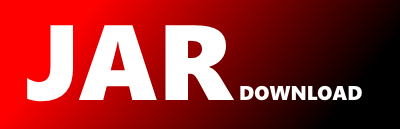
org.jclouds.azurecompute.arm.domain.AutoValue_ResourceProviderMetaData Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jclouds-shaded Show documentation
Show all versions of jclouds-shaded Show documentation
Provides a shaded jclouds with relocated guava and guice
The newest version!
package org.jclouds.azurecompute.arm.domain;
import java.util.List;
import javax.annotation.Generated;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_ResourceProviderMetaData extends ResourceProviderMetaData {
private final String resourceType;
private final List locations;
private final List apiVersions;
private AutoValue_ResourceProviderMetaData(
String resourceType,
List locations,
List apiVersions) {
this.resourceType = resourceType;
this.locations = locations;
this.apiVersions = apiVersions;
}
@Override
public String resourceType() {
return resourceType;
}
@Override
public List locations() {
return locations;
}
@Override
public List apiVersions() {
return apiVersions;
}
@Override
public String toString() {
return "ResourceProviderMetaData{"
+ "resourceType=" + resourceType + ", "
+ "locations=" + locations + ", "
+ "apiVersions=" + apiVersions
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof ResourceProviderMetaData) {
ResourceProviderMetaData that = (ResourceProviderMetaData) o;
return (this.resourceType.equals(that.resourceType()))
&& (this.locations.equals(that.locations()))
&& (this.apiVersions.equals(that.apiVersions()));
}
return false;
}
@Override
public int hashCode() {
int h = 1;
h *= 1000003;
h ^= this.resourceType.hashCode();
h *= 1000003;
h ^= this.locations.hashCode();
h *= 1000003;
h ^= this.apiVersions.hashCode();
return h;
}
@Override
public ResourceProviderMetaData.Builder toBuilder() {
return new Builder(this);
}
static final class Builder extends ResourceProviderMetaData.Builder {
private String resourceType;
private List locations;
private List apiVersions;
Builder() {
}
private Builder(ResourceProviderMetaData source) {
this.resourceType = source.resourceType();
this.locations = source.locations();
this.apiVersions = source.apiVersions();
}
@Override
public ResourceProviderMetaData.Builder resourceType(String resourceType) {
if (resourceType == null) {
throw new NullPointerException("Null resourceType");
}
this.resourceType = resourceType;
return this;
}
@Override
public ResourceProviderMetaData.Builder locations(List locations) {
if (locations == null) {
throw new NullPointerException("Null locations");
}
this.locations = locations;
return this;
}
@Override
List locations() {
if (locations == null) {
throw new IllegalStateException("Property \"locations\" has not been set");
}
return locations;
}
@Override
public ResourceProviderMetaData.Builder apiVersions(List apiVersions) {
if (apiVersions == null) {
throw new NullPointerException("Null apiVersions");
}
this.apiVersions = apiVersions;
return this;
}
@Override
List apiVersions() {
if (apiVersions == null) {
throw new IllegalStateException("Property \"apiVersions\" has not been set");
}
return apiVersions;
}
@Override
ResourceProviderMetaData autoBuild() {
String missing = "";
if (this.resourceType == null) {
missing += " resourceType";
}
if (this.locations == null) {
missing += " locations";
}
if (this.apiVersions == null) {
missing += " apiVersions";
}
if (!missing.isEmpty()) {
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_ResourceProviderMetaData(
this.resourceType,
this.locations,
this.apiVersions);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy