hashCode();
h *= 1000003;
h ^= this.protocol.hashCode();
h *= 1000003;
h ^= (sourcePortRange == null) ? 0 : this.sourcePortRange.hashCode();
h *= 1000003;
h ^= (destinationPortRange == null) ? 0 : this.destinationPortRange.hashCode();
h *= 1000003;
h ^= this.sourceAddressPrefix.hashCode();
h *= 1000003;
h ^= this.destinationAddressPrefix.hashCode();
h *= 1000003;
h ^= this.access.hashCode();
h *= 1000003;
h ^= (priority == null) ? 0 : this.priority.hashCode();
h *= 1000003;
h ^= this.direction.hashCode();
h *= 1000003;
h ^= (provisioningState == null) ? 0 : this.provisioningState.hashCode();
return h;
}
@Override
public NetworkSecurityRuleProperties.Builder toBuilder() {
return new Builder(this);
}
static final class Builder extends NetworkSecurityRuleProperties.Builder {
private String description;
private NetworkSecurityRuleProperties.Protocol protocol;
private String sourcePortRange;
private String destinationPortRange;
private String sourceAddressPrefix;
private String destinationAddressPrefix;
private NetworkSecurityRuleProperties.Access access;
private Integer priority;
private NetworkSecurityRuleProperties.Direction direction;
private String provisioningState;
Builder() {
}
private Builder(NetworkSecurityRuleProperties source) {
this.description = source.description();
this.protocol = source.protocol();
this.sourcePortRange = source.sourcePortRange();
this.destinationPortRange = source.destinationPortRange();
this.sourceAddressPrefix = source.sourceAddressPrefix();
this.destinationAddressPrefix = source.destinationAddressPrefix();
this.access = source.access();
this.priority = source.priority();
this.direction = source.direction();
this.provisioningState = source.provisioningState();
}
@Override
public NetworkSecurityRuleProperties.Builder description(@Nullable String description) {
this.description = description;
return this;
}
@Override
public NetworkSecurityRuleProperties.Builder protocol(NetworkSecurityRuleProperties.Protocol protocol) {
if (protocol == null) {
throw new NullPointerException("Null protocol");
}
this.protocol = protocol;
return this;
}
@Override
public NetworkSecurityRuleProperties.Builder sourcePortRange(@Nullable String sourcePortRange) {
this.sourcePortRange = sourcePortRange;
return this;
}
@Override
public NetworkSecurityRuleProperties.Builder destinationPortRange(@Nullable String destinationPortRange) {
this.destinationPortRange = destinationPortRange;
return this;
}
@Override
public NetworkSecurityRuleProperties.Builder sourceAddressPrefix(String sourceAddressPrefix) {
if (sourceAddressPrefix == null) {
throw new NullPointerException("Null sourceAddressPrefix");
}
this.sourceAddressPrefix = sourceAddressPrefix;
return this;
}
@Override
public NetworkSecurityRuleProperties.Builder destinationAddressPrefix(String destinationAddressPrefix) {
if (destinationAddressPrefix == null) {
throw new NullPointerException("Null destinationAddressPrefix");
}
this.destinationAddressPrefix = destinationAddressPrefix;
return this;
}
@Override
public NetworkSecurityRuleProperties.Builder access(NetworkSecurityRuleProperties.Access access) {
if (access == null) {
throw new NullPointerException("Null access");
}
this.access = access;
return this;
}
@Override
public NetworkSecurityRuleProperties.Builder priority(@Nullable Integer priority) {
this.priority = priority;
return this;
}
@Override
public NetworkSecurityRuleProperties.Builder direction(NetworkSecurityRuleProperties.Direction direction) {
if (direction == null) {
throw new NullPointerException("Null direction");
}
this.direction = direction;
return this;
}
@Override
public NetworkSecurityRuleProperties.Builder provisioningState(@Nullable String provisioningState) {
this.provisioningState = provisioningState;
return this;
}
@Override
public NetworkSecurityRuleProperties build() {
String missing = "";
if (this.protocol == null) {
missing += " protocol";
}
if (this.sourceAddressPrefix == null) {
missing += " sourceAddressPrefix";
}
if (this.destinationAddressPrefix == null) {
missing += " destinationAddressPrefix";
}
if (this.access == null) {
missing += " access";
}
if (this.direction == null) {
missing += " direction";
}
if (!missing.isEmpty()) {
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_NetworkSecurityRuleProperties(
this.description,
this.protocol,
this.sourcePortRange,
this.destinationPortRange,
this.sourceAddressPrefix,
this.destinationAddressPrefix,
this.access,
this.priority,
this.direction,
this.provisioningState);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy