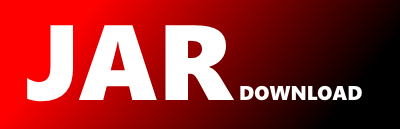
org.jclouds.azurecompute.arm.domain.AutoValue_VMHardware Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jclouds-shaded Show documentation
Show all versions of jclouds-shaded Show documentation
Provides a shaded jclouds with relocated guava and guice
The newest version!
package org.jclouds.azurecompute.arm.domain;
import javax.annotation.Generated;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_VMHardware extends VMHardware {
private final String name;
private final Integer numberOfCores;
private final Integer osDiskSizeInMB;
private final Integer resourceDiskSizeInMB;
private final Integer memoryInMB;
private final Integer maxDataDiskCount;
private final String location;
AutoValue_VMHardware(
String name,
Integer numberOfCores,
Integer osDiskSizeInMB,
Integer resourceDiskSizeInMB,
Integer memoryInMB,
Integer maxDataDiskCount,
String location) {
if (name == null) {
throw new NullPointerException("Null name");
}
this.name = name;
if (numberOfCores == null) {
throw new NullPointerException("Null numberOfCores");
}
this.numberOfCores = numberOfCores;
if (osDiskSizeInMB == null) {
throw new NullPointerException("Null osDiskSizeInMB");
}
this.osDiskSizeInMB = osDiskSizeInMB;
if (resourceDiskSizeInMB == null) {
throw new NullPointerException("Null resourceDiskSizeInMB");
}
this.resourceDiskSizeInMB = resourceDiskSizeInMB;
if (memoryInMB == null) {
throw new NullPointerException("Null memoryInMB");
}
this.memoryInMB = memoryInMB;
if (maxDataDiskCount == null) {
throw new NullPointerException("Null maxDataDiskCount");
}
this.maxDataDiskCount = maxDataDiskCount;
if (location == null) {
throw new NullPointerException("Null location");
}
this.location = location;
}
@Override
public String name() {
return name;
}
@Override
public Integer numberOfCores() {
return numberOfCores;
}
@Override
public Integer osDiskSizeInMB() {
return osDiskSizeInMB;
}
@Override
public Integer resourceDiskSizeInMB() {
return resourceDiskSizeInMB;
}
@Override
public Integer memoryInMB() {
return memoryInMB;
}
@Override
public Integer maxDataDiskCount() {
return maxDataDiskCount;
}
@Override
public String location() {
return location;
}
@Override
public String toString() {
return "VMHardware{"
+ "name=" + name + ", "
+ "numberOfCores=" + numberOfCores + ", "
+ "osDiskSizeInMB=" + osDiskSizeInMB + ", "
+ "resourceDiskSizeInMB=" + resourceDiskSizeInMB + ", "
+ "memoryInMB=" + memoryInMB + ", "
+ "maxDataDiskCount=" + maxDataDiskCount + ", "
+ "location=" + location
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof VMHardware) {
VMHardware that = (VMHardware) o;
return (this.name.equals(that.name()))
&& (this.numberOfCores.equals(that.numberOfCores()))
&& (this.osDiskSizeInMB.equals(that.osDiskSizeInMB()))
&& (this.resourceDiskSizeInMB.equals(that.resourceDiskSizeInMB()))
&& (this.memoryInMB.equals(that.memoryInMB()))
&& (this.maxDataDiskCount.equals(that.maxDataDiskCount()))
&& (this.location.equals(that.location()));
}
return false;
}
@Override
public int hashCode() {
int h = 1;
h *= 1000003;
h ^= this.name.hashCode();
h *= 1000003;
h ^= this.numberOfCores.hashCode();
h *= 1000003;
h ^= this.osDiskSizeInMB.hashCode();
h *= 1000003;
h ^= this.resourceDiskSizeInMB.hashCode();
h *= 1000003;
h ^= this.memoryInMB.hashCode();
h *= 1000003;
h ^= this.maxDataDiskCount.hashCode();
h *= 1000003;
h ^= this.location.hashCode();
return h;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy