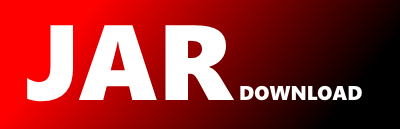
org.jclouds.azurecompute.arm.domain.AutoValue_Resource Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jclouds-shaded Show documentation
Show all versions of jclouds-shaded Show documentation
Provides a shaded jclouds with relocated guava and guice
The newest version!
package org.jclouds.azurecompute.arm.domain;
import java.util.Map;
import javax.annotation.Generated;
import org.jclouds.javax.annotation.Nullable;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_Resource extends Resource {
private final String id;
private final String name;
private final String type;
private final String location;
private final Map tags;
private final Resource.Identity identity;
private final SKU sku;
private final String managedBy;
private final String kind;
private final Plan plan;
private final Resource.ResourceProperties properties;
AutoValue_Resource(
String id,
String name,
String type,
String location,
@Nullable Map tags,
@Nullable Resource.Identity identity,
@Nullable SKU sku,
@Nullable String managedBy,
@Nullable String kind,
@Nullable Plan plan,
@Nullable Resource.ResourceProperties properties) {
if (id == null) {
throw new NullPointerException("Null id");
}
this.id = id;
if (name == null) {
throw new NullPointerException("Null name");
}
this.name = name;
if (type == null) {
throw new NullPointerException("Null type");
}
this.type = type;
if (location == null) {
throw new NullPointerException("Null location");
}
this.location = location;
this.tags = tags;
this.identity = identity;
this.sku = sku;
this.managedBy = managedBy;
this.kind = kind;
this.plan = plan;
this.properties = properties;
}
@Override
public String id() {
return id;
}
@Override
public String name() {
return name;
}
@Override
public String type() {
return type;
}
@Override
public String location() {
return location;
}
@Nullable
@Override
public Map tags() {
return tags;
}
@Nullable
@Override
public Resource.Identity identity() {
return identity;
}
@Nullable
@Override
public SKU sku() {
return sku;
}
@Nullable
@Override
public String managedBy() {
return managedBy;
}
@Nullable
@Override
public String kind() {
return kind;
}
@Nullable
@Override
public Plan plan() {
return plan;
}
@Nullable
@Override
public Resource.ResourceProperties properties() {
return properties;
}
@Override
public String toString() {
return "Resource{"
+ "id=" + id + ", "
+ "name=" + name + ", "
+ "type=" + type + ", "
+ "location=" + location + ", "
+ "tags=" + tags + ", "
+ "identity=" + identity + ", "
+ "sku=" + sku + ", "
+ "managedBy=" + managedBy + ", "
+ "kind=" + kind + ", "
+ "plan=" + plan + ", "
+ "properties=" + properties
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof Resource) {
Resource that = (Resource) o;
return (this.id.equals(that.id()))
&& (this.name.equals(that.name()))
&& (this.type.equals(that.type()))
&& (this.location.equals(that.location()))
&& ((this.tags == null) ? (that.tags() == null) : this.tags.equals(that.tags()))
&& ((this.identity == null) ? (that.identity() == null) : this.identity.equals(that.identity()))
&& ((this.sku == null) ? (that.sku() == null) : this.sku.equals(that.sku()))
&& ((this.managedBy == null) ? (that.managedBy() == null) : this.managedBy.equals(that.managedBy()))
&& ((this.kind == null) ? (that.kind() == null) : this.kind.equals(that.kind()))
&& ((this.plan == null) ? (that.plan() == null) : this.plan.equals(that.plan()))
&& ((this.properties == null) ? (that.properties() == null) : this.properties.equals(that.properties()));
}
return false;
}
@Override
public int hashCode() {
int h = 1;
h *= 1000003;
h ^= this.id.hashCode();
h *= 1000003;
h ^= this.name.hashCode();
h *= 1000003;
h ^= this.type.hashCode();
h *= 1000003;
h ^= this.location.hashCode();
h *= 1000003;
h ^= (tags == null) ? 0 : this.tags.hashCode();
h *= 1000003;
h ^= (identity == null) ? 0 : this.identity.hashCode();
h *= 1000003;
h ^= (sku == null) ? 0 : this.sku.hashCode();
h *= 1000003;
h ^= (managedBy == null) ? 0 : this.managedBy.hashCode();
h *= 1000003;
h ^= (kind == null) ? 0 : this.kind.hashCode();
h *= 1000003;
h ^= (plan == null) ? 0 : this.plan.hashCode();
h *= 1000003;
h ^= (properties == null) ? 0 : this.properties.hashCode();
return h;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy