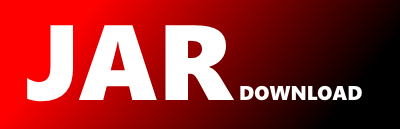
org.jclouds.azurecompute.arm.domain.AutoValue_Subnet_SubnetProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jclouds-shaded Show documentation
Show all versions of jclouds-shaded Show documentation
Provides a shaded jclouds with relocated guava and guice
The newest version!
package org.jclouds.azurecompute.arm.domain;
import java.util.List;
import javax.annotation.Generated;
import org.jclouds.javax.annotation.Nullable;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_Subnet_SubnetProperties extends Subnet.SubnetProperties {
private final String provisioningState;
private final String addressPrefix;
private final List ipConfigurations;
private AutoValue_Subnet_SubnetProperties(
@Nullable String provisioningState,
@Nullable String addressPrefix,
@Nullable List ipConfigurations) {
this.provisioningState = provisioningState;
this.addressPrefix = addressPrefix;
this.ipConfigurations = ipConfigurations;
}
@Nullable
@Override
public String provisioningState() {
return provisioningState;
}
@Nullable
@Override
public String addressPrefix() {
return addressPrefix;
}
@Nullable
@Override
public List ipConfigurations() {
return ipConfigurations;
}
@Override
public String toString() {
return "SubnetProperties{"
+ "provisioningState=" + provisioningState + ", "
+ "addressPrefix=" + addressPrefix + ", "
+ "ipConfigurations=" + ipConfigurations
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof Subnet.SubnetProperties) {
Subnet.SubnetProperties that = (Subnet.SubnetProperties) o;
return ((this.provisioningState == null) ? (that.provisioningState() == null) : this.provisioningState.equals(that.provisioningState()))
&& ((this.addressPrefix == null) ? (that.addressPrefix() == null) : this.addressPrefix.equals(that.addressPrefix()))
&& ((this.ipConfigurations == null) ? (that.ipConfigurations() == null) : this.ipConfigurations.equals(that.ipConfigurations()));
}
return false;
}
@Override
public int hashCode() {
int h = 1;
h *= 1000003;
h ^= (provisioningState == null) ? 0 : this.provisioningState.hashCode();
h *= 1000003;
h ^= (addressPrefix == null) ? 0 : this.addressPrefix.hashCode();
h *= 1000003;
h ^= (ipConfigurations == null) ? 0 : this.ipConfigurations.hashCode();
return h;
}
@Override
public Subnet.SubnetProperties.Builder toBuilder() {
return new Builder(this);
}
static final class Builder extends Subnet.SubnetProperties.Builder {
private String provisioningState;
private String addressPrefix;
private List ipConfigurations;
Builder() {
}
private Builder(Subnet.SubnetProperties source) {
this.provisioningState = source.provisioningState();
this.addressPrefix = source.addressPrefix();
this.ipConfigurations = source.ipConfigurations();
}
@Override
public Subnet.SubnetProperties.Builder provisioningState(@Nullable String provisioningState) {
this.provisioningState = provisioningState;
return this;
}
@Override
public Subnet.SubnetProperties.Builder addressPrefix(@Nullable String addressPrefix) {
this.addressPrefix = addressPrefix;
return this;
}
@Override
public Subnet.SubnetProperties.Builder ipConfigurations(@Nullable List ipConfigurations) {
this.ipConfigurations = ipConfigurations;
return this;
}
@Override
@Nullable List ipConfigurations() {
return ipConfigurations;
}
@Override
Subnet.SubnetProperties autoBuild() {
return new AutoValue_Subnet_SubnetProperties(
this.provisioningState,
this.addressPrefix,
this.ipConfigurations);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy