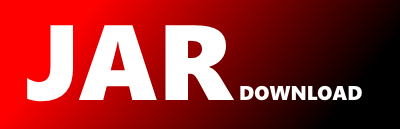
org.jclouds.azurecompute.arm.domain.AutoValue_ProbeProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jclouds-shaded Show documentation
Show all versions of jclouds-shaded Show documentation
Provides a shaded jclouds with relocated guava and guice
The newest version!
package org.jclouds.azurecompute.arm.domain;
import javax.annotation.Generated;
import org.jclouds.javax.annotation.Nullable;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_ProbeProperties extends ProbeProperties {
private final ProbeProperties.Protocol protocol;
private final int port;
private final String requestPath;
private final int intervalInSeconds;
private final int numberOfProbes;
private final String provisioningState;
private AutoValue_ProbeProperties(
@Nullable ProbeProperties.Protocol protocol,
int port,
@Nullable String requestPath,
int intervalInSeconds,
int numberOfProbes,
@Nullable String provisioningState) {
this.protocol = protocol;
this.port = port;
this.requestPath = requestPath;
this.intervalInSeconds = intervalInSeconds;
this.numberOfProbes = numberOfProbes;
this.provisioningState = provisioningState;
}
@Nullable
@Override
public ProbeProperties.Protocol protocol() {
return protocol;
}
@Override
public int port() {
return port;
}
@Nullable
@Override
public String requestPath() {
return requestPath;
}
@Override
public int intervalInSeconds() {
return intervalInSeconds;
}
@Override
public int numberOfProbes() {
return numberOfProbes;
}
@Nullable
@Override
public String provisioningState() {
return provisioningState;
}
@Override
public String toString() {
return "ProbeProperties{"
+ "protocol=" + protocol + ", "
+ "port=" + port + ", "
+ "requestPath=" + requestPath + ", "
+ "intervalInSeconds=" + intervalInSeconds + ", "
+ "numberOfProbes=" + numberOfProbes + ", "
+ "provisioningState=" + provisioningState
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof ProbeProperties) {
ProbeProperties that = (ProbeProperties) o;
return ((this.protocol == null) ? (that.protocol() == null) : this.protocol.equals(that.protocol()))
&& (this.port == that.port())
&& ((this.requestPath == null) ? (that.requestPath() == null) : this.requestPath.equals(that.requestPath()))
&& (this.intervalInSeconds == that.intervalInSeconds())
&& (this.numberOfProbes == that.numberOfProbes())
&& ((this.provisioningState == null) ? (that.provisioningState() == null) : this.provisioningState.equals(that.provisioningState()));
}
return false;
}
@Override
public int hashCode() {
int h = 1;
h *= 1000003;
h ^= (protocol == null) ? 0 : this.protocol.hashCode();
h *= 1000003;
h ^= this.port;
h *= 1000003;
h ^= (requestPath == null) ? 0 : this.requestPath.hashCode();
h *= 1000003;
h ^= this.intervalInSeconds;
h *= 1000003;
h ^= this.numberOfProbes;
h *= 1000003;
h ^= (provisioningState == null) ? 0 : this.provisioningState.hashCode();
return h;
}
@Override
public ProbeProperties.Builder toBuilder() {
return new Builder(this);
}
static final class Builder extends ProbeProperties.Builder {
private ProbeProperties.Protocol protocol;
private Integer port;
private String requestPath;
private Integer intervalInSeconds;
private Integer numberOfProbes;
private String provisioningState;
Builder() {
}
private Builder(ProbeProperties source) {
this.protocol = source.protocol();
this.port = source.port();
this.requestPath = source.requestPath();
this.intervalInSeconds = source.intervalInSeconds();
this.numberOfProbes = source.numberOfProbes();
this.provisioningState = source.provisioningState();
}
@Override
public ProbeProperties.Builder protocol(@Nullable ProbeProperties.Protocol protocol) {
this.protocol = protocol;
return this;
}
@Override
public ProbeProperties.Builder port(int port) {
this.port = port;
return this;
}
@Override
public ProbeProperties.Builder requestPath(@Nullable String requestPath) {
this.requestPath = requestPath;
return this;
}
@Override
public ProbeProperties.Builder intervalInSeconds(int intervalInSeconds) {
this.intervalInSeconds = intervalInSeconds;
return this;
}
@Override
public ProbeProperties.Builder numberOfProbes(int numberOfProbes) {
this.numberOfProbes = numberOfProbes;
return this;
}
@Override
public ProbeProperties.Builder provisioningState(@Nullable String provisioningState) {
this.provisioningState = provisioningState;
return this;
}
@Override
public ProbeProperties build() {
String missing = "";
if (this.port == null) {
missing += " port";
}
if (this.intervalInSeconds == null) {
missing += " intervalInSeconds";
}
if (this.numberOfProbes == null) {
missing += " numberOfProbes";
}
if (!missing.isEmpty()) {
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_ProbeProperties(
this.protocol,
this.port,
this.requestPath,
this.intervalInSeconds,
this.numberOfProbes,
this.provisioningState);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy